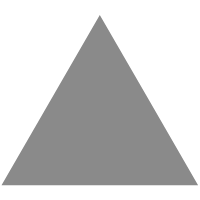
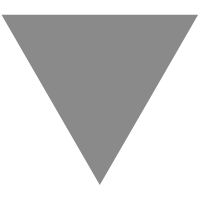
Detect Cryptocurrency Market Trends Using PHP: Exponential Moving Averages
source link: https://www.tuicool.com/articles/hit/niqqmuI
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
What are moving averages?
Moving averages allow you to visualise the price action for a financial instrument over a period of time. It’s widely used in technical analysis and referred to as a “lagging” indicator as it’s based on historical prices.
The two commonly used averages are simple moving average (SMA) and exponential moving average (EMA). The SMA is the simple average of a security over a specific period. The EMA is similar, but attributes greater weight to more recent prices.
Examples of SMA and EMA
The easiest way to understand SMA and EMA is looking at an example.
We’ll take the closing prices of a fictional security over the last 15 days as follows:
Week 1: 10, 12, 15, 11, 18
Week 2: 21, 17, 15, 10, 7
Week 3: 8, 9, 15, 17, 20
In this example, a 10-day SMA would be the average closing price of the previous 10 days. The SMA for the day after would be the previous 10 days, dropping the first day off the list. And so on.

The EMA is slightly more involved. It involves calculating the weighting multiplier, before determining the EMA by using the price, weighting value, and the previous day’s EMA.
Fortunately, you don’t need to worry about the underlying arithmetic involved. PHP has a library called Trader for taking care of technical analysis calculations.
The 8-day and 21-day EMA

The 8-day and 21-day EMAs are very popular short-term indicators used in both the stock market and cryptocurrency market.
The primary signals are:
- When the 8-day line crosses above the 21-day. This indicates a bullish crossover, and that the price will likely increase (buy signal).
- When the 8-day line crosses below the 21-day. This indicates a bearish crossover, and that the price will likely decrease (sell signal).
Looking at the chart above, I’ve marked where the 8-day (blue) and 21-day (red line) cross, resulting in the trend.
A word of caution
I am not a financial advisor, and none of the content in this article should be construed as financial advice. The crypto market is highly volatile, and you should do your own thorough research before making any investments.
It’s also important to note that technical analysis is not perfect. Because the market is driven by psychology, some signals could be completely off. That’s why it’s important to make use of various indicators at the same time (e.g. RSI and Volume). You would then look for confluence, that is, several indicators all showing a similar trend. Even then you can never be 100% sure.
Technical analysis is not a silver bullet.
Getting your PHP environment ready
We’ll be using the PHP Trader extension to calculate the EMA. You can confirm it’s installed by checking the result of phpinfo()
:

The historical price data is available via the excellent CryptoCompare API . We’ll be using their historical data API. The following request will show us the USD prices for BTC for the last 60 days:
https://min-api.cryptocompare.com/data/histoday?fsym=BTC&tsym=USD&limit=60

It regards the closing time as 12:00 GMT, and the time
field returns the Unix timestamp (epoch time).
Determine the EMAs
Add the following code to read the response from the CryptoCompare API:
<?php
$response = json_decode(file_get_contents('https://min-api.cryptocompare.com/data/histoday?fsym=BTC&tsym=USD&limit=60'));
This will return a response as follows if you do print_r($response)
:

Next, we’ll add the closing prices to an array:
$prices = [];
foreach ($response->Data as $v) { $prices[] = $v->close; }
With these prices saved, we can now make use of the trader_ema() PHP function to determine the 8-day and 21-day EMAs:
$ema8 = trader_ema($prices, 8); $ema21 = trader_ema($prices, 21);
A quick word of caution: by default, the Trader extension uses a precision of 3 decimals. To work with satoshi or coins that display to several decimals like XRP and XLM, you can either set the precision at run-time:
ini_set('trader.real_precision', '8');
Or within the php.ini file:
trader.real_precision=8
Now that we have our arrays of the EMAs, we will grab the last two of each to set the previous day’s EMA and current EMA:
$current_8 = array_pop($ema8); $current_21 = array_pop($ema21); $previous_8 = array_pop($ema8); $previous_21 = array_pop($ema21);
We can now simply do a check to see if a cross occurred, and react accordingly:
if ($current_8 > $current_21 && $previous_8 < $previous_21) { echo 'Buy'; } elseif ($current_8 < $current_21 && $previous_8 > $previous_21) { echo 'Sell'; } else { echo 'Do Nothing'; }
This is the full, final source code with some outputs:
<?php
ini_set(‘trader.real_precision’, ‘8’);
$response = json_decode(file_get_contents(‘<a href="https://min-api.cryptocompare.com/data/histoday?fsym=BTC&tsym=USD&limit=60%27" data-href="https://min-api.cryptocompare.com/data/histoday?fsym=BTC&tsym=USD&limit=60'" rel="nofollow noopener" target="_blank">https://min-api.cryptocompare.com/data/histoday?fsym=BTC&tsym=USD&limit=60'</a>));
$prices = [];
foreach ($response->Data as $k => $v) { $prices[] = $v->close; }
$ema8 = trader_ema($prices, 8); $ema21 = trader_ema($prices, 21);
$current_8 = array_pop($ema8); $current_21 = array_pop($ema21); $previous_8 = array_pop($ema8); $previous_21 = array_pop($ema21);
echo ‘Current 8-day: ‘ . $current_8 . “\n”; echo ‘Current 21-day: ‘ . $current_21 . “\n”; echo ‘Previous 8-day: ‘ . $previous_8 . “\n”; echo ‘Previous 21-day: ‘ . $previous_21 . “\n”;
if ($current_8 > $current_21 && $previous_8 < $previous_21) { echo ‘Buy’; } elseif ($current_8 < $current_21 && $previous_8 > $previous_21) { echo ‘Sell’; } else { echo ‘Do Nothing’; }
Real world uses
A simple real-world application for this, would be incorporating it into a trading bot. You could set it to run daily using a cron on a server. Run it after the latest closing price to detect if there was a crossover.
You can integrate with an exchange like Binance, and execute trades via their API if your bot spots certain trends. As mentioned before, try find confirmation from at least three different indicators to minimise your risk.
In the upcoming articles, we’ll be looking at more indicators and how they can be used to determine cryptocurrency market trends.
Upgrade your web dev skills!
Sign up to my newsletter where I’ll share insightful web development and blockchain-related articles to supercharge your skills.

Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK