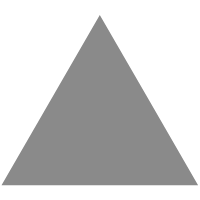
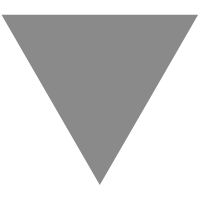
Eloquent TypeScript Tips
source link: https://www.tuicool.com/articles/hit/3qy6beE
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Eloquent TypeScript Tips
A few tricks on how to write TypeScript code to minimize time spent refactoring and reviewing code.

Use Types
You’re using TypeScript because you want to use types. This should be self-explanatory, but I have seen a lot of code example on the internet where people are writing TypeScript more like it is just regular JavaScript. By default typing is optional, not mandatory. To make sure that typing does not go unenforced, add the option noImplicitAny
to the tsConfig.json
file compiler options and set it to true
.
Also avoid using the “any” type if you can. Sometimes it’s a necessary evil, but don’t use it carelessly. Types do add more to your code, but they also make it way more readable.
Indentation and Curly Braces
As a professional developer, I’ve been baffled by the amount of sloppy indentation there is in the real world. Make sure you code with consistent spacing and always always always use spaces, never tabs, so that indentation doesn’t get screwed up when copy-n-pasting your code.
There are different conventions across different languages for placing curly braces at the end of statements. Some like putting them on the next line because it spaces the code out more and makes it easier to read, while others like keeping them on the same line because it makes the code feel more terse and is a bit overkill when you have short statements such as an if()
statement or for()
loop that only execute a single line of code.
I believe next lines are useful for methods and classes because they make the names/signatures of them stand out more when skimming through code. But I do think they are a bit overkill for short boolean and loop statements. Here’s a crazy idea, and this is how I code, use the next line for classes and methods and the same line for the content in methods.
So instead of this:
Do this:
Semicolons
I know I might get some flack for this one. But come on… just don’t use semicolons. It’s only more noise on the screen. If you are worried about about no semicolons causing problems when trying to minify your code, don’t fret — the transpiler has got you covered. It will automatically add them for you. The “Dog” class in the previous snippet will transpile to this with all default settings in tsconfig.json
:
Comments and Underscores
Put comments at the top of all of your TypeScript files briefly mentioning what the class if for, and who and when created it. This helps other developers know who made the module/class if they have questions about it. Only put comments for methods if there is no easy way to explain what the method does via the the method signature. Good, clean, well written code should only need minimal comments.
To help other developers know where methods in the code are coming from, use a beginning underscore for private
methods and variables and an ending underscore for protected
methods and variables. If you’re an Angular developer, I think this is especially handy for knowing what variables are accessed in the template vs which ones are only used in the component’s TypeScript file. Also, use ALL_CAPS for readonly
and const
.
Separation of Concerns
I’ve seen this all too often throughout my career where developers create giant methods, sometimes over a hundred lines long: this makes it hard to understand.
Separation of Concerns should apply to all programming languages. Every chunk of logic in your code should only do one thing. If a public method needs to do a lot of stuff, break it up in smaller private methods and have them return all their values to a single public one. Unfortunately, knowing what counts as a “chunk of logic” is not an exact science. A good rule of thumb that I use is if a method gets to be longer than 15–20 lines (including the signature), see if you can break it up into smaller ones. Also make sure that a group of private methods being used together by a single public one and aren’t being shared are separated with comments.
Always use Async/Await and Try/Catch blocks
The biggest criticism of JavaScript is callback hell. So as a piece of advice, I’d say avoid using callbacks whenever possible. Some people don’t like using try/catch blocks because they make code more bloated. Well if you’re a Java/C# developer I can understand this when there are a million different types of errors to throw around.
In TypeScript though, you just need to print out the single error object which is being thrown. The catch for using async/await is that whatever I/O you’re working with must return a promise. If you’re working with some third party library which does not return a promise, create a new method which wraps a Promise object around it and return the promise.
Hope this helped! Happy TypeScripting :)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK