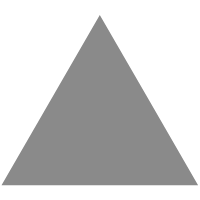
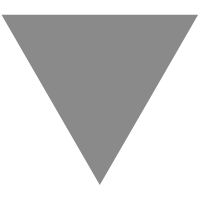
ReasonML Standard Library
source link: https://www.tuicool.com/articles/hit/An6bMnI
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Intro
Hello again, and welcome back to another installment in our series on learning ReasonML. So far we have learned about what ReasonML is, what its place is in the developer ecosystem, and we have learned about the fundamentals of coding with the language itself. Now we're going to change gears and put today's focus on ReasonML's standard library.
The standard library provides a lot of functionality straight out of the box. We can carry out operations over data types and information structures, interact with the file system, manipulate strings, work with complex numbers, and make full use of ReasonML's full potential as a functional language. However, we won't get there by just talking about how awesome it is, let's get started.
Introduction to the standard library.
First things first, we need to take a moment to look at the default print methods that are used by ReasonML. Let's run a quick code example so we can see what we're dealing with.
print_int(3); print_char('a'); print_string("a string"); print_endline("another string"); print_newline(); Js.log(1); Js.log('a'); Js.log("hello everybody");
So you may be thinking "that's an awful lot of print methods, what's the deal here?" Well, this first group is part of the ReasonML native print methods. These can be used on code that will be compiled to Javascript or straight to native assembly code. You'll also notice that we have to choose our print methods specific to the data type we are printing. Also we do have to manually dictate when we will start a new line ourselves.
The next group is part of the standard library's Js module. This code will only work for
projects being compiled to Javascript as it is a literal replication of the console.log
in the Javascript world.
Now it's time to move forward with the next area of focus and this will be the String module. Let's write out some code for this and we will go over some of its offerings.
/* String module */ let x: string = "hello"; let z = ["blah", "blah blah", "blah again"]; let length: int = String.length(x); let upper: string = String.uppercase(x); let capitalize: string = String.capitalize(x); let get: char = String.get(x, 3); let concatenate: string = String.concat(" + ", z); let containsOne: bool = String.contains(x, 'e'); let containsTwo: bool = String.contains(x, 'z'); Js.log(x); Js.log(length); Js.log(upper); Js.log(capitalize); Js.log(get); Js.log(concatenate); Js.log(containsOne); Js.log(containsTwo);
So now we're getting to see a bit of what kind of operations we can do with our String module. You can see that most of these operations are fairly self explanatory. This isn't a full exhaustive list of every option that the String module provides but it gives a good idea of some of what at least I view to be the most useful ones.
Now it's time to take a look at the List module which, no surprise, is a set of functionalities that we can apply to our lists. Let's check it out.
/* list module */ let a = [1, 2, 3, 4, 5]; let double = value => value * 2; let newList = List.map(double, a); let print = value => print_int(value); Js.log(List.length(a)); Js.log(List.iter(print, a)); Js.log(List.iter(print, newList)); for(item in List.nth(a, 0) to List.nth(a, List.length(a) - 1)) { Js.log(item); };
Great, now let's dig in to this. First it's worth noting the creation of our basic list
here. This leads us to our first method of interest which would be the the List.map
function. It operates just like in other functional or functional inspired languages like
Elixir or Javascript. It takes a function an applies it to each value in a list then
returns the list. In this case, we double every integer value within a list.
Next we have the self-explanatory List.length
method but now it's worth turning our
attention to the List.iter
method. This method simply provides us with a way to iterate
through our items in a list to do a particular job. Unlike the map
method. This one
does not return a new list so this makes the method good for things like we're doing here
which is printing off every value in the list.
Also, you may remember this for loop from yesterday and seeing this List.nth
method.
This is just allowing us to grab the index of a particular place in the list which in this
case enables our for loop to know where to start and where to finish in the scheme of this
for loop's actions.
Now that we have covered List it's now time to cover Array. Let's write some quick code so we can see what kind of things Array has to offer.
/* Array module */ let myArray = [|1, 2, 3, 4, 5|]; let triple = value => value * 3; let length = Array.length(myArray); let get = Array.get(myArray, 2); let set = Array.set(myArray, 1, 20); let map = Array.map(triple, myArray); let copy = Array.copy(myArray); let append = Array.append(myArray, map); let printArrayValue = value => Js.log(value); Array.iter(printArrayValue, myArray); Array.iter(printArrayValue, map); Array.iter(printArrayValue, append);
Great, you'll see here that the methods aren't too different from what we were seeing in the List module. You may recognize map and iter which do the exact same thing as we saw before. However, remembering that Array is in fact mutable, you can see we do have a set method for our array. We just pick the index, then choose what value will go in there. We also have this copy method which copies the array then we have append which is intended to quite literally append two different arrays together. Not too bad.
Conclusion
Good job, we may have only scratched the surface of what the standard library has to offer but this gives you a good start with what we have available to work with. In just a few short lessons we have covered quite a bit. We've just quickly covered the fundamentals of ReasonML, enough to get you off the ground and able to start experimenting with different projects that you may have in mind. I want to wish you the best as you go forward and I sincerely hope this lesson has helped you progress in your understanding of ReasonML and what it has to offer for you.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK