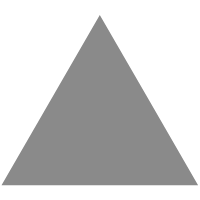
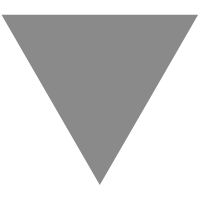
Blazor Application Bootstrap and Lifecycle Methods
source link: https://www.tuicool.com/articles/hit/jUNzAzi
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
We have seen in my last article how web assembly and C# can be a game changer and will help the C# to run on Browser so moving to the next article, let’s see how we can setup Blazor in our machine and how we can get started with our first application with Blazor.
Agenda
- Environment setup.
- Application Bootstrap Process.
- Application Life Cycle Methods.
- Conclusion.
1. Environment Setup
While writing this article, the new experimental Blazor framework 0.0.4 was announced by Microsoft. To get this version there are few prerequisites, defined below:
-
Install .NET Core SDK 2.1 from here.
-
Install VS 2017 Preview (15.7) with Web Workbench selected during installation.
-
The most important step is to install the language service extension for Blazor from here .
To verify the installation, open Visual Studio and create one ASP.NET Core web application. We should now be able to see following templates:
2. Getting Started and Building Our First Blazor App
We have done the setup part, now it’s time to create our first demo app with Blazor.
1. Create a new project with the ASP.NET Core web application selected. Name it BlazorDemoApp1 and click OK.
2. The next step is to select the Environment and use the proper Template to do so.
2.1. We need to make sure that .NET Core is selected. ASP.NET Core 2.0 or 2.1 should also be selected.
2.2 The next step is to select the template for the Blazor application for our demo. We will select the template called Blazor and press OK.
3. Application Bootstrap
Where does our application bootstrap? Obviously, it is the main method in the program.cs file. To see how it looks, lets look at the code snippet below:
public class Program { static void Main(string[] args) { var serviceProvider = new BrowserServiceProvider(services => { // Add any custom services here }); new BrowserRenderer(serviceProvider).AddComponent<App>("app"); } }
This is the place where we decide which component will be loaded. This DOM element selector argument will decide whether we want to load the root component or not; in our case, the app element in the index.html file will be used for the rendering.
The main method can be used to add the various services which will be used for the DI in later parts of the app.
When we see the index.html snippet below:
<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <meta name="viewport" content="-width"> <title>WebApplication2</title> <base href="/" /> <link href="css/bootstrap/bootstrap.min.css" rel="stylesheet" /> <link href="css/site.css" rel="stylesheet" /> </head> <body> <app>Loading...</app> <!--Root Component Loads here--> <script type="blazor-boot"></script> </body> </html>
Here, blazor-boot
is used as the script type. Now the question is why was it is used here in the index.html file?
When we build the application, blazor-boot
is replaced with the bootstrapping script which handles the .NET run time and executes the entry point of the application. The following screenshot can tell us what is loaded where we use blazor-boot
.
Here we can see all the things needed to run the .NET run time are loaded with this and our app gets bootstrapped.
Application Lifecycle methods
All right, we have set up our project, let's dig out the details of the application lifecycle method of the Blazor App. There are around 7 lifecycle methods available in the Blazor app; Blazor provides synchronous as well as asynchronous lifecycle methods, let's, one-by-one, how they can be used.
1. OnInit()
This is the synchronous version of the application method which gets executed when the component gets initialized. This gets executed when the component is completely loaded. We can use this method to load data from services, as after this method each control in the UI is loaded. This is executed when the component is ready and when it has received the values from the parent in the render tree
2. OnInitAsync()
This is the asynchronous version of the application method which gets executed when the component is initialized. This is called when the component is completely initialized and can be used to call the data service or to load the data from the service. This is executed when the component is ready and when it has received the values from the parent in the render tree.
3. OnParametersSet()
This is the synchronous way of setting the parameter when the component receives the parameter from its parent component. This is called when the initialization of the component occurs.
4. OnParametersSetAsync()
This is an asynchronous way of setting the parameter when the component received the parameter from the parent component, this gets called when the initialization of the component occurs.
5. ShouldRender()
We use this method to suppress the refreshing of the UI. If this method returns true then UI is refreshed, otherwise, changes are not sent to UI. One thing about ShouldRender()
is that it always does the initial rendering despite its return value.
6. OnAfterRender()
This is called each time the component finishes rendering all the references to the component and they all are populated. We can make use of this method to perform additional steps like initializing the other components.
6. OnAfterRenderAsync()
This method is an asynchronous version of the OnAfterRender()
which gets called when the rendering of all the references to the component are populated. We can use this method to perform additional initializing of third party components.
Here's some code demonstrating the application lifecycle:
@page "/" <h1>Application Life cycle Methods ..</h1> @foreach (var item in EventType){ @item <hr />} @functions{ List<string> EventType = new List<string>(); protected override void OnInit() { EventType.Add(" 1 OnInit"); } protected override async Task OnInitAsync() { EventType.Add("2 OnInit Async"); await Task.Delay(1000); } protected override void OnParametersSet() { EventType.Add("3 On Parameter set "); } protected override async Task OnParametersSetAsync() { EventType.Add(" 4 OnParametersSet Async Started"); await Task.Delay(1000); } protected override bool ShouldRender() { EventType.Add(" 5 Should render called"); return true; } protected override void OnAfterRender() { EventType.Add(" 6 OnAfterRenderStarted"); } protected override async Task OnAfterRenderAsync() { EventType.Add(" 7 OnAfterRender Async Started"); await Task.Delay(1000); } }
Running the above code we will get the following output:
We can see the steps and way it is been called one by one and the sequence of the application methods.
Conclusion
Blazor is the technology which uses web assembly for running the application. It uses ASP.NET Core to build the application. It has many similarities with the current UI framework languages like React or Angular and, being a C# developer, it will be a great platform to build the applications on, especially single page applications. Although not available for production systems, this certainly is an exciting time.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK