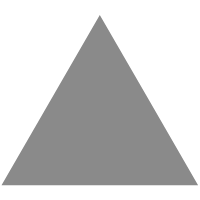
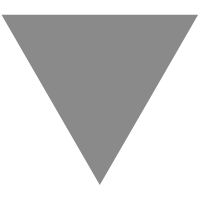
Golang创建最简单的HTTP和HTTPS服务
source link: https://studygolang.com/articles/14947?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Golang可以用很短的代码实现HTTP和HTTPS服务
HTTP服务
HTTP是基于传输层TCP协议的。
package main import ( "net/http" "fmt" "log" ) func main() { http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request){ fmt.Fprint(w, "Hello world") }) log.Fatal(http.ListenAndServe(":5001", nil)) }
HTTPS服务
HTTPS服务不同于HTTP服务,HTTPS是HTTP over SSL或HTTP over TLS。
SSL是“Secure Sockets Layer”的缩写,中文叫做“安全套接层”。它是在上世纪90年代中期,由NetScape公司设计的。为啥要发明 SSL 这个协议捏?因为原先互联网上使用的 HTTP 协议是明文的,存在很多缺点——比如传输内容会被偷窥(嗅探)和篡改。发明 SSL 协议,就是为了解决这些问题。
到了1999年,SSL 因为应用广泛,已经成为互联网上的事实标准。IETF 就在那年把 SSL 标准化。标准化之后的名称改为 TLS 是“Transport Layer Security”的缩写,中文叫做“传输层安全协议”。
很多相关的文章都把这两者并列称呼(SSL/TLS),因为这两者可以视作同一个东西的不同阶段。 参考
要启用HTTPS首先需要创建私钥和证书。
有两种方式生成私钥和证书:
- OpenSSL方式,生成私钥key.pem和证书cert.pem,3650代表有效期为10年
openssl genrsa -out key.pem 2048 openssl req -new -x509 -key key.pem -out cert.pem -days 3650
- Golang标准库crypto/tls里有generate_cert.go,可以生成私钥key.pem和证书cert.pem,host参数是必须的,需要注意的是 默认有效期是1年
go run $GOROOT/src/crypto/tls/generate_cert.go --host localhost
将生成的key.pem、cert.pem和以下代码放在同一目录下
package main import ( "log" "net/http" ) func handler(w http.ResponseWriter, req *http.Request) { w.Header().Set("Content-Type", "text/plain") w.Write([]byte("This is an example server.\n")) } func main() { http.HandleFunc("/", handler) log.Printf("About to listen on 10443. Go to https://127.0.0.1:10443/") // One can use generate_cert.go in crypto/tls to generate cert.pem and key.pem. // ListenAndServeTLS always returns a non-nil error. err := http.ListenAndServeTLS(":10443", "cert.pem", "key.pem", nil) log.Fatal(err) }
(END)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK