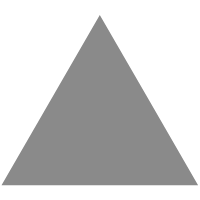
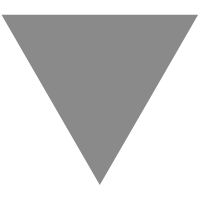
Showing a HERE Map with the Vue.js JavaScript Framework
source link: https://www.tuicool.com/articles/hit/6JZJ3qy
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
When developing a modern web application, very rarely does one use vanilla JavaScript when creating the frontend. HERE offers a JavaScript API for displaying and working with maps, but what if you’re using a modern framework? Previously I had written a tutorial titled, Displaying HERE Maps within your Angular Web Application which demonstrated getting set up with Angular, but what if you’re using another popular framework like Vue.js ?
We’re going to see how to use the HERE Maps API for JavaScript within a web application created with the Vue.js JavaScript framework.
The goal of our application is simple, show a map on the screen, and it can be seen in the image below.
The process involved will include working with native DOM elements, reusable components, and user defined properties that are bound to the map component and dynamically changeable.
The Requirements
To be successful with this project, there are a few requirements that must be met prior:
- You must have a developer account with HERE.
- You must have the Vue CLI installed and configured.
To work with HERE maps and other location services an account must be established. This can be done for free from the HERE Developer Portal . While not absolutely necessary when working with Vue, this particular tutorial will be making use of the Vue CLI . It can be obtained through the Node Package Manager (NPM).
Creating a New Project with the Vue CLI
For simplicity, we’re going to start with a new Vue.js project. With the Vue CLI, execute the following command:
vue create here-project
For this particular project we will not be using anything beyond the defaults when prompted by the stages of the Vue CLI. This means don’t worry about the Vue Router or Vuex.
When the project has been created, you can run it at any time by executing the following:
npm run serve
The above command will serve the application which is great for testing. When you’re ready to deploy the application, you’ll probably want to build it instead of serving it.
Developing a Reusable Map Component with Vue.js
When using vanilla JavaScript, you’re probably familiar with adding map code where it is needed. This causes a lot of duplicate code to exist and isn’t really scalable for the long term. While we could do it this way in a Vue.js application, it makes sense to create a dedicated map component instead.
Within your project, create a src/components/HereMap.vue file with the following code:
<template> <div class="here-map"></div> </template> <script> export default { name: "HereMap", data() { }, props: { }, created() { }, mounted() { } } </script> <style scoped></style>
As you can see in the above code, it is very incomplete. However, notice that we have a <template>
, <script>
, and <style>
block. For the extent of this application, the <style>
block will remain empty, so let’s ignore it going forward.
Because of how simple our UI is going to be, let’s focus on the <template>
first. It should contain the following:
<template> <div class="here-map"> <div ref="map" v-bind:style="{ width: width, height: height }"></div> </div> </template>
There are a few things to note in the above HTML markup. First, notice the ref
attribute. Because we are going to have direct interaction with the DOM component, we need to create a variable that references it. The variable is map
and it will be accessible from our <script>
block when we make it there. Next, notice the v-bind:style
attribute. We’re going to let the user define some dynamic styles. For the object, the key is the HTML style property while the value is actual a variable that will be found in the <script>
block.
Not too bad so far right?
Now we can take a look at the JavaScript that powers the map component. Within the same file, notice that we have a data
method, a created
method, a mounted
method, and a props
object. The data
method and the props
object will both hold variables for our component. However, anything in the props
object will be user defined component attributes.
The props
and data
will look like the following:
<script> export default { name: "HereMap", data() { return { map: {}, platform: {} } }, props: { appId: String, appCode: String, lat: String, lng: String, width: String, height: String }, created() { }, mounted() { } } </script>
The map
and platform
variables found in the data
function will hold information about our map and they will very closely resemble what is found in the official documentation for the HERE Maps API. The props
that we used will be all the attributes that can be bound to the component. They do not need to be double declared in the data
function.
Vue.js has a few lifecycle hooks that are executed when an application or component loads. One of the first is the created
method:
created() { this.platform = new H.service.Platform({ "app_id": this.appId, "app_code": this.appCode }); },
When the created
method executes we will initialize the HERE platform. This will include taking data from our bound properties and setting one of the component variables. Because the map is a DOM component, we need to wait until the components have rendered before we try to work with it, so we can’t do it in the created
method. Instead we can use the mounted
method:
mounted() { this.map = new H.Map( this.$refs.map, this.platform.createDefaultLayers().normal.map, { zoom: 10, center: { lng: this.lng, lat: this.lat } } ); }
In the mounted
method, we use one of our component variables as well as the ref
variable that was set via an attribute in the HTML. Finally, the lat
and lng
data comes from the component attributes.
More information on the Vue.js lifecycle hooks can be found in the official Vue documentation .
Displaying a Map in the Web Application
Now that we have a map component, we need to use it within our application. Given that this is a simple application, we’re going to be using it within the project’s src/App.vue file. Open this file and include the following:
<template> <div id="app"> <HereMap appId="APP-ID-HERE" appCode="APP-CODE-HERE" lat="37.7397" lng="-121.4252" width="100%" height="835px" /> </div> </template> <script> import HereMap from "./components/HereMap.vue" export default { name: 'app', components: { HereMap } } </script> <style> #app { font-family: 'Avenir', Helvetica, Arial, sans-serif; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; text-align: center; color: #2c3e50; } </style>
Again, we have a <template>
, <script>
, and <style>
block. You can choose not to use the default styles, or leave them in. In the above code, it is the defaults. Before we can use the HereMap
component, we need to import it and include it in the <script>
block. Once we’ve done this, we can use it in your HTML. Notice that the component attributes match those that we defined in the props
object of our component JavaScript.
Don’t forget to change the appId
and appCode
with yours before trying to run the application.
Conclusion
You just saw how to use the HERE Maps API for JavaScript to display a map within a web application created with the Vue.js JavaScript framework. As you probably noticed, most of the work that we did was more or less Vue.js configuration and not so much on the map configuration. This is typical for any frontend framework, however, once it is configured, development remains smooth.
If you’re an Angular developer and you want to see this same tutorial for Angular, check out theprevious tutorial that I wrote on the subject.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK