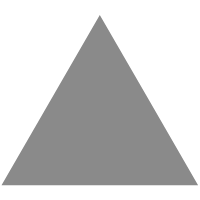
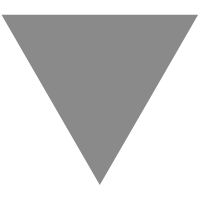
GitHub - diegohaz/styled-tools: Useful interpolated functions for CSS-in-JS
source link: https://github.com/diegohaz/styled-tools
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
styled-tools ?
Useful interpolated functions for styled-components ?, emotion, JSS and other CSS-in-JS libraries.
Install
$ npm install --save styled-tools
Usage
import styled, { css } from "styled-components"; import { prop, ifProp, switchProp } from "styled-tools"; const Button = styled.button` color: ${prop("color", "red")}; font-size: ${ifProp({ size: "large" }, "20px", "14px")}; background-color: ${switchProp("theme", { dark: "blue", darker: "mediumblue", darkest: "darkblue" })}; `; // renders with color: blue <Button color="blue" /> // renders with color: red <Button /> // renders with font-size: 20px <Button size="large" /> // renders with background-color: mediumblue <Button theme="darker" />
A more complex example:
const Button = styled.button` color: ${prop("theme.colors.white", "#fff")}; font-size: ${ifProp({ size: "large" }, prop("theme.sizes.lg", "20px"), prop("theme.sizes.md", "14px"))}; background-color: ${prop("theme.colors.black", "#000")}; ${switchProp("kind", { dark: css` background-color: ${prop("theme.colors.blue", "blue")}; border: 1px solid ${prop("theme.colors.blue", "blue")}; `, darker: css` background-color: ${prop("theme.colors.mediumblue", "mediumblue")}; border: 1px solid ${prop("theme.colors.mediumblue", "mediumblue")}; `, darkest: css` background-color: ${prop("theme.colors.darkblue", "darkblue")}; border: 1px solid ${prop("theme.colors.darkblue", "darkblue")}; ` })} ${ifProp("disabled", css` background-color: ${prop("theme.colors.gray", "#999")}; border-color: ${prop("theme.colors.gray", "#999")}; pointer-events: none; `)} `;
API
Table of Contents
prop
Returns the value of props[path]
or defaultValue
Parameters
path
stringdefaultValue
any
Examples
const Button = styled.button` color: ${prop("color", "red")}; `;
Returns any
ifProp
Returns pass
if prop is truthy. Otherwise returns fail
Parameters
Examples
// usage with styled-theme import styled from "styled-components"; import { ifProp } from "styled-tools"; import { palette } from "styled-theme"; const Button = styled.button` background-color: ${ifProp("transparent", "transparent", palette(0))}; color: ${ifProp(["transparent", "accent"], palette("secondary", 0))}; font-size: ${ifProp({ size: "large" }, "20px", ifProp({ size: "medium" }, "16px", "12px"))}; `;
Returns any
ifNotProp
Returns pass
if prop is falsy. Otherwise returns fail
Parameters
Examples
const Button = styled.button` font-size: ${ifNotProp("large", "20px", "30px")}; `;
Returns any
withProp
Calls a function passing properties values as arguments.
Parameters
Examples
// example with polished import styled from "styled-components"; import { darken } from "polished"; import { withProp, prop } from "styled-tools"; const Button = styled.button` border-color: ${withProp(prop("theme.primaryColor", "blue"), darken(0.5))}; font-size: ${withProp("theme.size", size => `${size + 1}px`)}; background: ${withProp(["foo", "bar"], (foo, bar) => `${foo}${bar}`)}; `;
Returns any
switchProp
Switches on a given prop. Returns the value or function for a given prop value.
Parameters
Examples
import styled, { css } from "styled-components"; import { switchProp, prop } from "styled-tools"; const Button = styled.button` font-size: ${switchProp(prop("size", "medium"), { small: prop("theme.sizes.sm", "12px"), medium: prop("theme.sizes.md", "16px"), large: prop("theme.sizes.lg", "20px") }}; ${switchProp("theme.kind", { light: css` color: LightBlue; `, dark: css` color: DarkBlue; ` })} `; <Button size="large" theme={{ kind: "light" }} />
Returns any
Types
Needle
License
MIT © Diego Haz
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK