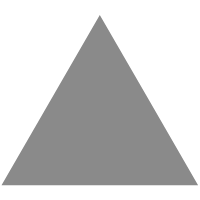
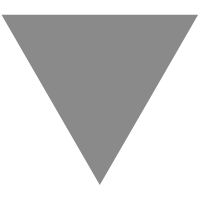
设计模式之责任链模式
source link: http://coderlengary.github.io/2018/08/08/DesignModeChain/?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
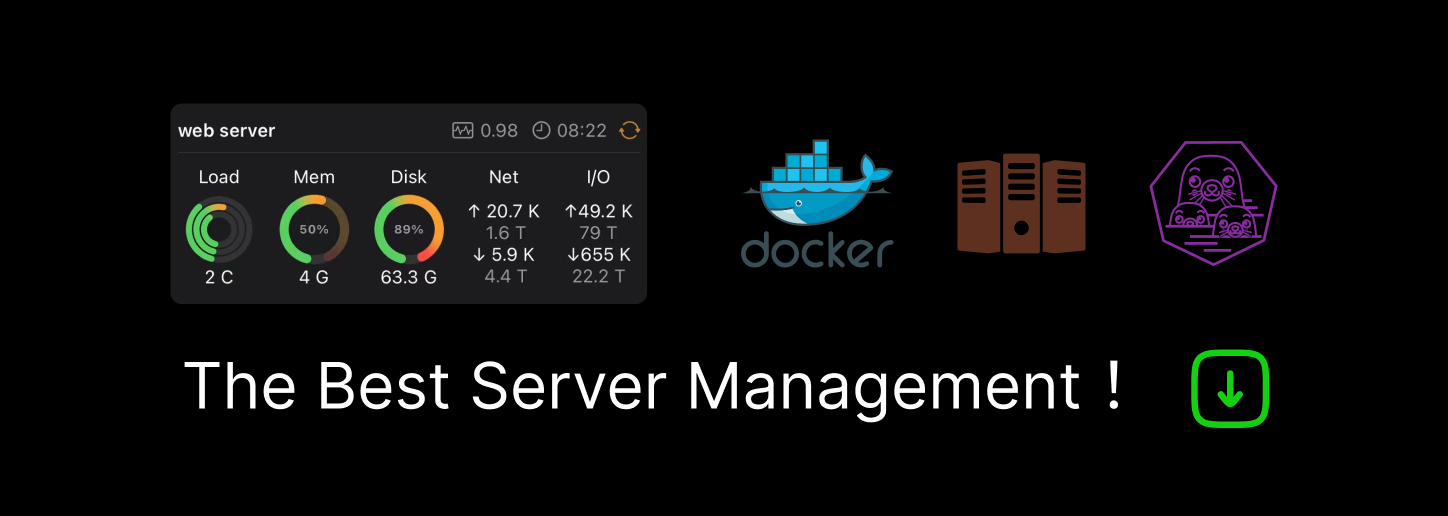
职责链模式(Chain of Responsibility):使多个对象都有机会处理同一个请求,从而避免请求的发送者和接收者之间的耦合关系。将这些对象连成一条链,并沿着这条链传递该请求,直到有一个对象处理它为止。
在日常生活中,这种情景处处可见,我们向上级请假,一旦天数超过上级的限制,上机就要再向他的上级请求批示。一级一级往上传。

责任链模式有如下角色:
- Ratify:处理事务的抽象角色
- Chain:抽象链,传递事务的抽象角色
- RealChain:抽象链的具体实现
我们举一个请假的例子,当组里一个成员要请假的时候,由他的小组领导人先处理,小组领导人批不了假,就交给经理处理,经理处理不了,就交给部门领导人处理。
假条类:
public class Request { private String name; private int days; public Request(String name, int days){ this.name = name; this.days = days; } public int getDays(){ return days; } }
请假结果类:
public class Result { public boolean isRatify; public String info; public Result() { } public Result(boolean isRatify, String info) { super(); this.isRatify = isRatify; this.info = info; } public boolean isRatify() { return isRatify; } public void setRatify(boolean isRatify) { this.isRatify = isRatify; } public String getReason() { return info; } public void setReason(String info) { this.info = info; } @Override public String toString() { return "Result [isRatify=" + isRatify + ", info=" + info + "]"; } }
链接口:
public interface Chain { Request getRequest(); Result proceed(Request request, int index); int getCurrentIndex(); }
具体链:
public class RealChain implements Chain{ private Request request; private List<Ratify> ratifyList; private int index; public RealChain(List<Ratify> ratifyList, Request request){ this.ratifyList = ratifyList; this.request = request; } //分发方法 @Override public Result proceed(Request request, int index) { this.index = index; Result result = null; if(index < ratifyList.size()){ Ratify ratify = ratifyList.get(index); result = ratify.deal(this); } return result; } @Override public Request getRequest(){ return request; } @Override public int getCurrentIndex(){ return index; } }
Ratify接口:
public interface Ratify{ Result deal(Chain chain); }
Ratify具体实现:
public class GroupLeader implements Ratify { @Override public Result deal(Chain chain) { Result result = null; Request request = chain.getRequest(); if(request.getDays()>2){ System.out.println("GroupLeader无法解决,转交给上级"); int currentIndex = chain.getCurrentIndex()+1; return chain.proceed(request, currentIndex); } return new Result(true, "已经由GroupLeader解决"); } } public class Manager implements Ratify { @Override public Result deal(Chain chain) { Result result = null; Request request = chain.getRequest(); if(request.getDays()>5){ System.out.println("Manager无法解决,转交给上级"); int currentIndex = chain.getCurrentIndex()+1; return chain.proceed(request, currentIndex); } return new Result(true, "已经由DepartmentHeader解决"); } } public class DepartmentHeader implements Ratify { @Override public Result deal(Chain chain) { Request request = chain.getRequest(); if(request.getDays()>7){ return new Result(true, "不接受请假"); } return new Result(true, "已经由DepartmentHeader解决"); } }
责任链模模式工具类:
public class ChainOfResponsibilityClient { private ArrayList<Ratify> ratifies; public ChainOfResponsibilityClient(){ ratifies = new ArrayList<Ratify>(); } public void add(Ratify ratify){ ratifies.add(ratify); } public Result execute(Request request){ ArrayList<Ratify> arrayList = new ArrayList<Ratify>(); arrayList.addAll(ratifies); arrayList.add(new GroupLeader()); arrayList.add(new Manager()); arrayList.add(new DepartmentHeader()); RealChain realChain = new RealChain(arrayList, request); return realChain.proceed(request, 0); } }
客户端:
public class JavaApplication51 { /** * @param args the command line arguments */ public static void main(String[] args) { Request request = new Request("张三",6); ChainOfResponsibilityClient client = new ChainOfResponsibilityClient(); Result result = client.execute(request); System.out.println("结果:" + result.toString()); } }
结果:
GroupLeader无法解决,转交给上级 Manager无法解决,转交给上级 结果:Result [isRatify=true, info=已经由DepartmentHeader解决]
Recommend
-
117
今天来说说程序员小猿和产品就关于需求发生的故事。前不久,小猿收到了产品的需求。 产品经理:小猿,为了迎合大众屌丝用户的口味,我们要放一张图,要露点的。 小猿:......露点?你大爷的,让身为正义与纯洁化身的我做这种需求,还露点。 产品经理:误会误会,是...
-
107
责任链模式又称为职责链模式,在23种设计模式中归类为行为型模式。行为型模式可以分为类行为型模式和对象行为型模式。 类行为型模式使用继承关系在几个类之间分配行为,类行为型模式主要通过多态等方式来分配父类与子类的职责。
-
22
责任链,我感觉对就根据需求动态的组织一些工作流程,比如完成一件事有5个步骤,而第1步,第2步,第3步它们的顺序可以在某些时候是不固定的,而这就符合责任链的范畴,我们根据需求去设计我们的这些链条,去自己指定它们的执行顺序,下面看...
-
29
责任链模式 GitHub代码链接 1. 简介 Chain of Responsibility Pattern为请求创建一个接受者对...
-
7
Go设计模式: 责任链模式 今天我们来介绍责任链模式,从名字可以看出来,它应当是一系列的操作。的确如此,看看维基百科的定义: 责任链模式在面向对象程式设计里是一种软件设计模式,它包含了一些命令对象和一系列的处理对象。...
-
18
手撸golang 行为型设计模式 责任链模式 缘起 最近复习设计模式 拜读谭勇德的<<设计模式就该这样学>> 本系列笔记拟采用golang练习之 责任链模式 责任链模式(Chain of...
-
4
设计模式-责任链模式 作为一个上班族,我们可能会经常听到“管理流程混乱”,“职责边界...
-
7
使多个对象都有机会处理请求,从而避免请求的发送者和接受者之间耦合关系,将这些对象连成一条链,并沿着这条链传递请求,直到有一个对象处理它为止Chain of Responsibility模式的应用场合在于“一个请求可能有多个接受者,但是最后真正的接受者只有...
-
23
写在前面记录学习设计模式的笔记提高对设计模式的灵活运用学习地址https://www.bilibili.com/vide...
-
3
责任链模式(Chain Of Responsibility Design Pattern),也叫做职责链,是将请求的发送和接收解耦,让多个接收对象都有机会处理这个请求。当有请求发生时,可将请求沿着这条链传递,直到有对象处理它为止。
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK