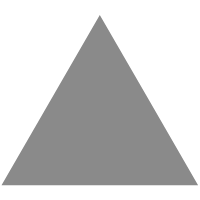
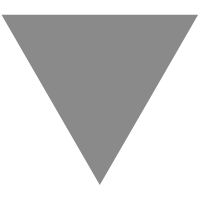
Developing With CUBA: A Big Shift From Spring? (Part 1)
source link: https://www.tuicool.com/articles/hit/nyYzAjY
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
When reading requirements for yet another web project for internal corporate use, you usually see a pretty common set of well-defined data storage structure (or sometimes it's an existing legacy DB), various forms for data entry, quite complex business logic, reporting and integrations with lots of existing corporate systems from accounting to supply management, thousands of concurrent users. What are your first thoughts?
"OK, I'll take a well-known RDBMS, Hibernate/JPA+Spring Boot, add REST API, and use my favorite/the latest JS framework to implement UI."
"Ah. And, I need to set up Spring Security and, maybe, add some custom code to protect data at the row level. How will I implement it? Probably through database views or virtual private database."
"And, all these DAOs are similar and boring, but I need to implement them."
"And, I need to use something like ModelMapper to convert JPA Entities to DTOs for REST."
"Don't forget to tell John — our new intern — about lazy fetching and JPA joins."
"Oh boy, can I get rid of all this routine stuff and focus on the critical piece of business logic implementation, instead of implementing yet another login form and Entity-to-DTO conversion?"
This article is for developers who have implemented at least a couple of projects from scratch using the Spring Framework (including Spring Boot) and are, now, thinking about boosting their productivity. In this article, I will show you how to get rid of very common, time-killing, routine tasks by using the CUBA platform.
Another Framework Again?
The number one question from developers when they hear about a new framework is: "Why do I need this when I can take Spring Boot and implement everything from scratch as I used to?" Well, fair enough — a new platform requires learning new principles and dealing with new limitations, leaving all years of your experience behind. Even if your current framework is not brilliant, you know it all; you know all the pitfalls and workarounds for them.
But, what if I tell you that CUBA does not require a U-turn (or even right angle turn) from the traditional Spring-way of development, but, instead, takes a slight step aside that allows you to eliminate boilerplate noise as hundreds of lines of DTOs, conversion utils, data pagination, and data filtering components are eliminated, creating configuration files for Spring Security (JPA, Cache — you name it).
We will start from the beginning and then show how CUBA application development follows the model that is used for almost all Spring-based applications and will let you use all your Spring kung-fu skills that you've learned in your developer's career to deliver more at the end. This post is focused on back-end code to make our story smaller and more concise.
Spring Application Architecture
The typical architecture of a Spring application can be googled easily and, in 90 percent of cases, it can be represented as a three-layered application with some cross-cutting areas. Let's have a look at a typical Spring application.
Domain Model— usually created manually, there are some tools for creating a domain model based on a datastore structure though.
Repository Layer— This includes classes that work with a data storage, also known as DAOs, repositories, etc. That's where all those ORM frameworks (and their siblings) rule. It usually contains classes that perform CRUD operations, using only one entity class from a domain model.
Service Layer— Sometimes, developers create an additional layer to separate business logic and data CRUD operations. This layer is useful if you have a complex business logic involving different types of data sources, external service integrations, etc.
Web/Controllers Layer(REST/MVC) —This set of classes deals with either a REST API (that will be consumed by browser-based applications) or views implemented using JSPs, template frameworks (thymeleaf, velocity), or JVM Frameworks (GWT, Vaadin, Wicket, etc.). Usually, controllers manipulate DTOs rather than entity objects, due to API structure or representation in views. Therefore, developers often have to implement bi-directional conversion between an entity model and a DTO model.
If all the above sounds familiar (and even like "Captain Obvious" to you), then that is a great sign that you are able to get started with CUBA.
Reference Application — Pet Clinic
They say, "words are cheap, show me your code."Spring has its own well-known "reference" application called the Pet Clinic, which can be found on GitHub . Below, we will show you how your Spring Developer's skills can be used when developing a backend for the new fork of the Pet Clinic — with CUBA now. There is a very good and detailed description of the reference application from Antoine Rey here ; we will discuss some of their main points below.
Data Model
The ER diagram of the database is shown in the diagram. The actual object domain model in the application's code is a bit more complex and includes some inheritance. You can find the UML in the presentation mentioned above.
Repository Level
There are four repositories to deal with main entities: owner, pet, visit, and vet. Those repositories are based on the Spring JPA Framework and contain almost no code, thanks to Spring JPA, but you can find a custom query in Owner repository to fetch owners and their pets in one request.
UI Screens
The application consists of nine screens that allow us to view all data and, even, edit some of it, including pet owners, pets, and visits. We won't talk about them now, but I need to mention that those screens are just simple CRUD forms that are pretty common for the most data-oriented applications.
Additional Features
Apart from simple CRUD functionality, the application provides some not-so-obvious functionality that shows the power of the Spring Framework:
- Caching — The vet's list is cached, so there are no queries to DB when the vet list is refreshed.
- Validator — This checks to see if all fields are filled during the creation of a new record about a pet.
- Formatter — This is used for proper display of a pet type.
- i18n - This application is available in English and German languages.
- Transaction management — Some DB queries are made read-only.
A Side Note
I like this picture a lot, it reflects my feelings about using libraries and frameworks. To use any framework efficiently, you need to understand how it works inside. For example, Spring Boot hides a lot of things from you, and you will be surprised at how many classes lay behind one simple JPA interface initialization. Here are some notes about the "magic" happening in the Spring Boot Pet Clinic application:
- There is no cache configuration code apart from the @Caсheable annotation, but, somehow, Spring Boot "knows" how to set up a cache implementation (EhCache in our case).
- Repositories are not marked as
@Transactional
(neither is their parent classorg.springframework.data.repository.Repository
), but allsave()
methods work just fine there.
But, despite all this, Spring Boot is a very popular framework, because it's transparent and predictable. It has a very detailed documentation, and it's open source, so you can read how things work and drill down into any method and see what's going on there. I guess everyone likes transparent and manageable frameworks — in fact, using them makes your application extremely maintainable.
Check out our next installment where we will take a look at how to go about using the PetClinic application implemented with the CUBA Framework. Stay tuned!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK