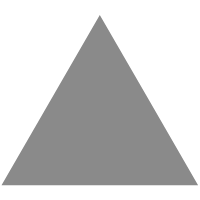
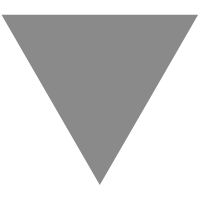
GitHub - uias/Pageboy: ? A simple, highly informative page view controller.
source link: https://github.com/uias/Pageboy
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
TL;DR UIPageViewController done properly.
Pageboy is a simple, highly informative page view controller.
⭐️ Features
- Simplified data source management.
- Enhanced delegation; featuring exact relative positional data and reliable updates.
- Infinite scrolling support.
- Automatic timer-based page transitioning.
- Support for custom page transitions.
? Requirements
Pageboy requires iOS 9 / tvOS 10 or above; and is written in Swift 4.2.
? Installation
CocoaPods
Pageboy is available through CocoaPods. To install it, simply add the following line to your Podfile:
pod 'Pageboy', '~> 2.6'
And run pod install
.
Carthage
Pageboy is available through Carthage. Simply install carthage with Homebrew using the following command:
$ brew update $ brew install carthage
Add Pageboy to your Cartfile
:
github "uias/Pageboy" ~> 2.6
? Usage
Getting Started
- Create an instance of a
PageboyViewController
and provide it with aPageboyViewControllerDataSource
.
class PageViewController: PageboyViewController, PageboyViewControllerDataSource { override func viewDidLoad() { super.viewDidLoad() self.dataSource = self } }
- Implement the
PageboyViewControllerDataSource
functions.
func numberOfViewControllers(in pageboyViewController: PageboyViewController) -> Int { return viewControllers.count } func viewController(for pageboyViewController: PageboyViewController, at index: PageboyViewController.PageIndex) -> UIViewController? { return viewControllers[index] } func defaultPage(for pageboyViewController: PageboyViewController) -> PageboyViewController.Page? { return nil }
- Enjoy.
Delegation
Unfortunately, UIPageViewController
doesn't provide the most useful delegate methods for detecting positional data. PageboyViewControllerDelegate
provides a number of functions for being able to detect where the page view controller is, and where it's headed.
willScrollToPageAtIndex
The page view controller is about to embark on a transition to a new page.
func pageboyViewController(_ pageboyViewController: PageboyViewController, willScrollToPageAt index: Int, direction: PageboyViewController.NavigationDirection, animated: Bool)
didScrollToPosition
The page view controller was scrolled to a relative position along the way transitioning to a new page. Also provided is the direction of the transition.
func pageboyViewController(_ pageboyViewController: PageboyViewController, didScrollTo position: CGPoint, direction: PageboyViewController.NavigationDirection, animated: Bool)
didScrollToPage
The page view controller has successfully completed a scroll transition to a page.
func pageboyViewController(_ pageboyViewController: PageboyViewController, didScrollToPageAt index: Int, direction: PageboyViewController.NavigationDirection, animated: Bool)
didReload
The page view controller has reloaded its child view controllers.
func pageboyViewController(_ pageboyViewController: PageboyViewController, didReloadWith currentViewController: UIViewController, currentPageIndex: PageboyViewController.PageIndex)
⚡️ Extras
-
reloadPages()
- Reload the view controllers in the page view controller. (Refreshes the data source). -
scrollToPage()
- Scroll the page view controller to a new page programatically.public func scrollToPage(_ pageIndex: PageIndex, animated: Bool, completion: PageTransitionCompletion? = nil)
-
.navigationOrientation
- Whether to orientate the pages horizontally or vertically. -
.isScrollEnabled
- Whether or not scrolling is allowed on the page view controller. -
.isInfiniteScrollEnabled
- Whether the page view controller should infinitely scroll at the end of page ranges. -
.currentViewController
- The currently visible view controller if it exists. -
.currentPosition
- The exact current relative position of the page view controller. -
.currentIndex
- The index of the currently visible page. -
.showsPageControl
- Whether to show the built-in page control. -
.parentPageboy
- Access the parentPageboyViewController
from any childUIViewController
.class ChildViewController: UIViewController { func doSomething() { parentPageboy?.scrollToPage(.next, animated: true) } }
Transitioning
Pageboy also provides custom animated transition support. This can be customised via the .transition
property on PageboyViewController
.
pageboyViewController.transition = Transition(style: .push, duration: 1.0)
The following styles are available:
.push
.fade
.moveIn
.reveal
Note: By default this is set to nil
, which uses the standard animation provided by UIPageViewController
.
Auto Scrolling
PageboyAutoScroller
is available to set up timer based automatic scrolling of the PageboyViewController
:
pageboyViewController.autoScroller.enable()
Support for custom intermission duration and other scroll behaviors is also available.
??? About
- Created by Merrick Sapsford (@MerrickSapsford)
- Contributed to by a growing list of others.
❤️ Contributing
Bug reports and pull requests are welcome on GitHub at https://github.com/uias/Pageboy.
??♂️ License
The library is available as open source under the terms of the MIT License.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK