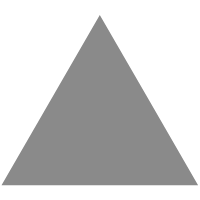
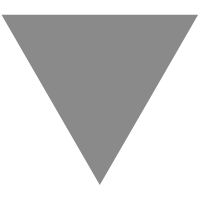
Laravel 5.6 Form Validation Client-Side Using jQuery
source link: https://www.tuicool.com/articles/hit/UVZvArv
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Laravel 5.6 Server Side Validation Example Using Resource Controller
.I am using jquery and JavaScript to validate laravel form client side.You can use any third party javascript libs as well to validate laravel form, But I am creating own method to validate form input fields and display message.
Laravel 5.6 Form Validation
We have created add employee form inPrevious Tutorial, Now we will create generalized javascript method to validate form.
function validateForm(type) { let formname = $('#Employee-'+type); let isValidate = true; formname.find(".require-field").each(function(index, el) { let ele_name = $(el).attr('name'); if ($(el).val() == "") { isValidate = false; $(el).parent('div.form-group').addClass('has-error'); $('div[name='+ele_name+'-error-span]').html('<span class="help-block"><strong>The template '+ele_name+' is required.</strong></span>'); } }); console.log(isValidate); if(!isValidate) { return false; } }
Above method will validate add and edit form page, We just pass form type as a parameter(add or edit).I am finding all required fields of forms and check value, if the input field are required and value is empty then display validation message otherwise return true(all validation rule has been passed).
We will modify add employee form information as per above method.
<form action="{{ route('employee.store') }}" id="Employee-add" method="POST" onsubmit="return validateForm('add')">
modified form id #Employee-add
and called validateForm()
method on onsubmit form event.
Now add '.require-field'
class with in form element, that tells JavaScript validation method – its a required field and validate.
<div class="form-group {{ $errors->has('name') ? ' has-error' : '' }}"> <label class="control-label">Employee Name</label> <input type="text" class="form-control require-field" name="name" value="{{Request::old('name')}}" onkeyup="removeErrors(this)"> @if ($errors->has('name')) <span class="help-block"><strong>{{ $errors->first('name') }}</strong></span> @endif <div name="name-error-span"></div> </div>
I have also associated one more div ‘name-error-span’ with each required input field, This div will use to display validation message.
I also added removeErrors()
method to hide validation failed message on keyup input, if the user has been enter some values into input field.
Till now, above code will validate laravel form and display message but not hide display message, Lets create removeErrors()
method to hide message.
function removeErrors(el){ ele_name = $(el).attr('name'); if ($(el).val() != "") { $(el).parent('div.form-group').removeClass('has-error'); $('div[name='+ele_name+'-error-span]').hide(); } else { $(el).parent('div.form-group').addClass('has-error'); $('div[name='+ele_name+'-error-span]').show(); } }
Conclusion:
We have created laravel form using blade template.I have also added client side validation using jquery to validate form inputs.The validation message are display into span using bootstrap css framework.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK