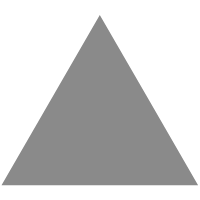
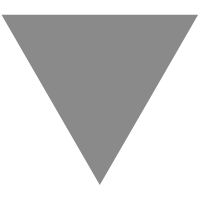
Add Row to Table View iOS Tutorial
source link: https://www.tuicool.com/articles/hit/qiuYraU
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Inside Table Views the data is displayed using rows. These rows can be manipulated, in this tutorial we will add some rows using user input. This tutorial is made with Xcode 10 and built for iOS 12
Open Xcode and create a new Single View App.

For product name, use IOS12AddRowsTableViewTutorial and then fill out the Organization Name and Organization Identifier with your customary values. Enter Swift as Language and choose Next.

Go to the storyboard . Remove the View Controller from the Storyboard and drag a Navigation Controller to the Scene. This will also add the Table View Controller . Select the Navigation Controller and go to The Attribute inspector. In the View Controller section check the "Is Initial View Controller" checkbox.

Select the Table View Cell and go to the Attributes Inspector. In the Table View Cell section set the Identifier to "car Cell" .

Double-click the Title Bar in the Table View Controller and insert the title Cars . Add a Bar button to the right side of the Navigation Bar of Our Table View Controller. Select the bar button item and go to the Attributes Inspector. In the Bar Button Item section change the System Item to “Add”. We will use this button to show a new View Controller where we can add new items. The storyboard should look like this.

Drag a new View Controller on the storyboard to the right of our Table View Controller. Drag a Navigation Bar to the Top of the View.. Add the following to this View Controller.
-
Title of "Add Car"
-
Left Bar Button Item, change the System Item to Cancel in the Attributes Inspector.
-
Right Bar Button Item, change the System Item to Done in the Attributes Inspector.
-
Text Field
The ViewController should look like this.

Next, Ctrl + Drag from the Add button of Our Table View Controller to the View Controller and select Present Modally. This transformation is called a segue, which means when the user clicks the Add button the new View Controller will be placed on top of the Table View.

Since the View Controller is removed from the Storyboard the ViewController.swift file can also be deleted from the project. Add a new file to the project, select iOS->Source->Cocoa Touch Class. Name it CarListViewController and make it a subclass of UITableViewController.

The TableViewController class needs to be linked to The Table View Controller object in the Storyboard. Select it and go the Identity Inspector. In the Custom Class section change the class to ViewController.

Go to CarListViewController.swift and create an array containing the table data.
var cars = [String]() var newCar: String = ""
The cars property is an empty array of Strings and the newCar property is an empty String. In the viewDidLoad method the cars array will be filled.
override func viewDidLoad() { super.viewDidLoad() cars = ["BMW","Audi","Volkswagen"] }
The CarListViewController class contains some boilerplate code. Change the following delegate methods
override func viewDidLoad() { super.viewDidLoad() cars = ["BMW","Audi","Volkswagen"] }
// 1 override func numberOfSections(in tableView: UITableView) -> Int { return 1 } // 2 override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int { return cars.count } // 3 override func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell { let cell = tableView.dequeueReusableCell(withIdentifier: "carCell", for: indexPath) cell.textLabel?.text = cars[indexPath.row] return cell }
-
There is only one section in the Table View so 1 needs to be returned in the numberOfSections(in:) method.
-
The number of rows is equal to the number of items in the cars array so the count property of the array class is used.
-
The name of the car at the current index of the cars array is assigned to the text property of the textLabel property of the current cell.
Build and Runthe project and the car names will be displayed in our TableView.

We can get to the Add Cars screen using the + button, but this isn't functioning yet. Add another file to the project. Choose iOS->Source->Cocoa Touch Class. Name the class CarDetailViewController and make it a subclass of UIViewController.

Select the Add Cars View Controller in the Storyboard, and go to the identity inspector. In the Custom Class section, change the Class to CarDetailViewController . Open the Assistant Editor and make sure the CarDetailViewController.swift file is visible. Ctrl and drag from the Text Field to the CarDetailViewController class and create the following Outlet

Also add a property to hold the car name
var name: String = ""
Currently, there is no way back from the CarDetailViewController to the CarListViewController. To achieve this we make use of the unwind segue. In CarListViewController.swift add the following IBAction methods.
@IBAction func cancel(segue:UIStoryboardSegue) { } @IBAction func done(segue:UIStoryboardSegue) { }
Go to the Storyboard . Ctrl+Drag from the Cancel button to the green exit button on the View Controller and select the cancel: method. Repeat the same for the done button. Now on the Document Outline two unwind segue buttons in the Car Detail View Controller list are displayed.

Select the unwind segues and give it a identifier of cancelSegue and doneSegue in the Attributes inspector. We need these identifiers in our code. Go to CarDetailViewController.swift and add the prepareForSegue method
override func prepare(for segue: UIStoryboardSegue, sender: Any?) { if segue.identifier == "doneSegue" { name = carName.text! } }
When the user presses the done button, the name property is set with the text of our textField. Next implement the Done IBAction method in CarListViewController.swift
@IBAction func done(segue:UIStoryboardSegue) { let carDetailVC = segue.source as! CarDetailViewController newCar = carDetailVC.name cars.append(newCar) tableView.reloadData() }
The CarDetailViewController is set as the source, next the name of the car is added to the cars array. Build and Run the project, add a car in the textfield, the car is added to the Table Vie

You can download the source code of the IOS11ATutorial at the ioscreator repository on Github
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK