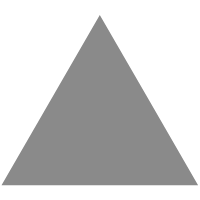
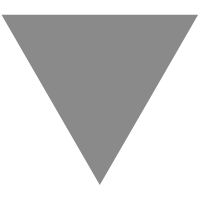
A Look at Arrays and Generics in Java
source link: https://www.tuicool.com/articles/hit/zAveQni
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In this post, I will attempt to explain the problem of using arrays and generics in Java. Let's get started!
Generics was first introduced in JDK5, and all its following libraries have continued to include generics. For a brief introduction on generics, I want to point out that generics provide compile-time type safety. Generics is tough, and I will not dive too deep into the world of generics, but I will show you a basic example using a "ClassCastException," which is extraordinarily tough to debug.
Example
1) First, I create an abstract class Foo<T>
public abstract class Foo<T> { abstract void add(T... elements); void addUnlessNull(T... elements) { for (T element : elements) { if (element != null) add(element); } } }
2) Next, we implement a String version of Foo
:
import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class StringFoo extends Foo<String> { private List<String> list = new ArrayList<>(); @Override void add(String... elements) { list.addAll(Arrays.asList(elements)); } public static void main(String[] args) { StringFoo ss = new StringFoo(); ss.addUnlessNull("Hello", "World"); // Class Cast Exception } }
To my surprise, when I ran this code that supposedly compiles safely, it threw a ClassCastException
out of nowhere, and I am stuck. Now, check the below stack trace:
Exception in thread "main" java.lang.ClassCastException: [Ljava.lang.Object; cannot be cast to [Ljava.lang.String; at StringFoo.add(StringFoo.java:1) at Foo.addUnlessNull(Foo.java:9) at StringFoo.main(StringFoo.java:17)
If you trace the stack log, you will get:
"StringFoo.add(StringFoo.java:1)"
This looks at the first line import java.util.ArrayList
, which is misleading and doesn't help us achieve what we are trying to accomplish. So, what might be happening under the hood?
This could be one or more of the following:
-
Erasure,
-
Varargs,
-
Or, the bridge method
Let me briefly explain each of these:
Erasure: Erasure converts each of T in the Foo<T> class to the lower bound of the T , i.e. the object at compile time. So, at runtime, the JVM has no knowledge of T; all it sees is the object.
Varargs: All varargs parameters are packaged in arrays. In the case of object[]
, you will need to add T
elements that get converted to the add Object[]
elements at compile time. As a rule of thumb, avoid mixing arrays and generics.
Bridge method: This is the most mysterious method of all. Let's see what happens after compiling the code.
public abstract class Foo<T> { abstract void add(T... elements); //Really Object[] after erasure void addUnlessNull(T... elements) { // Really Object[] after erasure for (T element : elements) { if (element != null) add(element); } } } import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class StringFoo extends Foo<String> { private List<String> list = new ArrayList<>(); /** Synthetic bridge method - not present in source! */ /* * void add(Object[] a) { // overrides abstract method { add(String[] a) // * Really throws ClassCastException } */ @Override void add(String... elements) { list.addAll(Arrays.asList(elements)); } public static void main(String[] args) { StringFoo ss = new StringFoo(); ss.addUnlessNull("Hello", "World"); // Class Cast Exception } }
The compiler checks that in abstract class Foo<T>
there is added to the Object[]
elements, but the implementing class StringFoo
doesn't have a specific type of implementation. So, the compiler, in its infinite wisdom and mercy, creates a Bridge method:
void add(Object[] a) { add(String[] a) ; }
This above code really tries to cast an Object[]
to a String[]
and, then, throws a ClassCastException
.
The moral to the story here is: don't mix arrays and generics!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK