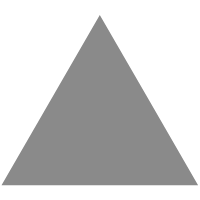
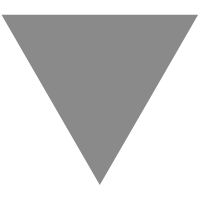
PHP Software Architecture Part 1: MVC – Sameer Nyaupane – Medium
source link: https://medium.com/@sameernyaupane/php-software-architecture-part-1-mvc-1c7bf042a695
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
PHP Software Architecture Part 1: MVC
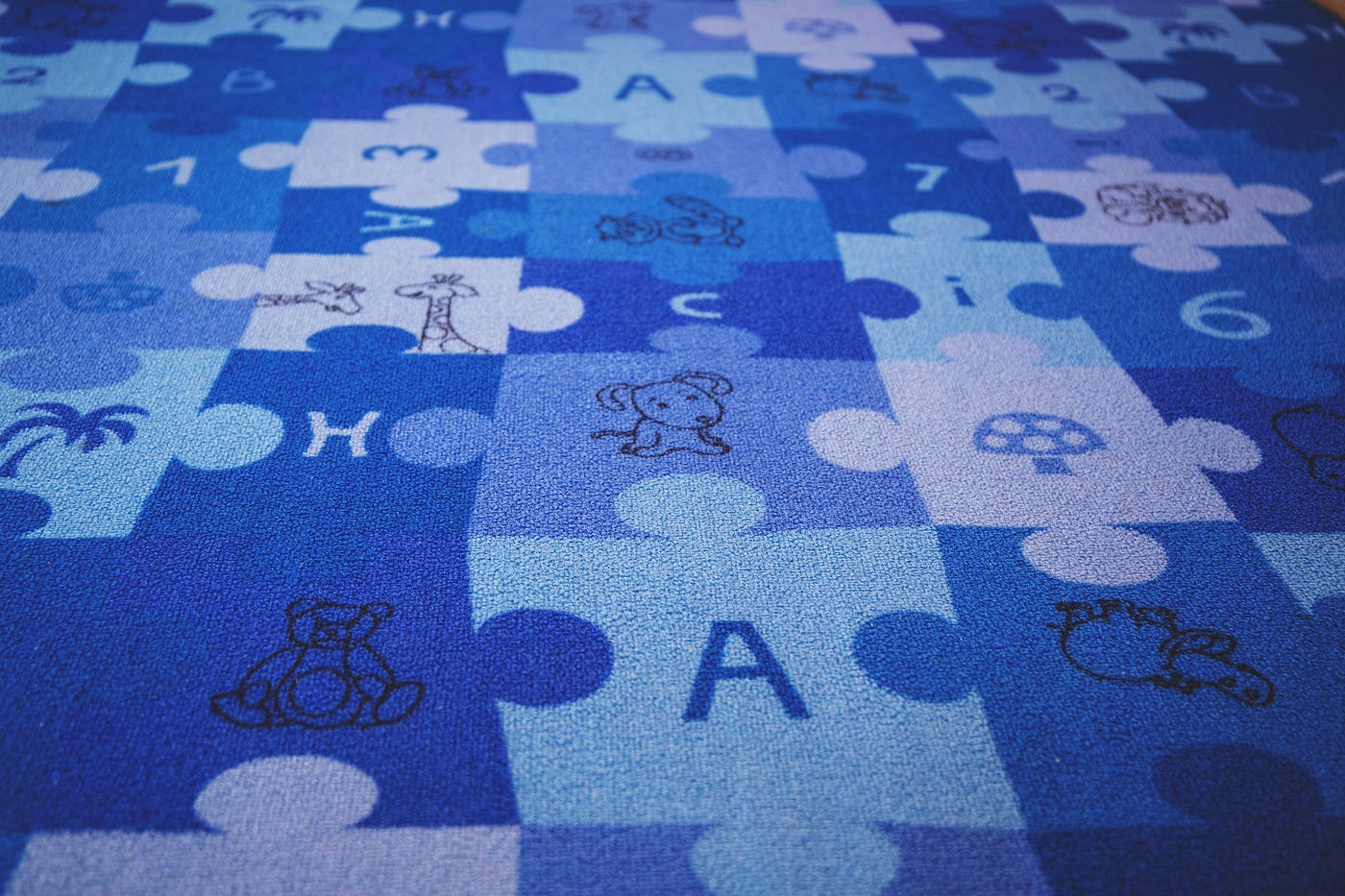
In this series, we will be talking about PHP software architecture. Some may call it PHP application architecture or even PHP web architecture. They’re all talking about the same architecture that we use for organizing our application.
The first software architectural pattern that most of us encounter early as a PHP developer is the Model View Controller (MVC).
Almost every modern PHP framework comes with MVC architecture baked in.
First of all, what is MVC and where did it come from?
MVC (Model-View-Controller) was formulated by Trygve Reenskaug in 1979 for the SmallTalk language.
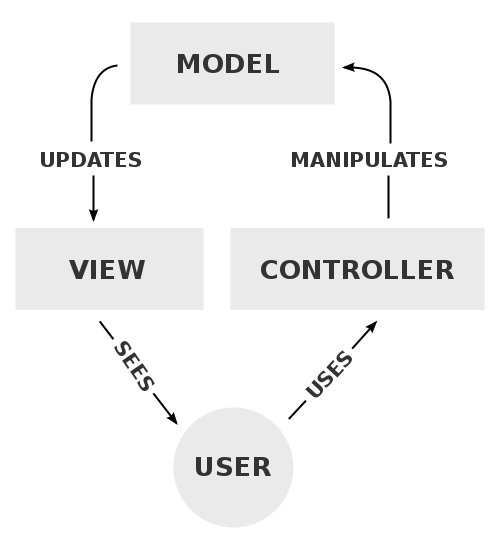
It separates the code into three layers. Model is the representation of all the data used, and the code required to make it persist. The Controller handles requests and updates the model. And lastly, View offers ways to extract and represent the data from the Model
It was originally meant for the GUI interface in desktop applications.
Thus the View actually observed the changes in Model and updated itself automatically. But this approach has changed as it made its way to the web. This is because updating the view automatically is not feasible for the web.
The view is no longer using any observer pattern. It is usually passed the updated Model data by the Controller in almost all of the web frameworks.
For example, Laravel, the most popular PHP framework as of now, uses MVC pattern out of the box.
So, what are the benefits of MVC?
- It helps separate the different parts of an application. This makes it easier to modify and assign developers on different tasks.
- It makes maintaining small applications easy.
And the downsides:
- It does not scale well with bigger applications.
- It encourages fat controllers/or fat models. Causing spaghetti code as the code base grows.
- It limits separation of concerns to only three different parts.
To show an example of how the MVC can become a bottleneck in growing applications, I will be using the Laravel framework for code examples in this series.
Let’s imagine we are working for a travel company.
Our boss comes along and asks us to develop a flight booking system. He wants us to call the third party flight booking API engine to check for available flights. And not only that but to log each response we get to the file system. Finally he wants us to save our data in the database for future use.
The first task that we need to do is to search for available flights through an API.
We think for a while, and go “Aha! We know, we’ll use the Laravel framework with the new MVC pattern that we just read about”.
We quickly start banging some code. After a while let’s say we come up code like below.
Flight Controller:
To breakdown what we are doing in this controller:
First we call the public function “getFlightAvailability” which prepares the user input and then calls the private function “callFlightAvailabilityApi” with necessary inputs supplied. Then this function calls our third party API. The response is then logged by calling yet another private function called “ log” to the file system. After that we get back to the first function “getFlightAvailability” where it invokes another private function called “ saveData” which saves the response we need to the database.
As you can see. There is a lot of stuff going in here. This is actually taken from a fully functional application that I wrote a few years ago. The only difference is, I’ve mashed all the different parts into the Controller, to show the limitation of MVC architecture. In Part 2 of the series, we will go through how we can solve the issues regarding the current approach.
This Controller is a true fat controller that we speak of. It has grown into a monster. It is going to be difficult to update this and maintain.
FlightTicket Model:
The Model here is the Laravel ActiveRecord Model “User”. In Laravel it is a recommended practice to use your Model for relationship and persistence. So due to this reason, we have all our logic in the Controller instead of in the Model.
View:
The view contains the HTML that we use to show the user a formatted output. To keep the post less complex and simple to follow, I’ll let you imagine the view with some HTML tags. Nothing fancy with the Views that we need to worry about anyways.
So we can see that MVC starts to fall apart as soon as we grow out of small CRUD apps. We cannot rely just on MVC to structure our ever growing codebase.
In the next part, I will be discussing on how we can tame this flight search logic with a better architecture. We will go through Repositories, Domain and Service patterns to refactor the above code.
Thank you for reading, and hope you enjoyed it.
Please hit that “Clap” and share it with your friends, if you found it useful! :)
Link to Part 2: https://medium.com/@sameernyaupane/php-software-architecture-part-2-the-alternatives-1bd54e7f7b6d
PHP Software Architecture Part 2: The Alternatives
Before we go into the refactoring process, let’s first look at the alternatives we already have. We will go through…
Check out my other series at:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK