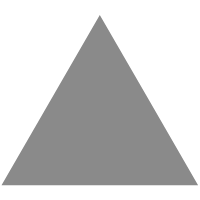
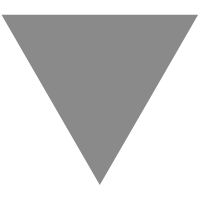
Java迭代器升级版探究
source link: https://www.linuxidc.com/Linux/2018-07/153127.htm?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Alei最近和迭代器较上了劲,之前自以为深究过迭代器,不成想原来是坐井观天,以蠡测海。上文中写的东西哪里算什么深入探究?!但亡羊补牢,犹未迟也,经我多次试验,终于弄懂其中某些精巧机制,闲话少说,我们进入正题。
注意,之后所有的知识点都以 ArrayList 这个容器类为例来进行详细说明
在讨论这个问题之前我们得首先在意两个成员变量:
1、ArrayList 类里继承于 AbstractList 类的成员变量 modCount:
protected transient int modCount = 0;
2、ArrayList 类的私有内部类 Itr 里的成员变量 expectedModCount:
int expectedModCount = modCount;
再看下Itr类源码:
private class Itr implements Iterator<E> {
int cursor; // index of next element to return
int lastRet = -1; // index of last element returned; -1 if no such
int expectedModCount = modCount;
public boolean hasNext() {
return cursor != size;
}
@SuppressWarnings("unchecked")
public E next() {
checkForComodification();
int i = cursor;
if (i >= size)
throw new NoSuchElementException();
Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length)
throw new ConcurrentModificationException();
cursor = i + 1;
return (E) elementData[lastRet = i];
}
public void remove() {
if (lastRet < 0)
throw new IllegalStateException();
checkForComodification();
try {
ArrayList.this.remove(lastRet);
cursor = lastRet;
lastRet = -1;
expectedModCount = modCount;
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
}
@Override
@SuppressWarnings("unchecked")
public void forEachRemaining(Consumer<? super E> consumer) {
Objects.requireNonNull(consumer);
final int size = ArrayList.this.size;
int i = cursor;
if (i >= size) {
return;
}
final Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length) {
throw new ConcurrentModificationException();
}
while (i != size && modCount == expectedModCount) {
consumer.accept((E) elementData[i++]);
}
// update once at end of iteration to reduce heap write traffic
cursor = i;
lastRet = i - 1;
checkForComodification();
}
final void checkForComodification() {
if (modCount != expectedModCount)
throw new ConcurrentModificationException();
}
}
当我们使用 ArrayList 容器的 iterator() 方法后,在栈空间里创建了一个此类特定的迭代器对象,同时将成员变量 modCount 的值赋予成员变量 expectedModCount。知道这个有趣的事情后可以先打住,让我们再来看看 ArrayList 类 remove() 方法的源码:
参数为 int 类型的 remove():
public E remove(int index) {
rangeCheck(index);
modCount++;
E oldValue = elementData(index);
int numMoved = size - index - 1;
if (numMoved > 0)
System.arraycopy(elementData, index+1, elementData, index,
numMoved);
elementData[--size] = null; // clear to let GC do its work
return oldValue;
}
参数为 Object 类型的 remove():
public boolean remove(Object o) {
if (o == null) {
for (int index = 0; index < size; index++)
if (elementData[index] == null) {
fastRemove(index);
return true;
}
} else {
for (int index = 0; index < size; index++)
if (o.equals(elementData[index])) {
fastRemove(index);
return true;
}
}
return false;
}
/*
* Private remove method that skips bounds checking and does not
* return the value removed.
*/
private void fastRemove(int index) {
modCount++;
int numMoved = size - index - 1;
if (numMoved > 0)
System.arraycopy(elementData, index+1, elementData, index,
numMoved);
elementData[--size] = null; // clear to let GC do its work
}
以 ArrayList 类里 remove() 方法为例来看,只要我们调用此方法一次,那么 modCount 便自加一次 1。于是我们可以理解,modCount 是一个记录容器对象修改次数的变量,它是一个计数器。小伙伴门完全可以去查源码,不仅仅是 remove(),凡是涉及对 ArrayList 对象的增、删、改的任何一种方法,当我们调用一次这类方法,那 modCount 便会自加一次 1,即,记录一次容器对象的改变。例如,当我创建一个 ArrayList 对象 al 后,我调用 al.add() 一次,调用 al.remove() 一次,再调用 al.add() 一次后,那么 modCount = 3,由此说明 al 被修改了3次。
在没有创建迭代器对象之前的任何对容器对象的增删改操作只会让 modCount 自加,当我们创建一个对应容器类的迭代器对象之后,int expectedModCount = modCount,迭代器对象里的 expectedModCount 成员变量被初始化为与 modCount 里的数值一样的值。
有了迭代器,然后用迭代器进行迭代,就涉及到迭代器对象的 hasNext();next()方法了,我们看下这两个方法的源码:
public boolean hasNext() {
return cursor != size;
}
@SuppressWarnings("unchecked")
public E next() {
checkForComodification();
int i = cursor;
if (i >= size)
throw new NoSuchElementException();
Object[] elementData = ArrayList.this.elementData;
if (i >= elementData.length)
throw new ConcurrentModificationException();
cursor = i + 1;
return (E) elementData[lastRet = i];
}
由此可见,两个方法都不会改变 expectedModCount 的值,那怎么理解 expectedModCount 这个成员变量呢?再看迭代器里的 remove() 方法源码:
public void remove() {
if (lastRet < 0)
throw new IllegalStateException();
checkForComodification();
try {
ArrayList.this.remove(lastRet);
cursor = lastRet;
lastRet = -1;
expectedModCount = modCount;
} catch (IndexOutOfBoundsException ex) {
throw new ConcurrentModificationException();
}
}
在 remove() 方法的方法体里,有“expectedModCount = modCount; “这样一行语句,那么不管我们调用多少次迭代器的 remove() 方法,始终会让 expectedModCount 的数值等于 modCount 的值,这里的 expectedModCount 可理解为使用迭代器对容器类对象进行修改的”期望修改次数“,就是说:迭代器里的这个”期望修改次数“一定要和已经记录下的容器的修改次数 modCount 一样,那么当你通过迭代器对容器类对象遍历并进行修改时,使用迭代器本身的 remove() 才有意义(即让 expectedModCount = modCount)!!而在 next() 方法体里首行的 checkForComodification() 方法是这样定义的:
final void checkForComodification() {
if (modCount != expectedModCount)
throw new ConcurrentModificationException();
}
看了源码,我们应该知道: checkForComodification() 的作用是检查 expectedModCount 和 modCount 的值是否相等,如果不等,则抛出 ConcurrentModificationException 这个异常。这下显而易见了,在我们通过迭代器进行遍历时,若使用非迭代器对象提供的修改容器类对象的任何方法,则 modCount 的值增大,而 expectedModCount 地值不发生改变,那么在进入下一次循环时,next() 方法体里首行的 checkForComodification() 方法检查到 expectedModCount 与 modCount 不等后抛出了 ConcurrentModificationException。
那么,在通过迭代器进行迭代时,容器对象里的任何元素都不能通过容器类所提供的方法进行增删改的操作么?非也非也,Alei留下这样一段代码:
public static void main(String[] args) {
Collection c = new ArrayList();
c.add(new String("aaa"));
c.add(new String("bbb"));
c.add(new String("ccc"));
c.add(new String("ddd"));
c.add(new String("fff"));
c.add(new String("eee"));
for (Object o : c) {
// System.out.print(o + " ");
if (o.equals("fff")) {
c.remove(o);
}
}
System.out.println(c);
}
当我们运行这段程序,你将会发现 ”fff“ 这个字符串对象怎么被成功删除了?!这也是我之前一直疑惑且略显白痴的地方。其实,我可以下定结论:在通过迭代器进行迭代时,容器对象里的倒数第二个元素一定可以过容器类所提供的 remove() 方法进行删除操作(不管这个容器的 size 有多大)。这又是为什么呢?哈哈,对于这个问题,留待小伙伴们自行解决吧^_^!
Linux公社的RSS地址: https://www.linuxidc.com/rssFeed.aspx
本文永久更新链接地址: https://www.linuxidc.com/Linux/2018-07/153127.htm
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK