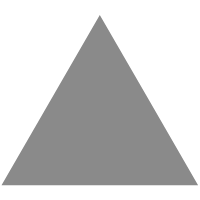
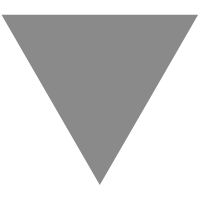
GitHub - callstack/linaria: Zero-runtime CSS in JS library
source link: https://github.com/callstack/linaria
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
README.md
Zero-runtime CSS in JS library.
Features
- Familiar CSS syntax with Sass like nesting.
- CSS is extracted at build time, no runtime is included.
- JavaScript expressions are supported and evaluated at build time.
- Critical CSS can be extracted for inlining during SSR.
- Integrates with existing tools like Webpack to provide features such as Hot Reload.
Installation
Install it like a regular npm package:
yarn add linaria
We recommend to use linaria/loader
if you use Webpack:
module: { rules: [ { test: /\.js$/, use: ['babel-loader', 'linaria/loader'], }, ], },
If you don't use Webpack, you can add the linaria/babel
preset to your Babel configuration:
{ "presets": [ "env", "react", ["linaria/babel", { "single": true, "filename": "styles.css", "outDir": "dist" }] ] }
Documentation
- API and usage
- Configuring Babel
- Dynamic Styles
- Theming
- Server Rendering
- Bundlers integration
- Linting
- Example
How it works
Linaria lets you write CSS code in a tagged template literal in your JavaScript files. The Babel plugin extracts the CSS rules to real CSS files, and generates unique class names to use.
Example is worth a thousand words:
import React from 'react'; import { css, include, styles } from 'linaria'; import { modularScale, hiDPI } from 'polished'; import fonts from './fonts'; import colors from './colors'; const title = css` text-transform: uppercase; `; const container = css` padding: 3em; `; const header = css` ${include(title)}; font-family: ${fonts.heading}; font-size: ${modularScale(2)}; ${hiDPI(1.5)} { font-size: ${modularScale(2.5)} } `; export default function Header({ className }) { return ( <div {...styles(container, className)}> <h1 {...styles(header)} /> </div> ); } export function Block() { return <div {...styles(container)} />; } export function App() { return <Header {...styles(title)} />; }
After being transpiled, the code will output following CSS:
.title__jt5ry4 { text-transform: uppercase; } .container__jdh5rtz { padding: 3em; } .header__xy4ertz { text-transform: uppercase; font-family: Helvetica, sans-serif; /* constants are automatically inlined */ font-size: 2.66em; } @media only screen and (min-resolution: 144dpi), only screen and (min-resolution: 1.5dppx) { .header__xy4ertz { font-size: 3.3325em; } }
And the following JavaScipt:
import React from 'react'; import { styles } from 'linaria/build/index.runtime'; const title = 'title__jt5ry4'; const container = 'container__jdh5rtz'; const header = 'header__xy4ertz'; export default function Header({ className }) { return ( <div {...styles(container, className)}> <h1 {...styles(header)} /> </div> ); } export function App() { return <Header {...styles(title)} />; }
Trade-offs
-
Dynamic styles are not supported with
css
tag. See Dynamic Styles for alternative approaches. -
Modules used in the CSS rules cannot have side-effects. For example:
import { css } from 'linaria'; import colors from './colors'; const title = css` color: ${colors.text}; `;
Here, there should be no side-effects in the
colors.js
file, or any file it imports. We recommend to move helpers and shared configuration to files without any side-effects.
Editor Plugins
CSS Autocompletion
- VSCode, Atom, SublimeText – typescript-styled-plugin
VSCode
- Syntax Highlighting - Styled Components Plugin
Atom
- Syntax Highlighting - Babel Grammar
Recommended Libraries
- linaria-jest – Jest testing utilities for Linaria.
- babel-plugin-object-styles-to-template - Babel plugin to write styles in object syntax with linaria
- polished.js - A lightweight toolset for writing styles in JavaScript.
Inspiration
Acknowledgements
This project wouldn't have been possible without the following libraries or the people behind them.
Special thanks to @kentcdodds for his babel plugin and @threepointone for his suggestions and encouragement.
Contributors
Thanks goes to these wonderful people (emoji key):
Paweł Trysła
? ? ?
Satyajit Sahoo
? ?
Michał Pierzchała
? ? ?
Lucas
?
Alexey Pronevich
?
Wojtek Szafraniec
?
Tushar Sonawane
? ?
Ferran Negre
?
Jakub Beneš
? ?
Oscar Busk
? ?
Dawid
? ?
This project follows the all-contributors specification. Contributions of any kind welcome!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK