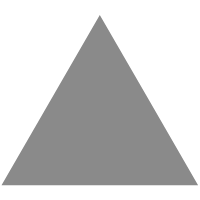
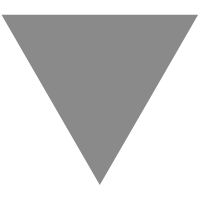
GitHub - posva/vue-promised: ? Promises as components
source link: https://github.com/posva/vue-promised
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
vue-promised

Transform your Promises into components !
Installation
npm install vue-promised
# or
yarn add vue-promised
Migrating from v0.2.x
Migrating to v1 should be doable in a small amount of time as the only breaking changes are some slots name and the way Promised
component is imported.
Check releases notes to see the list of breaking changes
Usage
Import the component to use it
import { Promised } from 'vue-promised' Vue.component('Promised', Promised)
promise
should be a Promise but can also be null
. data
will contain the result of the promise. You can of course name it the way you want:
Using pending
, default and `error slots
<Promised :promise="promise"> <!-- Use the "pending" slot for loading content --> <h1 slot="pending">Loading</h1> <!-- The default scoped slots will be used as the result --> <h1 slot-scope="data">Success!</h1> <!-- The "rejected" scoped slot will be used if there is an error --> <h1 slot="rejected" slot-scope="error">Error: {{ error.message }}</h1> </Promised>
Using one single combined
slot
You can also provide a single combined
slot that will receive a context with all relevant information
<Promised :promise="promise"> <pre slot="combined" slot-scope="{ isPending, isDelayOver, data, error }"> pending: {{ isPending }} is delay over: {{ isDelayOver }} data: {{ data }} error: {{ error && error.message }} </pre> </Promised>
This allows to create more advanced async templates like this one featuring a Search component that must be displayed while the searchResults
are being fetched:
<Promised :promise="searchResults" :pending-delay="200"> <div slot="combined" slot-scope="{ isPending, isDelayOver, data, error }"> <!-- data contains previous data or null when starting --> <Search :disabled-pagination="isPending || error" :items="data || []"> <!-- The Search handles filtering logic with pagination --> <template slot-scope="{ results, query }"> <ProfileCard v-for="user in results" :user="user"/> </template> <!-- If there is an error, data is null, therefore there are no results and we can display the error --> <MySpinner v-if="isPending && isDelayOver" slot="loading"/> <template slot="noResults"> <p v-if="error" class="error">Error: {{ error.message }}</p> <p v-else class="info">No results for "{{ query }}"</p> </template> </Search> </div> </Promised>
context
object
isPending
: istrue
while the promise is in a pending status. Becomes true once the promise is resolved or rejected. It is resetted tofalse
whenpromise
prop changes.isDelayOver
: istrue
once thependingDelay
is over or ifpendingDelay
is 0. Becomesfalse
after the specified delay (200 by default). It is resetted whenpromise
prop changes.data
: contains last resolved value frompromise
. This means it will contain the previous succesfully (non cancelled) result.error
: contais last rejection ornull
if the promise was fullfiled.
API Reference
Promised
component
Promised
will watch its prop promise
and change its state accordingly.
props
Name Description Typepromise
Promise to be resolved
Promise
tag
Wrapper tag used if multiple elements are passed to a slot. Defaults to span
String
pendingDelay
Delay in ms to wait before displaying the pending slot. Defaults to 200
Number
slots
Name Description Scopepending
Content to display while the promise is pending and before pendingDelay is over
—
default
Content to display once the promise has been successfully resolved
data
: resolved value
rejected
Content to display if the promise is rejected
error
: rejection reason
combined
Combines all slots to provide a granular control over what should be displayed
context
See details
License
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK