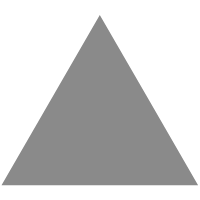
53
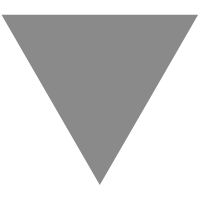
GitHub - adtac/go-lcns: A license key generation and verification library for Go
source link: https://github.com/adtac/go-lcns
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
go-lcns
go-lcns
is a license generation and verification library for Go that uses RSA cryptography underneath. I use this for the enterprise edition of Commento.
Usage
A code snippet speaks a thousand words. Check out godoc for more details.
import "github.com/adtac/go-lcns"
// Read the public and private keys from disk. In practice, you'd be doing only // one of these; the server would read the private key and the client would // read the public key. publicKey, err := ReadPublicKey("testfiles/publickey.crt") privateKey, err := ReadPrivateKey("testfiles/keypair.pem")
// First let's generate licenses with payloads of built-in types (such as int, // string). Usually, these payloads contain some identifying information about the // client. You'll be doing the license key generation on the server side, of course. licenseKeyString, err := GenerateFromPayload(privateKey, "some payload")
// Now on the client side, let's verify the license by making sure the signature // matches and extract the payload. You'll be doing this on the client side. payload, err := VerifyAndExtractPayload(publicKey, licenseKeyString) if err != nil { // The license was probably invalid or corrupt. } fmt.Println(payload) // "some payload"
Using custom structs as payloads is possible, too, but you need to first register the struct. Remember to export all the fields!
type foo struct { Bar string Baz int } x := foo{"bar", 100}
// On the server side, you generate the license key. gob.Register(foo{}) licenseKeyString, err = GenerateFromPayload(privateKey, x)
// On the client side, you verify the license key. Make sure to use the same struct; // you may get panics, errors, and other monsters otherwise. gob.Register(foo{}) payload, err = VerifyAndExtractPayload(publicKey, licenseKeyString) if err != nil { // The license was probably invalid or corrupt. } // You can cast the empty interface returned by VerifyAndExtractPayload like so. fooObject := payload.(foo)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK