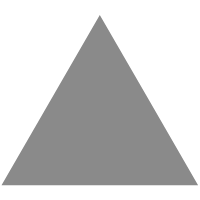
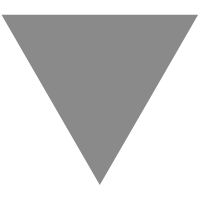
Working With Stream APIs in Java 1.8
source link: https://www.tuicool.com/articles/hit/QfYvuyu
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
The Stream concept was introduced in Java 1.8 and remains present in the java.util.stream package. It is used to process the object from the collection or any group of objects or data source. We can easily understand the Java stream concept by its name. For example, in a stream, water flows from one water source to a destination. We can perform operations like filtering, collecting, etc. via a stream to get useful water flow. It is the same when working with Java streams. We think that an object flows from one object source to its destination through Stream pipelines. Keep in mind: a stream pipeline is composed of a stream source, zero or more intermediate operations, and a terminal operation. Let's take a closer look!
We should be familiar with a few basic concepts before going any further. Remember one thing: Streams don’t actually store elements; they are computed on demand.
1. C.stream()
— We are going to get a stream object where C is the collection object.
2. filter()
— This is used to perform filtering based on a predicate. The predicate is nothing but a boolean condition. Basically, it is a functional interface and can be replaced by a lambda expression.
3. collect()
— This is a terminal operation used to collect filtered or mapped data. For example, check out the following:
ArrayList<Integer> arrayList = new ArrayList<Integer>(); arrayList.add(0); arrayList.add(10); arrayList.add(20); arrayList.add(5); arrayList.add(15); arrayList.add(25); System.out.println(arrayList);
The output will be [0,10,20,5,15,25]. Now, we want to get a new ArrayList
of integers, which contains only even integers from the existing ArrayList
object. Here, we will demonstrate how we process a collection object without the stream concept.
List<Integer> list = new ArrayList<Integer>(); for(Integer i:arrayList){ if(i%2 == 0) list.add(i); } System.out.println(arrayList);
The output will be [0,10,20].
With the stream concept, it will look something like the following:
List<Integer> list = arrayList.stream().filter(i->i%2==0).collect(Collectors.toList()); System.out.println(arrayList);
For this code, the output will be [0,10,20]. In a collection, we will perform the bulk operation, which is highly recommended for using streams.
Let's take a look at some other useful methods for implementing Streams.
4. map()
— For every object, if we want to perform an action and want a result object, then we use the Map
method. If we want to increase all the ArrayList
data by five, then we can use this function:
List<Integer> l = arrayList.stream().map(i-> i+5).collect(Collectors.toList());
It always takes the functional interface (an interface that contains only one interface), so we can replace it with the lambda expression.
5. count()
— We can use this to count how many objects are there in stream ( not in data source).
long noOfEvenInteger = arrayList.stream().filter(i-> i%2!=0).count();
6. sorted()
— We use this method to perform sorting in ascending order.
List<Integer> l = arrayList.stream().sorted().collect(Collectors.toList()); return an ascending order list.
For customization, we go to the comparator concept. The comparator is also on the functional interface, so we can also replace it with a lambda expression.
The Comparator contains the compare()
method, and we can replace it with the lambda expression compare (obj1, obj2)- return -ve if obj1 has to come before obj2, return +ve if obj1 has to come after obj2, and return 0 if both are equal.
We perform sorting in descending order here and compare method (i1, i2)-> (i1<i2)?1:(i1 > i2)?-1:0. We compare two objects: i1 and i2. If i1 is smaller than i2, it means i1 has to come before i2. So, it will return +ve, or else, it will check to see if i1 is bigger or not. If yes, it means i1 has to come after i2. Then, it will return -ve, and if both are equal, then it will return 0.
List<Integer> list = arrayList.stream().sorted((i1,i2)->(i1, i2)-> (i1<i2)?1:(i1 > i2)?-1:0).collect(Collectors.toList());
7> forEach() - for every element if we want to perform some functionality.
arrayList.stream().forEach(System.out::println);
it will print all object.We can call our own method also.
Consumer<Integer> fun = i->{System.out.println("square of"+i+"is = "+(i*i))}; arrayList.stream().forEach(fun);
8. toArray()
— We use this to convert a stream of an object into an array.
Integer[] arr =arrayList.stream().toArray(Integer::new);
9. Stream.of(arr)
— We use this to get a stream from an array or another group of data:
Stream s = stream.of(10,1,2,12,34); s.forEach(System.out::println);
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK