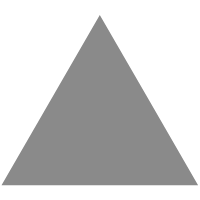
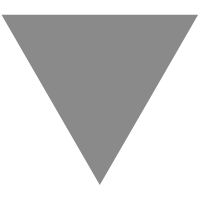
深入Spring Boot:快速集成Dubbo + Hystrix
source link: http://www.importnew.com/29019.html?amp%3Butm_medium=referral
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
背景
Hystrix 旨在通过控制那些访问远程系统、服务和第三方库的节点,从而对延迟和故障提供更强大的容错能力。Hystrix具备拥有回退机制和断路器功能的线程和信号隔离,请求缓存和请求打包,以及监控和配置等功能。
Dubbo是Alibaba开源的,目前国内最流行的java rpc框架。
本文介绍在spring应用里,怎么把Dubbo和Hystrix结合起来使用。
Spring Boot应用
生成dubbo集成spring boot的应用
对于不熟悉dubbo 集成spring boot应用的同学,可以在这里直接生成dubbo + spring boot的工程: http://start.dubbo.io/
配置spring-cloud-starter-netflix-hystrix
spring boot官方提供了对hystrix的集成,直接在pom.xml里加入依赖:
<dependency> <groupId>org.springframework.cloud</groupId> <artifactId>spring-cloud-starter-netflix-hystrix</artifactId> <version>1.4.4.RELEASE</version> </dependency>
然后在Application类上增加 @EnableHystrix
来启用hystrix starter:
@SpringBootApplication @EnableHystrix public class ProviderApplication {
配置Provider端
在Dubbo的Provider上增加 @HystrixCommand
配置,这样子调用就会经过Hystrix代理。
@Service(version = "1.0.0") public class HelloServiceImpl implements HelloService { @HystrixCommand(commandProperties = { @HystrixProperty(name = "circuitBreaker.requestVolumeThreshold", value = "10"), @HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "2000") }) @Override public String sayHello(String name) { // System.out.println("async provider received: " + name); // return "annotation: hello, " + name; throw new RuntimeException("Exception to show hystrix enabled."); } }
配置Consumer端
对于Consumer端,则可以增加一层method调用,并在method上配置 @HystrixCommand
。当调用出错时,会走到 fallbackMethod = "reliable"
的调用里。
@Reference(version = "1.0.0") private HelloService demoService; @HystrixCommand(fallbackMethod = "reliable") public String doSayHello(String name) { return demoService.sayHello(name); } public String reliable(String name) { return "hystrix fallback value"; }
通过上面的配置,很简单地就完成了Spring Boot里Dubbo + Hystrix的集成。
传统Spring Annotation应用
传统spring annotation应用的配置其实也很简单,和spring boot应用不同的是:
-
显式配置Spring AOP支持:
@EnableAspectJAutoProxy
-
显式通过
@Configuration
配置HystrixCommandAspect
Bean。
@Configuration @EnableDubbo(scanBasePackages = "com.alibaba.dubbo.samples.annotation.action") @PropertySource("classpath:/spring/dubbo-consumer.properties") @ComponentScan(value = {"com.alibaba.dubbo.samples.annotation.action"}) @EnableAspectJAutoProxy static public class ConsumerConfiguration { @Bean public HystrixCommandAspect hystrixCommandAspect() { return new HystrixCommandAspect(); } }
Hystrix集成Spring AOP原理
在上面的例子里可以看到,Hystrix对Spring的集成是通过Spring AOP来实现的。下面简单分析下实现。
@Aspect public class HystrixCommandAspect { @Pointcut("@annotation(com.netflix.hystrix.contrib.javanica.annotation.HystrixCommand)") public void hystrixCommandAnnotationPointcut() { } @Pointcut("@annotation(com.netflix.hystrix.contrib.javanica.annotation.HystrixCollapser)") public void hystrixCollapserAnnotationPointcut() { } @Around("hystrixCommandAnnotationPointcut() || hystrixCollapserAnnotationPointcut()") public Object methodsAnnotatedWithHystrixCommand(final ProceedingJoinPoint joinPoint) throws Throwable { Method method = getMethodFromTarget(joinPoint); Validate.notNull(method, "failed to get method from joinPoint: %s", joinPoint); if (method.isAnnotationPresent(HystrixCommand.class) && method.isAnnotationPresent(HystrixCollapser.class)) { throw new IllegalStateException("method cannot be annotated with HystrixCommand and HystrixCollapser " + "annotations at the same time"); } MetaHolderFactory metaHolderFactory = META_HOLDER_FACTORY_MAP.get(HystrixPointcutType.of(method)); MetaHolder metaHolder = metaHolderFactory.create(joinPoint); HystrixInvokable invokable = HystrixCommandFactory.getInstance().create(metaHolder); ExecutionType executionType = metaHolder.isCollapserAnnotationPresent() ? metaHolder.getCollapserExecutionType() : metaHolder.getExecutionType(); Object result; try { if (!metaHolder.isObservable()) { result = CommandExecutor.execute(invokable, executionType, metaHolder); } else { result = executeObservable(invokable, executionType, metaHolder); } } catch (HystrixBadRequestException e) { throw e.getCause() != null ? e.getCause() : e; } catch (HystrixRuntimeException e) { throw hystrixRuntimeExceptionToThrowable(metaHolder, e); } return result; }
-
HystrixCommandAspect
里定义了两个注解的AspectJ Pointcut:@HystrixCommand
,@HystrixCollapser
。所有带这两个注解的spring bean都会经过AOP处理 -
在
@Around
AOP处理函数里,可以看到Hystrix会创建出HystrixInvokable
,再通过CommandExecutor
来执行
spring-cloud-starter-netflix-hystrix的代码分析
-
@EnableHystrix
引入了@EnableCircuitBreaker
,@EnableCircuitBreaker
引入了EnableCircuitBreakerImportSelector
@EnableCircuitBreaker public @interface EnableHystrix { } @Import(EnableCircuitBreakerImportSelector.class) public @interface EnableCircuitBreaker { }
-
EnableCircuitBreakerImportSelector
继承了SpringFactoryImportSelector<EnableCircuitBreaker>
,使spring加载META-INF/spring.factories
里的EnableCircuitBreaker
声明的配置在META-INF/spring.factories
里可以找到下面的配置,也就是引入了HystrixCircuitBreakerConfiguration
。org.springframework.cloud.client.circuitbreaker.EnableCircuitBreaker=\ org.springframework.cloud.netflix.hystrix.HystrixCircuitBreakerConfiguration
-
在
HystrixCircuitBreakerConfiguration
里可以发现创建了HystrixCommandAspect
@Configuration public class HystrixCircuitBreakerConfiguration { @Bean public HystrixCommandAspect hystrixCommandAspect() { return new HystrixCommandAspect(); }
可见 spring-cloud-starter-netflix-hystrix
实际上也是创建了 HystrixCommandAspect
来集成Hystrix。
另外 spring-cloud-starter-netflix-hystrix
里还有metrics, health, dashboard等集成。
-
对于dubbo provider的
@Service
是一个spring bean,直接在上面配置@HystrixCommand
即可 -
对于dubbo consumer的
@Reference
,可以通过加一层简单的spring method包装,配置@HystrixCommand
即可 -
Hystrix本身提供
HystrixCommandAspect
来集成Spring AOP,配置了@HystrixCommand
和@HystrixCollapser
的spring method都会被Hystrix处理
Recommend
-
59
废话不多说,今天说说Spring Boot和Dubbo的整合,注册服务中心用的是Zookeeper,至于Dubbo、Zookeeper为何物我在此不再多言,不知道是什么自己去百度,本文适用于对微服务或者RPC了解的人准备的! 先介绍一下整合过程中用到...
-
39
dubbo-spring-boot-starter English Dubbo Spring Boot Starter。也可以关注dubbo官方的
-
81
(点击上方公众号,可快速关注) 来源:hengyunabc , blog.csdn.net/hengyuna...
-
42
一、前言 一个现实的场景是:当我们开发一个Web工程时,架构师和开发工程师可能更关心项目技术结构上的设计。而几乎所有结构良好的软件(项目)都使用了分层设计。分层设计是将项目按技术职能分为几个内聚的部分,从而将技术或接...
-
0
Here’s the table of contents: 依赖包安装 全局安装package.json中的依赖项 devDependencies 在项目目录中安装,其中,–save-dev是本地安装 npm install webpac...
-
1
使用Testcontainer对Spring Boot实现集成测试 在使用容...
-
1
Spring Boot 3.0 (二十):Spring Boot 集成 Memcached 2023/01/20...
-
0
在日常的后端开发中,使用mybatis作为DAO层的持久框架已经是惯例。但很多时候都是在别人搭好的框架中进行开发,对怎么搭建环境是一知半解,今天就来实践下。 一、集成分哪些步骤 来看下集成mybatis需要哪些步骤, 1、确定环境及依赖 2...
-
19
Dubbo应用迁移到docker的问题 Dubbo是阿里开源的一套服务治理与rpc框架,服务的提供者通过zookeeper把自己的服务发布上去,然后服务调用方通过zk获取服务的ip和端口,dubbo客户端通过自己的软负载功能自动选择服务提...
-
4
实例讲解SpringBoot集成Dubbo的步骤及过程 作者:架构师老卢 2023-10-18 07:36:56 Spring Boot 是一个开源的 Java Web 框架,它可以帮助开发者快速创建独立的、生产级别的 Spring 应用程序。Spring Boot 提供了很...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK