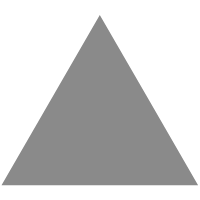
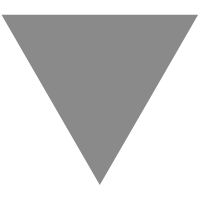
SpriteKit Scenes iOS Tutorial
source link: https://www.tuicool.com/articles/hit/vaqAF32
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
With Sprite Kit you can create multiple scenes, one for each screen of the app. It is also possible to use animation transition between your scenes. In this tutorial we will create two scenes and create a transition. This tutorial is made with Xcode 10 and built for iOS 12.
Open Xcode and select the Game template.

For product name, use IOS12SpriteKitScenesTutorial and then fill out the Organization Name and Organization Identifier with your customary values. Enter Swift as Language and SpriteKit as Game Technology and choose Next.

Go to the GameScene.sks file and delete the hello World label. Select the Scene and in the Attributes inspector change the X and Y values of the Anchor point to 0.

For this tutorial we will need two images which will act as buttons to start the transitioning between our scenes. Download thezipfile, unpack it and add the images to the project.
First we need to create a new scene. Add a new File to the Project and choose iOS -> Source -> Cocoa Touch Class. Choose Next, name the class SecondScene and make it a subscene of SKScene.

Also add a new file to the project. Choose iOS -> Resource -> SpriteKit Scene File and name it Secondscene.sks. Go to the SecondScene.sks file and select the Scene and in the Attributes inspector change the X and Y values of the Anchor point to 0.
The Game template added some code to the project we will not need. Go to the GameScene.swift file and delete all code inside the GameScene class. Next, add the following didMoveToView method
override func didMove(to view: SKView) { backgroundColor = SKColor(red: 0.15, green:0.15, blue:0.3, alpha: 1.0) let button = SKSpriteNode(imageNamed: "nextButton.png") button.position = CGPoint(x: self.frame.size.width/2, y: self.frame.size.height/2) button.name = "nextButton" self.addChild(button) }
The background color is changed and the nextButton is positioned to the center of the scene. Next, we need to respond to a click from this button. Add the touchesBegan(_:with) method
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) { if let touch = touches.first { let location = touch.location(in: self) let nodesarray = nodes(at: location) for node in nodesarray { if node.name == "nextButton" { let secondScene = SecondScene(fileNamed: "SecondScene") let transition = SKTransition.flipVertical(withDuration: 1.0) secondScene?.scaleMode = .aspectFill scene?.view?.presentScene(secondScene!, transition: transition) } } } }
When the image is clicked the scene is transitioned to the second scene using a flipVertical transition with a duration of 1 second. Go to the SecondScene.swift file and add the didMove(to:)View and touchesBegan(_:with:) methods
override func didMove(to view: SKView) { backgroundColor = SKColor(red: 0.15, green:0.15, blue:0.3, alpha: 1.0) let button = SKSpriteNode(imageNamed: "previousbutton.png") button.position = CGPoint(x: self.frame.size.width/2, y: self.frame.size.height/2) button.name = "previousButton" self.addChild(button) } override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) { if let touch = touches.first { let location = touch.location(in: self) let nodesarray = nodes(at: location) for node in nodesarray { if node.name == "previousButton" { let firstScene = GameScene(fileNamed: "GameScene") let transition = SKTransition.doorsCloseHorizontal(withDuration: 0.5) firstScene?.scaleMode = .aspectFill scene?.view?.presentScene(firstScene!, transition: transition) } } } }
The code is almost identical from the GameScene, except now a closing door transition is used. Build and Run the project, press the button to start the transitioning between the two scenes.

You can download the source code of the IOS12SpriteKitScenesTutorial at the ioscreator repository on Github .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK