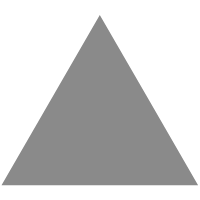
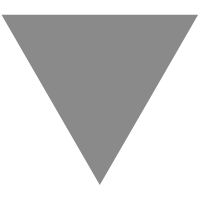
GitHub - magit/libegit2: Emacs bindings for libgit2
source link: https://github.com/magit/libegit2
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
libgit2 bindings for Emacs
This is an experimental module for libgit2 bindings to Emacs, intended to boost the performance of magit.
Other work in this direction:
- ksjogo/emacs-libgit2 in C, has been dormant for more than a year.
- ubolonton/magit-libgit2 in Rust.
This module is written in C, and aims to be a thin wrapper around libgit2. That means that all functions in the libgit2 reference should translate more-or-less directly to Emacs, in the following sense:
- Function names are the same, except with underscores replaced by hyphens. The prefix is changed
from
git-
tolibgit-
. - Predicate functions are given a
-p
suffix, and words like "is" are removed, e.g.git_repository_is_bare
becomeslibgit-repository-bare-p
. - Output parameters become return values.
- Error codes become error signals (type
giterr
). - Return types map to their natural Emacs counterparts, or opaque user pointers when not applicable
(e.g. for
git-???
structures). Exceptions:git-oid
andgit-buf
types are converted to Emacs strings. - Boolean parameters or pointers towards the end of argument lists whose natural default value is false or NULL will be made optional.
Quality-of-life convenience functionality is better implemented in Emacs Lisp than in C.
Building
There is a loader file written in Emacs Lisp that will build the module for you, but the
git submodule
steps need to be run manually.
git submodule init
git submodule update
mkdir build
cd build
cmake ..
make
If you're on OSX and using Macports, you may need to set CMAKE_PREFIX_PATH
to avoid linking
against the wrong libiconv. For example,
cmake -DCMAKE_PREFIX_PATH=/opt/local ..
Testing
Ensure that you have Cask installed.
cask install
cd build
make test
Using
Ensure that libgit.el
is somewhere in your load path. Then
(require 'libgit)
If the dynamic module was not already built, you should be asked to do it manually.
If you use Borg, the following .gitmodules
entry should
work.
[submodule "libegit2"]
path = lib/libegit2
url = [email protected]:TheBB/libegit2.git
build-step = git submodule init
build-step = git submodule update
build-step = mkdir -p build
build-step = cd build && cmake ..
build-step = cd build && make
Contributing
Adding a function
- Find the section that the function belongs to (i.e.
git_SECTION_xyz
). - Create, if necessary,
src/egit-SECTION.h
andsrc/egit-SECTION.c
. - In
src/egit-SECTION.h
, declare the function withEGIT_DEFUN
. See existing headers for examples. - In
src/egit-SECTION.c
, document the function withEGIT_DOC
. See existing files for examples. - In
src/egit-SECTION.c
, implement the function. See existing files for examples.- Always check argument types in the beginning. Use
EGIT_ASSERT
for this. These macros may return. - Then, extract the data needed from
emacs_value
. This may involve allocating buffers for strings. - Call the
libgit2
backend function. - Free any memory you might need to free that was allocated in step 2.
- Check the error code if applicable with
EGIT_CHECK_ERROR
. This macro may return. - Create return value and return.
- Always check argument types in the beginning. Use
- In
src/egit.c
, create aDEFUN
call inegit_init
. You may need to include a new header.
Adding a type
Sometimes a struct of type git_???
may need to be returned to Emacs as an opaque user pointer.
To do this, we use a wrapper structure with a type information tag.
Usually, objects that belong to a repository need to keep the repository alive until after they are freed. To do this, we use a hash table with reference counting semantics for repositories to ensure that none of them are freed out of turn.
- In
src/egit.h
add an entry to theegit_type
enum for the new type. - In
src/egit.h
ass a newEGIT_ASSERT
macro for the new type. - In
src/egit.c
add a new entry to theegit_finalize
switch statement to free a structure. If the new structure needs to keep a repository alive (usually the "owner" in libgit2 terms), also callegit_decref_repository
on these (see existing code for examples). - In
src/egit.c
add a new entry to theegit_wrap
switch statement to increase the reference counts of the repository if it must be kept alive. - In
src/egit.c
add a new entry to theegit_typeof
switch statement. - In
src/egit.c
add a newegit_TYPE_p
predicate function. - In
src/egit.c
create aDEFUN
call inegit_init
for the predicate function. - In
interface.h
add two new symbols,TYPE-p
andTYPE
. - In
interface.c
initialize those symbols in theem_init
function.
Function list
This is a complete list of functions in libgit2. It therefore serves more or less as an upper bound on the amount of work needed.
Legend:
- ✔️ Function is implemented
- ❌ Function should probably not be implemented (reason given)
- ⁉️ Undecided
Some functions are defined in libgit2 headers in the sys
subdirectory, and are not reachable from
a standard include (i.e. #include "git2.h"
). For now, we will skip those on the assumption that
they are more specialized.
Estimates (updated periodically):
- Implemented: 54 (7.0%)
- Should not implement: 80 (10.4%)
- To do: 637 (82.6%)
- Total: 771
extra
These are functions that do not have a libgit2
equivalent.
- ✔️
git-object-p
- ✔️
git-reference-p
- ✔️
git-repository-p
- ✔️
git-typeof
- ✔️
git-reference-direct-p
- ✔️
git-reference-symbolic-p
- other type-checking predicates as we add more types
annotated
- ❌
git-annotated-commit-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-annotated-commit-from-fetchhead
- ⁉️
git-annotated-commit-from-ref
- ⁉️
git-annotated-commit-from-revspec
- ⁉️
git-annotated-commit-id
- ⁉️
git-annotated-commit-lookup
attr
- ⁉️
git-attr-add-macro
- ⁉️
git-attr-cache-flush
- ⁉️
git-attr-foreach
- ⁉️
git-attr-get
- ⁉️
git-attr-get-many
- ⁉️
git-attr-value
blame
- ⁉️
git-blame-buffer
- ⁉️
git-blame-file
- ❌
git-blame-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-blame-get-hunk-byindex
- ⁉️
git-blame-get-hunk-byline
- ⁉️
git-blame-get-hunk-count
- ⁉️
git-blame-init-options
blob
- ⁉️
git-blob-create-frombuffer
- ⁉️
git-blob-create-fromdisk
- ⁉️
git-blob-create-fromstream
- ⁉️
git-blob-create-fromstream-commit
- ⁉️
git-blob-create-fromworkdir
- ⁉️
git-blob-dup
- ⁉️
git-blob-filtered-content
- ❌
git-blob-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-blob-id
- ⁉️
git-blob-is-binary
- ⁉️
git-blob-lookup
- ⁉️
git-blob-lookup-prefix
- ⁉️
git-blob-owner
- ⁉️
git-blob-rawcontent
- ⁉️
git-blob-rawsize
branch
- ⁉️
git-branch-create
- ⁉️
git-branch-create-from-annotated
- ⁉️
git-branch-delete
- ⁉️
git-branch-is-checked-out
- ⁉️
git-branch-is-head
- ⁉️
git-branch-iterator-free
- ⁉️
git-branch-iterator-new
- ⁉️
git-branch-lookup
- ⁉️
git-branch-move
- ⁉️
git-branch-name
- ⁉️
git-branch-next
- ⁉️
git-branch-set-upstream
- ⁉️
git-branch-upstream
buf
Probably none of these functions are necessary, since we can expose buffers to Emacs as strings.
- ❌
git-buf-contains-nul
- ❌
git-buf-free
(memory management shouldn't be exposed to Emacs) - ❌
git-buf-grow
- ❌
git-buf-is-binary
- ❌
git-buf-set
checkout
- ⁉️
git-checkout-head
- ⁉️
git-checkout-index
- ⁉️
git-checkout-init-options
- ⁉️
git-checkout-tree
cherrypick
- ⁉️
git-cherrypick
- ⁉️
git-cherrypick-commit
- ⁉️
git-cherrypick-init-options
clone
- ✔️
git-clone
- ⁉️
git-clone-init-options
commit
- ⁉️
git-commit-amend
- ⁉️
git-commit-author
- ⁉️
git-commit-body
- ⁉️
git-commit-committer
- ⁉️
git-commit-create
- ⁉️
git-commit-create-buffer
- ⁉️
git-commit-create-from-callback
- ⁉️
git-commit-create-from-ids
- ⁉️
git-commit-create-v
- ⁉️
git-commit-create-with-signature
- ⁉️
git-commit-dup
- ⁉️
git-commit-extract-signature
- ❌
git-commit-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-commit-header-field
- ⁉️
git-commit-id
- ⁉️
git-commit-lookup
- ⁉️
git-commit-lookup-prefix
- ⁉️
git-commit-message
- ⁉️
git-commit-message-encoding
- ⁉️
git-commit-message-raw
- ⁉️
git-commit-nth-gen-ancestor
- ⁉️
git-commit-owner
- ⁉️
git-commit-parent
- ⁉️
git-commit-parent-id
- ⁉️
git-commit-parentcount
- ⁉️
git-commit-raw-header
- ⁉️
git-commit-summary
- ⁉️
git-commit-time
- ⁉️
git-commit-time-offset
- ⁉️
git-commit-tree
- ⁉️
git-commit-tree-id
config
- ⁉️
git-config-add-backend
- ⁉️
git-config-add-file-ondisk
- ⁉️
git-config-backend-foreach-match
- ⁉️
git-config-delete-entry
- ⁉️
git-config-delete-multivar
- ❌
git-config-entry-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-config-find-global
- ⁉️
git-config-find-programdata
- ⁉️
git-config-find-system
- ⁉️
git-config-find-xdg
- ⁉️
git-config-foreach
- ⁉️
git-config-foreach-match
- ❌
git-config-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-config-get-bool
- ⁉️
git-config-get-entry
- ⁉️
git-config-get-int32
- ⁉️
git-config-get-int64
- ⁉️
git-config-get-mapped
- ⁉️
git-config-get-multivar-foreach
- ⁉️
git-config-get-path
- ⁉️
git-config-get-string
- ⁉️
git-config-get-string-buf
- ⁉️
git-config-init-backend
- ❌
git-config-iterator-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-config-iterator-glob-new
- ⁉️
git-config-iterator-new
- ⁉️
git-config-lock
- ⁉️
git-config-lookup-map-value
- ⁉️
git-config-multivar-iterator-new
- ⁉️
git-config-new
- ⁉️
git-config-next
- ⁉️
git-config-open-default
- ⁉️
git-config-open-global
- ⁉️
git-config-open-level
- ⁉️
git-config-open-ondisk
- ⁉️
git-config-parse-bool
- ⁉️
git-config-parse-int32
- ⁉️
git-config-parse-int64
- ⁉️
git-config-parse-path
- ⁉️
git-config-set-bool
- ⁉️
git-config-set-int32
- ⁉️
git-config-set-int64
- ⁉️
git-config-set-multivar
- ⁉️
git-config-set-string
- ⁉️
git-config-snapshot
cred
- ⁉️
git-cred-default-new
- ❌
git-cred-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-cred-has-username
- ⁉️
git-cred-ssh-custom-new
- ⁉️
git-cred-ssh-interactive-new
- ⁉️
git-cred-ssh-key-from-agent
- ⁉️
git-cred-ssh-key-memory-new
- ⁉️
git-cred-ssh-key-new
- ⁉️
git-cred-username-new
- ⁉️
git-cred-userpass
- ⁉️
git-cred-userpass-plaintext-new
describe
- ⁉️
git-describe-commit
- ⁉️
git-describe-format
- ⁉️
git-describe-result-free
- ⁉️
git-describe-workdir
diff
- ⁉️
git-diff-blob-to-buffer
- ⁉️
git-diff-blobs
- ⁉️
git-diff-buffers
- ⁉️
git-diff-commit-as-email
- ⁉️
git-diff-find-init-options
- ⁉️
git-diff-find-similar
- ⁉️
git-diff-foreach
- ⁉️
git-diff-format-email
- ⁉️
git-diff-format-email-init-options
- ❌
git-diff-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-diff-from-buffer
- ⁉️
git-diff-get-delta
- ⁉️
git-diff-get-perfdata
- ⁉️
git-diff-get-stats
- ⁉️
git-diff-index-to-index
- ⁉️
git-diff-index-to-workdir
- ⁉️
git-diff-init-options
- ⁉️
git-diff-is-sorted-icase
- ⁉️
git-diff-merge
- ⁉️
git-diff-num-deltas
- ⁉️
git-diff-num-deltas-of-type
- ⁉️
git-diff-patchid
- ⁉️
git-diff-patchid-init-options
- ⁉️
git-diff-print
- ⁉️
git-diff-print-callback--to-buf
- ⁉️
git-diff-print-callback--to-file-handle
- ⁉️
git-diff-stats-deletions
- ⁉️
git-diff-stats-files-changed
- ❌
git-diff-stats-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-diff-stats-insertions
- ⁉️
git-diff-stats-to-buf
- ⁉️
git-diff-status-char
- ⁉️
git-diff-to-buf
- ⁉️
git-diff-tree-to-index
- ⁉️
git-diff-tree-to-tree
- ⁉️
git-diff-tree-to-workdir
- ⁉️
git-diff-tree-to-workdir-with-index
fetch
- ⁉️
git-fetch-init-options
filter
- ⁉️
git-filter-init
- ⁉️
git-filter-list-apply-to-blob
- ⁉️
git-filter-list-apply-to-data
- ⁉️
git-filter-list-apply-to-file
- ⁉️
git-filter-list-contains
- ❌
git-filter-list-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-filter-list-length
- ⁉️
git-filter-list-load
- ⁉️
git-filter-list-new
- ⁉️
git-filter-list-push
- ⁉️
git-filter-list-stream-blob
- ⁉️
git-filter-list-stream-data
- ⁉️
git-filter-list-stream-file
- ⁉️
git-filter-lookup
- ⁉️
git-filter-register
- ⁉️
git-filter-source-filemode
- ⁉️
git-filter-source-flags
- ⁉️
git-filter-source-id
- ⁉️
git-filter-source-mode
- ⁉️
git-filter-source-path
- ⁉️
git-filter-source-repo
- ⁉️
git-filter-unregister
giterr
Probably none of these functions will be necessary, since we expose errors to Emacs as signals.
- ❌
giterr-clear
- ❌
giterr-last
- ❌
giterr-set-oom
- ❌
giterr-set-str
graph
- ⁉️
git-graph-ahead-behind
- ⁉️
git-graph-descendant-of
hashsig
- ⁉️
git-hashsig-compare
- ⁉️
git-hashsig-create
- ⁉️
git-hashsig-create-fromfile
- ❌
git-hashsig-free
(memory management shouldn't be exposed to Emacs)
ignore
- ⁉️
git-ignore-add-rule
- ⁉️
git-ignore-clear-internal-rules
- ⁉️
git-ignore-path-is-ignored
index
- ⁉️
git-index-add
- ⁉️
git-index-add-all
- ⁉️
git-index-add-bypath
- ⁉️
git-index-add-frombuffer
- ⁉️
git-index-caps
- ⁉️
git-index-checksum
- ⁉️
git-index-clear
- ⁉️
git-index-conflict-add
- ⁉️
git-index-conflict-cleanup
- ⁉️
git-index-conflict-get
- ⁉️
git-index-conflict-iterator-free
- ⁉️
git-index-conflict-iterator-new
- ⁉️
git-index-conflict-next
- ⁉️
git-index-conflict-remove
- ⁉️
git-index-entry-is-conflict
- ⁉️
git-index-entry-stage
- ⁉️
git-index-entrycount
- ⁉️
git-index-find
- ⁉️
git-index-find-prefix
- ❌
git-index-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-index-get-byindex
- ⁉️
git-index-get-bypath
- ⁉️
git-index-has-conflicts
- ⁉️
git-index-new
- ⁉️
git-index-open
- ⁉️
git-index-owner
- ⁉️
git-index-path
- ⁉️
git-index-read
- ⁉️
git-index-read-tree
- ⁉️
git-index-remove
- ⁉️
git-index-remove-all
- ⁉️
git-index-remove-bypath
- ⁉️
git-index-remove-directory
- ⁉️
git-index-set-caps
- ⁉️
git-index-set-version
- ⁉️
git-index-update-all
- ⁉️
git-index-version
- ⁉️
git-index-write
- ⁉️
git-index-write-tree
- ⁉️
git-index-write-tree-to
indexer
- ⁉️
git-indexer-append
- ⁉️
git-indexer-commit
- ❌
git-indexer-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-indexer-hash
- ⁉️
git-indexer-new
libgit2
- ⁉️
git-libgit2-features
- ⁉️
git-libgit2-init
- ⁉️
git-libgit2-opts
- ⁉️
git-libgit2-shutdown
- ⁉️
git-libgit2-version
mempack
- ⁉️
git-mempack-dump
- ⁉️
git-mempack-new
- ⁉️
git-mempack-reset
merge
- ⁉️
git-merge
- ⁉️
git-merge-analysis
- ⁉️
git-merge-base
- ⁉️
git-merge-base-many
- ⁉️
git-merge-base-octopus
- ⁉️
git-merge-bases
- ⁉️
git-merge-bases-many
- ⁉️
git-merge-commits
- ⁉️
git-merge-file
- ⁉️
git-merge-file-from-index
- ⁉️
git-merge-file-init-input
- ⁉️
git-merge-file-init-options
- ⁉️
git-merge-file-result-free
- ⁉️
git-merge-init-options
- ⁉️
git-merge-trees
message
- ⁉️
git-message-prettify
- ⁉️
git-message-trailer-array-free
- ⁉️
git-message-trailers
note
- ⁉️
git-note-author
- ⁉️
git-note-commit-create
- ⁉️
git-note-commit-iterator-new
- ⁉️
git-note-commit-read
- ⁉️
git-note-commit-remove
- ⁉️
git-note-committer
- ⁉️
git-note-create
- ⁉️
git-note-foreach
- ❌
git-note-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-note-id
- ⁉️
git-note-iterator-free
- ⁉️
git-note-iterator-new
- ⁉️
git-note-message
- ⁉️
git-note-next
- ⁉️
git-note-read
- ⁉️
git-note-remove
object
- ❌
git-object--size
(memory management shouldn't be exposed to Emacs) - ⁉️
git-object-dup
- ❌
git-object-free
(memory management shouldn't be exposed to Emacs) - ✔️
git-object-id
- ⁉️
git-object-lookup
- ⁉️
git-object-lookup-bypath
- ⁉️
git-object-lookup-prefix
- ⁉️
git-object-owner
- ⁉️
git-object-peel
- ✔️
git-object-short-id
- ❌
git-object-string2type
(see below) - ❌
git-object-type
(can be covered by a more generalgit-typeof
for all opaque user pointers) - ❌
git-object-type2string
(see above) - ⁉️
git-object-typeisloose
odb
- ⁉️
git-odb-add-alternate
- ⁉️
git-odb-add-backend
- ⁉️
git-odb-add-disk-alternate
- ⁉️
git-odb-backend-loose
- ⁉️
git-odb-backend-one-pack
- ⁉️
git-odb-backend-pack
- ⁉️
git-odb-exists
- ⁉️
git-odb-exists-prefix
- ⁉️
git-odb-expand-ids
- ⁉️
git-odb-foreach
- ❌
git-odb-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-odb-get-backend
- ⁉️
git-odb-hash
- ⁉️
git-odb-hashfile
- ⁉️
git-odb-init-backend
- ⁉️
git-odb-new
- ⁉️
git-odb-num-backends
- ⁉️
git-odb-object-data
- ⁉️
git-odb-object-dup
- ❌
git-odb-object-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-odb-object-id
- ⁉️
git-odb-object-size
- ⁉️
git-odb-object-type
- ⁉️
git-odb-open
- ⁉️
git-odb-open-rstream
- ⁉️
git-odb-open-wstream
- ⁉️
git-odb-read
- ⁉️
git-odb-read-header
- ⁉️
git-odb-read-prefix
- ⁉️
git-odb-refresh
- ⁉️
git-odb-stream-finalize-write
- ❌
git-odb-stream-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-odb-stream-read
- ⁉️
git-odb-stream-write
- ⁉️
git-odb-write
- ⁉️
git-odb-write-pack
oid
Probably none of these functions will be necessary, since we can expose OIDs to Emacs as strings.
- ❌
git-oid-cmp
- ❌
git-oid-cpy
- ❌
git-oid-equal
- ❌
git-oid-fmt
- ❌
git-oid-fromraw
- ❌
git-oid-fromstr
- ❌
git-oid-fromstrn
- ❌
git-oid-fromstrp
- ❌
git-oid-iszero
- ❌
git-oid-ncmp
- ❌
git-oid-nfmt
- ❌
git-oid-pathfmt
- ❌
git-oid-shorten-add
- ❌
git-oid-shorten-free
- ❌
git-oid-shorten-new
- ❌
git-oid-strcmp
- ❌
git-oid-streq
- ❌
git-oid-tostr
- ❌
git-oid-tostr-s
oidarray
- ❌
git-oidarray-free
(memory management shouldn't be exposed to Emacs)
openssl
- ⁉️
git-openssl-set-locking
packbuilder
- ⁉️
git-packbuilder-foreach
- ❌
git-packbuilder-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-packbuilder-hash
- ⁉️
git-packbuilder-insert
- ⁉️
git-packbuilder-insert-commit
- ⁉️
git-packbuilder-insert-recur
- ⁉️
git-packbuilder-insert-tree
- ⁉️
git-packbuilder-insert-walk
- ⁉️
git-packbuilder-new
- ⁉️
git-packbuilder-object-count
- ⁉️
git-packbuilder-set-callbacks
- ⁉️
git-packbuilder-set-threads
- ⁉️
git-packbuilder-write
- ⁉️
git-packbuilder-written
patch
- ❌
git-patch-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-patch-from-blob-and-buffer
- ⁉️
git-patch-from-blobs
- ⁉️
git-patch-from-buffers
- ⁉️
git-patch-from-diff
- ⁉️
git-patch-get-delta
- ⁉️
git-patch-get-hunk
- ⁉️
git-patch-get-line-in-hunk
- ⁉️
git-patch-line-stats
- ⁉️
git-patch-num-hunks
- ⁉️
git-patch-num-lines-in-hunk
- ⁉️
git-patch-print
- ⁉️
git-patch-size
- ⁉️
git-patch-to-buf
pathspec
- ❌
git-pathspec-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-pathspec-match-diff
- ⁉️
git-pathspec-match-index
- ⁉️
git-pathspec-match-list-diff-entry
- ⁉️
git-pathspec-match-list-entry
- ⁉️
git-pathspec-match-list-entrycount
- ⁉️
git-pathspec-match-list-failed-entry
- ⁉️
git-pathspec-match-list-failed-entrycount
- ❌
git-pathspec-match-list-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-pathspec-match-tree
- ⁉️
git-pathspec-match-workdir
- ⁉️
git-pathspec-matches-path
- ⁉️
git-pathspec-new
proxy
- ⁉️
git-proxy-init-options
push
- ⁉️
git-push-init-options
rebase
- ⁉️
git-rebase-abort
- ⁉️
git-rebase-commit
- ⁉️
git-rebase-finish
- ❌
git-rebase-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-rebase-init
- ⁉️
git-rebase-init-options
- ⁉️
git-rebase-inmemory-index
- ⁉️
git-rebase-next
- ⁉️
git-rebase-open
- ⁉️
git-rebase-operation-byindex
- ⁉️
git-rebase-operation-current
- ⁉️
git-rebase-operation-entrycount
refdb
- ⁉️
git-refdb-backend-fs
- ⁉️
git-refdb-compress
- ❌
git-refdb-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-refdb-init-backend
- ⁉️
git-refdb-new
- ⁉️
git-refdb-open
- ⁉️
git-refdb-set-backend
reference
- ❌
git-reference--alloc
(insys
) - ❌
git-reference--alloc-symbolic
(insys
) - ⁉️
git-reference-cmp
- ✔️
git-reference-create
- ✔️
git-reference-create-matching
- ✔️
git-reference-delete
- ✔️
git-reference-dup
- ✔️
git-reference-dwim
- ✔️
git-reference-ensure-log
- ⁉️
git-reference-foreach
- ⁉️
git-reference-foreach-glob
- ⁉️
git-reference-foreach-name
- ❌
git-reference-free
(memory management shouldn't be exposed to Emacs) - ✔️
git-reference-has-log
- ✔️
git-reference-is-branch
- ✔️
git-reference-is-note
- ✔️
git-reference-is-remote
- ✔️
git-reference-is-tag
- ✔️
git-reference-is-valid-name
- ⁉️
git-reference-iterator-free
- ⁉️
git-reference-iterator-glob-new
- ⁉️
git-reference-iterator-new
- ✔️
git-reference-list
- ✔️
git-reference-lookup
- ✔️
git-reference-name
- ✔️
git-reference-name-to-id
- ⁉️
git-reference-next
- ⁉️
git-reference-next-name
- ⁉️
git-reference-normalize-name
- ✔️
git-reference-owner
- ✔️
git-reference-peel
- ✔️
git-reference-remove
- ⁉️
git-reference-rename
- ✔️
git-reference-resolve
- ⁉️
git-reference-set-target
- ✔️
git-reference-shorthand
- ⁉️
git-reference-symbolic-create
- ⁉️
git-reference-symbolic-create-matching
- ⁉️
git-reference-symbolic-set-target
- ✔️
git-reference-symbolic-target
- ✔️
git-reference-target
- ✔️
git-reference-target-peel
- ✔️
git-reference-type
reflog
- ⁉️
git-reflog-append
- ⁉️
git-reflog-delete
- ⁉️
git-reflog-drop
- ⁉️
git-reflog-entry-byindex
- ⁉️
git-reflog-entry-committer
- ⁉️
git-reflog-entry-id-new
- ⁉️
git-reflog-entry-id-old
- ⁉️
git-reflog-entry-message
- ⁉️
git-reflog-entrycount
- ❌
git-reflog-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-reflog-read
- ⁉️
git-reflog-rename
- ⁉️
git-reflog-write
refspec
- ⁉️
git-refspec-direction
- ⁉️
git-refspec-dst
- ⁉️
git-refspec-dst-matches
- ⁉️
git-refspec-force
- ⁉️
git-refspec-rtransform
- ⁉️
git-refspec-src
- ⁉️
git-refspec-src-matches
- ⁉️
git-refspec-string
- ⁉️
git-refspec-transform
remote
- ⁉️
git-remote-add-fetch
- ⁉️
git-remote-add-push
- ⁉️
git-remote-autotag
- ⁉️
git-remote-connect
- ⁉️
git-remote-connected
- ⁉️
git-remote-create
- ⁉️
git-remote-create-anonymous
- ⁉️
git-remote-create-detached
- ⁉️
git-remote-create-with-fetchspec
- ⁉️
git-remote-default-branch
- ⁉️
git-remote-delete
- ⁉️
git-remote-disconnect
- ⁉️
git-remote-download
- ⁉️
git-remote-dup
- ⁉️
git-remote-fetch
- ❌
git-remote-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-remote-get-fetch-refspecs
- ⁉️
git-remote-get-push-refspecs
- ⁉️
git-remote-get-refspec
- ⁉️
git-remote-init-callbacks
- ⁉️
git-remote-is-valid-name
- ⁉️
git-remote-list
- ⁉️
git-remote-lookup
- ⁉️
git-remote-ls
- ⁉️
git-remote-name
- ⁉️
git-remote-owner
- ⁉️
git-remote-prune
- ⁉️
git-remote-prune-refs
- ⁉️
git-remote-push
- ⁉️
git-remote-pushurl
- ⁉️
git-remote-refspec-count
- ⁉️
git-remote-rename
- ⁉️
git-remote-set-autotag
- ⁉️
git-remote-set-pushurl
- ⁉️
git-remote-set-url
- ⁉️
git-remote-stats
- ⁉️
git-remote-stop
- ⁉️
git-remote-update-tips
- ⁉️
git-remote-upload
- ⁉️
git-remote-url
repository
- ❌
git-repository--cleanup
(insys
) - ✔️
git-repository-commondir
- ⁉️
git-repository-config
- ⁉️
git-repository-config-snapshot
- ✔️
git-repository-detach-head
- ⁉️
git-repository-discover
- ⁉️
git-repository-fetchhead-foreach
- ❌
git-repository-free
(memory management shouldn't be exposed to Emacs) - ✔️
git-repository-get-namespace
- ⁉️
git-repository-hashfile
- ✔️
git-repository-head
- ✔️
git-repository-head-detached
- ✔️
git-repository-head-for-worktree
- ✔️
git-repository-head-unborn
- ✔️
git-repository-ident
- ⁉️
git-repository-index
- ✔️
git-repository-init
- ⁉️
git-repository-init-ext
- ⁉️
git-repository-init-init-options
- ✔️
git-repository-is-bare
- ✔️
git-repository-is-empty
- ✔️
git-repository-is-shallow
- ✔️
git-repository-is-worktree
- ⁉️
git-repository-item-path
- ⁉️
git-repository-mergehead-foreach
- ✔️
git-repository-message
- ✔️
git-repository-message-remove
- ❌
git-repository-new
(insys
) - ⁉️
git-repository-odb
- ✔️
git-repository-open
- ✔️
git-repository-open-bare
- ⁉️
git-repository-open-ext
- ⁉️
git-repository-open-from-worktree
- ✔️
git-repository-path
- ⁉️
git-repository-refdb
- ❌
git-repository-reinit-filesystem
(insys
) - ❌
git-repository-set-bare
(insys
) - ❌
git-repository-set-config
(insys
) - ✔️
git-repository-set-head
- ✔️
git-repository-set-head-detached
- ⁉️
git-repository-set-head-detached-from-annotated
- ✔️
git-repository-set-ident
- ⁉️
git-repository-set-index
- ⁉️
git-repository-set-namespace
- ⁉️
git-repository-set-odb
- ⁉️
git-repository-set-refdb
- ✔️
git-repository-set-workdir
- ✔️
git-repository-state
- ✔️
git-repository-state-cleanup
- ❌
git-repository-submodule-cache-all
(insys
) - ❌
git-repository-submodule-cache-clear
(insys
) - ✔️
git-repository-workdir
- ⁉️
git-repository-wrap-odb
reset
- ⁉️
git-reset
- ⁉️
git-reset-default
- ⁉️
git-reset-from-annotated
revert
- ⁉️
git-revert
- ⁉️
git-revert-commit
- ⁉️
git-revert-init-options
revparse
- ⁉️
git-revparse
- ⁉️
git-revparse-ext
- ✔️
git-revparse-single
revwalk
- ⁉️
git-revwalk-add-hide-cb
- ❌
git-revwalk-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-revwalk-hide
- ⁉️
git-revwalk-hide-glob
- ⁉️
git-revwalk-hide-head
- ⁉️
git-revwalk-hide-ref
- ⁉️
git-revwalk-new
- ⁉️
git-revwalk-next
- ⁉️
git-revwalk-push
- ⁉️
git-revwalk-push-glob
- ⁉️
git-revwalk-push-head
- ⁉️
git-revwalk-push-range
- ⁉️
git-revwalk-push-ref
- ⁉️
git-revwalk-repository
- ⁉️
git-revwalk-reset
- ⁉️
git-revwalk-simplify-first-parent
- ⁉️
git-revwalk-sorting
signature
- ⁉️
git-signature-default
- ⁉️
git-signature-dup
- ❌
git-signature-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-signature-from-buffer
- ⁉️
git-signature-new
- ⁉️
git-signature-now
smart
- ⁉️
git-smart-subtransport-git
- ⁉️
git-smart-subtransport-http
- ⁉️
git-smart-subtransport-ssh
stash
- ⁉️
git-stash-apply
- ⁉️
git-stash-apply-init-options
- ⁉️
git-stash-drop
- ⁉️
git-stash-foreach
- ⁉️
git-stash-pop
status
- ⁉️
git-status-byindex
- ⁉️
git-status-file
- ⁉️
git-status-foreach
- ⁉️
git-status-foreach-ext
- ⁉️
git-status-init-options
- ⁉️
git-status-list-entrycount
- ⁉️
git-status-list-free
- ⁉️
git-status-list-get-perfdata
- ⁉️
git-status-list-new
- ⁉️
git-status-should-ignore
strarray
- ❌
git-strarray-copy
- ❌
git-strarray-free
(memory management shouldn't be exposed to Emacs)
stream
- ⁉️
git-stream-register-tls
submodule
- ⁉️
git-submodule-add-finalize
- ⁉️
git-submodule-add-setup
- ⁉️
git-submodule-add-to-index
- ⁉️
git-submodule-branch
- ⁉️
git-submodule-fetch-recurse-submodules
- ⁉️
git-submodule-foreach
- ❌
git-submodule-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-submodule-head-id
- ⁉️
git-submodule-ignore
- ⁉️
git-submodule-index-id
- ⁉️
git-submodule-init
- ⁉️
git-submodule-location
- ⁉️
git-submodule-lookup
- ⁉️
git-submodule-name
- ⁉️
git-submodule-open
- ⁉️
git-submodule-owner
- ⁉️
git-submodule-path
- ⁉️
git-submodule-reload
- ⁉️
git-submodule-repo-init
- ⁉️
git-submodule-resolve-url
- ⁉️
git-submodule-set-branch
- ⁉️
git-submodule-set-fetch-recurse-submodules
- ⁉️
git-submodule-set-ignore
- ⁉️
git-submodule-set-update
- ⁉️
git-submodule-set-url
- ⁉️
git-submodule-status
- ⁉️
git-submodule-sync
- ⁉️
git-submodule-update
- ⁉️
git-submodule-update-init-options
- ⁉️
git-submodule-update-strategy
- ⁉️
git-submodule-url
- ⁉️
git-submodule-wd-id
tag
- ⁉️
git-tag-annotation-create
- ⁉️
git-tag-create
- ⁉️
git-tag-create-frombuffer
- ⁉️
git-tag-create-lightweight
- ⁉️
git-tag-delete
- ⁉️
git-tag-dup
- ⁉️
git-tag-foreach
- ❌
git-tag-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-tag-id
- ⁉️
git-tag-list
- ⁉️
git-tag-list-match
- ⁉️
git-tag-lookup
- ⁉️
git-tag-lookup-prefix
- ⁉️
git-tag-message
- ⁉️
git-tag-name
- ⁉️
git-tag-owner
- ⁉️
git-tag-peel
- ⁉️
git-tag-tagger
- ⁉️
git-tag-target
- ⁉️
git-tag-target-id
- ⁉️
git-tag-target-type
time
- ⁉️
git-time-monotonic
trace
- ⁉️
git-trace-set
transport
- ⁉️
git-transport-dummy
- ⁉️
git-transport-init
- ⁉️
git-transport-local
- ⁉️
git-transport-new
- ⁉️
git-transport-register
- ⁉️
git-transport-smart
- ⁉️
git-transport-smart-certificate-check
- ⁉️
git-transport-smart-credentials
- ⁉️
git-transport-smart-proxy-options
- ⁉️
git-transport-ssh-with-paths
- ⁉️
git-transport-unregister
tree
- ⁉️
git-tree-create-updated
- ⁉️
git-tree-dup
- ⁉️
git-tree-entry-byid
- ⁉️
git-tree-entry-byindex
- ⁉️
git-tree-entry-byname
- ⁉️
git-tree-entry-bypath
- ⁉️
git-tree-entry-cmp
- ⁉️
git-tree-entry-dup
- ⁉️
git-tree-entry-filemode
- ⁉️
git-tree-entry-filemode-raw
- ⁉️
git-tree-entry-free
- ⁉️
git-tree-entry-id
- ⁉️
git-tree-entry-name
- ⁉️
git-tree-entry-to-object
- ⁉️
git-tree-entry-type
- ⁉️
git-tree-entrycount
- ❌
git-tree-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-tree-id
- ⁉️
git-tree-lookup
- ⁉️
git-tree-lookup-prefix
- ⁉️
git-tree-owner
- ⁉️
git-tree-walk
treebuilder
- ⁉️
git-treebuilder-clear
- ⁉️
git-treebuilder-entrycount
- ⁉️
git-treebuilder-filter
- ❌
git-treebuilder-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-treebuilder-get
- ⁉️
git-treebuilder-insert
- ⁉️
git-treebuilder-new
- ⁉️
git-treebuilder-remove
- ⁉️
git-treebuilder-write
- ⁉️
git-treebuilder-write-with-buffer
worktree
- ⁉️
git-worktree-add
- ⁉️
git-worktree-add-init-options
- ❌
git-worktree-free
(memory management shouldn't be exposed to Emacs) - ⁉️
git-worktree-is-locked
- ⁉️
git-worktree-is-prunable
- ⁉️
git-worktree-list
- ⁉️
git-worktree-lock
- ⁉️
git-worktree-lookup
- ⁉️
git-worktree-open-from-repository
- ⁉️
git-worktree-prune
- ⁉️
git-worktree-prune-init-options
- ⁉️
git-worktree-unlock
- ⁉️
git-worktree-validate
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK