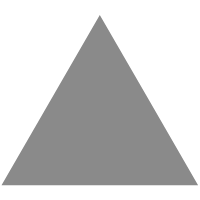
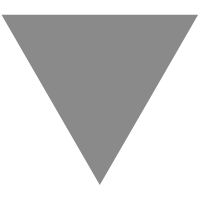
GitHub - raphamorim/react-tv: [WIP] React development for TV (Renderer for low m...
source link: https://github.com/raphamorim/react-tv
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
React-TV ·
react-tv: React Renderer for low memory applications.
react-tv-cli: React Packager for TVs.
Currently under development.
import React from 'react'
import ReactTV, { Platform } from 'react-tv'
class Clock extends React.Component {
state = { date: new Date() }
componentDidMount() {
setInterval(() => this.setState({date: new Date()}), 1000)
}
render() {
if (Platform('webos')) {
return (
<h1>Time is {this.state.date.toLocaleTimeString()}</h1>
)
}
return <h2>This App is available only at LG webOS</h2>
}
}
ReactTV.render(<Clock/>, document.getElementById('root'))
Summary
About React-TV
React-TV is an ecosystem for TV based React applications (from the renderer to CLI for pack/build applications).
At the moment we're focusing on webOS and SmartTV.
React-TV's aims to be a better tool for building and developing fast for TVs.
Understanding the Problem
tl;dr: Crafting a high-performance TV user interface using React
Crafting a high-performance TV user interface using React is a real challenge, because of some reasons:
- Limited graphics acceleration
- Single core CPUs
- High Memory Usage for a common TV App
These restrictions make super responsive 60fps experiences especially tricky. The strategy is step in the renderer: Applying reactive concepts to unblock the processing on the renderer layer, plug the TV's keyListener, avoid React.createElement.
In addition: Unify the build for multiple TV platforms.
Articles
Friendly list of tutorials and articles:
react-tv-cli
To install react-tv-cli
(CLI Packager):
$ yarn global add react-tv-cli
Support for React-TV-CLI
Target Platform | Status | Available Version |
---|---|---|
LG webOS |
stable | 0.3.1 |
Samsung Tizen |
ongoing | x |
Samsung Orsay |
not started yet | x |
Sony PS4 |
not started yet | x |
Nintendo Switch |
not started yet | x |
Developing for webOS
Short Description: webOS, also known as Open webOS or LG webOS, (previously known as HP webOS and Palm webOS, stylized as webOS) is a Linux kernel-based multitasking operating system for smart devices such as Smart TVs and it has been used as a mobile operating system.
First of all, setup your webOS Environment:
Setup webOS Enviroment
Then, init your react-tv project:
$ react-tv-cli init <my-app-name>
From the project directory, install the dependencies to enable building:
$ yarn install
You will need to keep the list of files related to your app on the React-TV entry up to date in package.json
. The init command will already add index.html
, bundle.js
and style.css
to the package.
{
"name": "my-app-name",
"react-tv": {
"files": [
"index.html",
"bundle.js",
"style.css"
]
}
}
To build your project:
$ yarn build
Once the project is built, you can run it on a specific device or emulator:
$ react-tv-cli run-webos <device>
- When you not specify the device, it runs on VirtualBox webOS Simulator
react-tv
To install react-tv
(React Renderer):
$ yarn add react-tv
Platform
When building a cross-platform TV app, you'll want to re-use as much code as possible. You'll probably have different scenarios where different code might be necessary.
For instance, you may want to implement separated visual components for LG-webOS
and Samsung-Tizen
.
React-TV provides the Platform
module to easily organize your code and separate it by platform:
import { Platform } from 'react-tv'
console.log(Platform('webos')) // true
console.log(Platform('tizen')) // false
console.log(Platform('orsay')) // false
renderOnAppLoaded
Takes a component and returns a higher-order component version of that component, which renders only after application was launched, allows to not write diffent logics for many devices.
import { renderOnAppLoaded } from 'react-tv'
const Component = () => (<div></div>)
const App = renderOnAppLoaded(Component)
findDOMNode
Similar to react-dom findDOMNode
Navigation
If you want to start with Navigation for TVs. React-TV provides a package for spatial navigation with declarative support based on Netflix navigation system.
React-TV Navigation exports withFocusable
and withNavigation
which act as helpers for Navigation.
import React from 'react'
import ReactTV from 'react-tv'
import { withFocusable, withNavigation } from 'react-tv-navigation'
const Item = ({focused, setFocus, focusPath}) => {
focused = (focused) ? 'focused' : 'unfocused'
return (
<div onClick={() => { setFocus() }} >
It's {focused} Item
</div>
)
}
const Button = ({setFocus}) => {
return (
<div onClick={() => { setFocus('item-1') }}>
Back To First Item!
</div>
)
}
const FocusableItem = withFocusable(Item)
const FocusableButton = withFocusable(Button)
function App({currentFocusPath}) {
return (
<div>
<h1>Current FocusPath: '{currentFocusPath}'</h1>,
<FocusableItem focusPath='item-1'/>
<FocusableItem focusPath='item-2'/>
<FocusableButton
focusPath='button'
onEnterPress={() => console.log('Pressed enter on Button!')}/>
</div>
)
}
const NavigableApp = withNavigation(App)
ReactTV.render(<NavigableApp/>, document.querySelector('#app'))
See examples/navigation for more details about usage.
Examples
Clock App
Youtube App
References:
webOS
Videos
Windows
Essentials to beginner
Developing for SmartTV Guidelines
React Basics and Renderer Architecture
Roadmap
Stage 1
Initial proof-of-concept. [DONE]
- CLI Build Abstraction of LG webOS (
run-webos
,run-webos-dev
) - Create a guide or script to Install all LG webOS environment
- Renderer ReactElements to simple DOM
- Support HOF and HOC
- Support State and Lifecycle
- Keyboard Navigation
- Check
webos
Platform - Migrate to
React-Reconciler
Stage 2 [IN PROGRESS]
Implement essential functionality needed for daily use by early adopters.
- Support render to Canvas instead DOM using
React.CanvasComponent
-
run-webos
support TV device as param - Optmizate DOMRenderer for TV
- Start CLI for Tizen
- Develop helpers for webOS debbug (e.g: Log System).
- Support Cross Platform
- Check executable bin path for Windows, OSX and Linux
- Bind all TV key listeners on
React.Element
- Improve documentation
- Benchmark it
Stage 3
Add additional features users expect from a Renderer. Then fix bugs and stabilize through continuous daily use. At this point we can start to experiment with innovative ideas and paradigms.
- Start CLI for Orsay
- Update Benchmarks
- Handle common errors
- Reactive Renderer
- Testing and stability
See ReactTV's Changelog.
Currently ReactTV is licensed by MIT License
Credits
Thanks react-dom for be example and a inspiration code :)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK