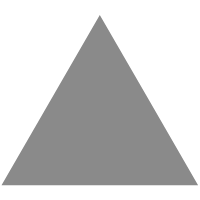
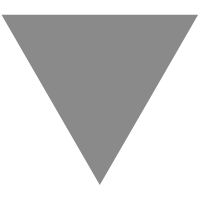
MongoDB and Python
source link: https://www.tuicool.com/articles/hit/RreqEz7
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python is used in many applications, mainly due to its flexibility and availability of various libraries. It works for just about any types of scenarios. This also suggests, it is often coupled with database systems. MongoDB, a NoSql. The intentions of this blog is to show through examples how python can be used to interact with MongoDB. We will specifically use pymongo, a library built by Mongo developers to interact with the MongoDB.
MongoDB
MongoDB is a free and open-source cross-platform document-oriented NoSQL database. It uses JSON like documents. This also entails the flexibility of data and doesn’t require a schema.
Installation of MongoDB
Follow the official documentation to install MongoDB.
- Linux : https://docs.mongodb.com/manual/administration/install-on-linux/
- macOS: https://docs.mongodb.com/manual/tutorial/install-mongodb-on-os-x/
- Windows: https://docs.mongodb.com/manual/tutorial/install-mongodb-on-windows/
pymongo
PyMongo is a Python distribution containing tools for working with MongoDB, and is the recommended way to work with MongoDB from Python. There are various other libraries for the ease of interaction that provide higher level abstractions and also perform document validation, etc. One such library is MongoEngine. MongoEngine is an object document mapper (ODM), which is roughly equivalent to a SQL-based object relational mapper (ORM). We will stick to the official library for MongoDB in this blog.
Installation of pymongo
pip install pymongo
Establishing a connection
>>> from pymongo import MongoClient >>> client = MongoClient() # defaults to host="localhost", port=27017
A helper function to create a connection
from pymongo import MongoClient def mongo_connection(host="localhost", port=27017, username=None, password=None): if username and password: mongo_uri = 'mongodb://{user}:{password}@{host}:{port}'.format(user=username, password=password, host=host, port=port) return MongoClient(mongo_uri) return MongoClient('mongodb://{host}:{port}'.format(host=host, port=port))
As seen in the above snippet, you could also construct an URI and pass it onto the MongoClient class and get the connection.
Selecting Database
There are two ways to select databases from the MongoClient instance. You could either access it as an attribute of the MongoClient instance or in a more dictionary styled construct on the instance. The later is generally useful when you receive the database name as a parameter to some function.
db = client.example_db
OR
db = client['example_db']
Note: Even if the database specified doesn’t exist, it won’t raise an error, it creates one as soon as you insert a document to one of it’s collections. In MongoDB, a collection is a grouping that contains documents. Collections can be thought of as tables and documents as rows in SQL database.
Insert a single document to MongoDB
books_collection = db.books data = { 'title': 'Pymongo Introduction', 'excerpt: 'pymongo is an official library for accessing and interacting with MongoDB.', 'author': 'Mongo' } result = books_collection.insert_one(data) print('One post: {0}'.format(result.inserted_id))
In order to insert a document to a collection in MongoDB database, we select the collection through same directive as we selected database. In the above code snippet we pass the JSON data to the insert_one() method. We can access the Object Identifier as an attribute to the response. An ObjectID is a unique identifier of a document in a collection. This OID is generated by Mongo.
Insert multiple documents to MongoDB
new_books = [ { 'title': 'Pymongo Introduction', 'excerpt: 'pymongo is an official library for accessing and interacting with MongoDB.', 'author': 'Mongo' }, { 'title': 'TheTaraNights', 'excerpt': 'good coders automate', 'author': 'Bhishan' }, { 'title': 'Automate the Boring Stuff with Python', 'excerpt': 'Anything and everything that is done manually can be automated', 'author': 'AI. Sweigart' }, ] result = books_collection.insert_many(new_books) print('Multiple posts: {0}'.format(result.inserted_ids))
In order to insert multiple documents to a collection, we can use the insert_many method which accepts a list of documents. Similarly we can access the list of Object Identifiers for the documents inserted via .inserted_ids attribute
Retrieving a document
bhishan_book = books_collection.find_one({'author': 'Bhishan'}) print(bhishan_book)
The above code snippet finds the first document that matches the criteria and returns it. If we want all the books by author ‘Bhishan’, we would use find() method instead of find_one().
bhishan_book = books_collection.find({'author': 'Bhishan'}) print(bhishan_book)
<pymongo.cursor.Cursor object at 0x107822f78>
The find() method returns a cursor object which can be iterated like a normal iterable in Python.
for each_book in bhishan_book: print(each_book)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK