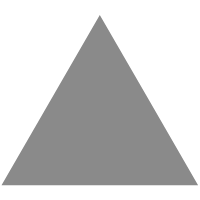
40
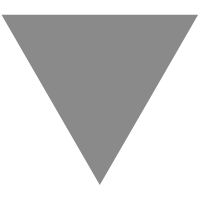
Go中的Array和Slice
source link: https://studygolang.com/articles/15658?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Go中的Array和Slice
翻译来于: https://blog.golang.org/slices
操作
extend
func Extend(slice []int, element int) []int { n := len(slice) slice = slice[0 : n+1] slice[n] = element return slice }
在下面的使用会出现错误:
func main() { var iBuffer [10]int slice := iBuffer[0:0] for i := 0; i < 20; i++ { slice = Extend(slice, i) fmt.Println(slice) } } 底层的buffer最大长度为10,所有在extend到10的时候会出现out of range
扩展版本的extend
func Extend(slice []int, element int) []int { n := len(slice) if n == cap(slice) { // Slice is full; must grow. // We double its size and add 1, so if the size is zero we still grow. newSlice := make([]int, len(slice), 2*len(slice)+1) copy(newSlice, slice) slice = newSlice } slice = slice[0 : n+1] slice[n] = element return slice }
首先要保证len和cap的带下关系。当len不等于cap的时候extends slice
的大小。
copy
copy(newSlice, slice) copy(slice[index+1:], slice[index:])
一定要保证容量的问题
array to slice
var array = [n]int slice = array[:] slice = array[begin:end]
slice to array
在调用append方法的时候需要传入的是个数不定的element func Append(slice []int, elements ...int) []int{ } slice1 := []int{0, 1, 2, 3, 4} slice2 := []int{55, 66, 77} slice1 = Append(slice1, slice2...) // The '...' is essential!
nil slice 和 empty slice
参考: https://blog.golang.org/go-slices-usage-and-internals
var slice []int 这里的slice==nil 但是 slice = make([]int,0,0) slice不等于nil
cap len 操作
nil slice的cap和len的大小都为0 并且可以使用append操作
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK