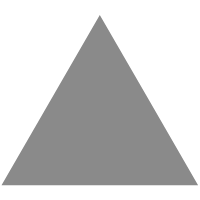
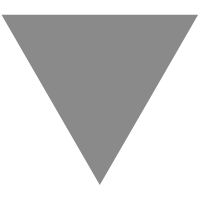
Let's create our map method
source link: https://www.tuicool.com/articles/hit/7n2yyyz
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
If you want to take a closer look on how JS works and how we created our own filter function, you can see my earlier post:
I think this two posts combined are a good way to find out how standard array methods work in JS.
It's very important to know that Array.prototype.map() returns a new array which means that the old array is not mutated. (Mutation is a very important concept when you start to work with React and Redux libraries). Enough talking, now let's code. Here is a simple example how map works.
var arr = [1,2,3]; var newArr = arr.map(function(element){ return element + 1; }); console.log('arr: ', arr); // arr is not mutated and returns [1,2,3] console.log('newArr: ', newArr ); //newArr returns [2,3,4]
Now, Let's go code our own map function
In this example we will not check if the first parameter is an array or if the second parameter is a callback function, and so on. Detailed syntax of map function you can find on developer.mozilla.org.
let arr = [1,2,3]; Array.prototype.ownMap = function(callback) { let mappedArr = []; for(let i=0; i < this.length; i++) { mappedArr.push(callback(this[i])) } return mappedArr; } let newArr = arr.ownMap(function(element) { return element + 1; }); console.log('arr: ', arr); // not mutated and returns [1, 2, 3] console.log('newArr: ', newArr); // returns [2, 3, 4]
Now let's play with indexes because an index can be send as the second parameter in map callback function.
let arr = [1,2,3,4]; Array.prototype.ownMap = function(callback) { let mappedArr = []; for(let i=0; i < this.length; i++) { mappedArr.push(callback(this[i], i)) // we defined i as second argument } return mappedArr; } let newArr = arr.ownMap(function(element, index) { return element + index; }); console.log('arr: ', arr); // not mutated and returns [1, 2, 3, 4] console.log('newArr: ', newArr); // returns [1, 3, 5, 7]
Conclusion
When you are learning something it's always best to know how things work under the hood. Please, let me know, if there is something I didn't mention and it's good to know.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK