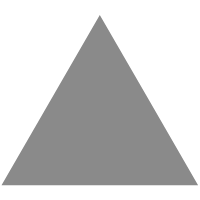
49
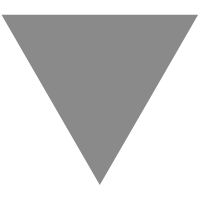
golang中使用mongo
source link: https://studygolang.com/articles/14055?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
笔者使用的mongo驱动是mgo, 这个使用的人比较多,文档也比较齐全
- 官网地址: http://labix.org/mgo
- 文档地址: https://godoc.org/labix.org/v2/mgo
- 源码地址: https://github.com/go-mgo/mgo
1. mgo包安装
go get gopkg.in/mgo.v2
但是貌似现在从gopkg.in下载不了,迂回一下,先从github上下载
go get github.com/go-mgo/mgo
下载好了之后,在$GOPATH/src/下面创建文件夹gopkg.in/mgo.v2, 然后将github.com/go-mgo/mgo的内容,拷贝到gopkg.in/mgo.v2
2. 测试代码
// mongo_test project main.go package main import ( "fmt" "math/rand" "time" "gopkg.in/mgo.v2" "gopkg.in/mgo.v2/bson" ) type GameReport struct { // id bson.ObjectId `bson:"_id"` Game_id int64 Game_length int64 Game_map_id string } func err_handler(err error) { fmt.Printf("err_handler, error:%s\n", err.Error()) panic(err.Error()) } func main() { dail_info := &mgo.DialInfo{ Addrs: []string{"127.0.0.1"}, Direct: false, Timeout: time.Second * 1, Database: "game_report", Source: "admin", Username: "test1", Password: "123456", PoolLimit: 1024, } session, err := mgo.DialWithInfo(dail_info) if err != nil { fmt.Printf("mgo dail error[%s]\n", err.Error()) err_handler(err) } defer session.Clone() // set mode session.SetMode(mgo.Monotonic, true) c := session.DB("game_report").C("game_detail_report") r := rand.New(rand.NewSource(time.Now().UnixNano())) report := GameReport{ // id: bson.NewObjectId(), Game_id: 100, Game_length: r.Int63() % 3600, Game_map_id: "hello", } err = c.Insert(report) if err != nil { fmt.Printf("try insert record error[%s]\n", err.Error()) err_handler(err) } result := GameReport{} var to_find_game_id int64 = 100 err = c.Find(bson.M{"game_id": to_find_game_id}).One(&result) if err != nil { fmt.Printf("try find record error[%s]\n", err.Error()) err_handler(err) } fmt.Printf("res, game_id[%d] length[%d] game_map_id[%s]\n", to_find_game_id, result.Game_length, result.Game_map_id) // try find all report var results []GameReport err = c.Find(bson.M{}).All(&results) if err != nil { fmt.Printf("try game all record of game_detail_report error[%s]\n", err.Error()) err_handler(err) } result_count := len(results) fmt.Printf("result count: %d\n", result_count) for i, report := range results { fmt.Printf("index: %d, report{ game_id: %d, game_length: %d, game_map_id: %s}\n", i, report.Game_id, report.Game_length, report.Game_map_id) } }
这样要注意的一点是 GameReport 里面的字段都要首字母大写,否则不会写入mongo
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK