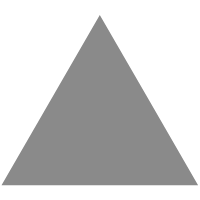
6
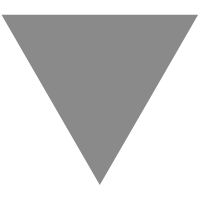
springboot如何集成netty,实现聊天室
source link: https://blog.51cto.com/u_7239686/10243705
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
springboot如何集成netty,实现聊天室
精选 原创要在Spring Boot中集成Netty以实现一个聊天室,你需要以下几个步骤:
- 添加Netty依赖:
在
pom.xml
文件中添加Netty依赖:
<dependency>
<groupId>io.netty</groupId>
<artifactId>netty-all</artifactId>
<version>4.1.52.Final</version>
</dependency>
或者,在Gradle中添加:
implementation 'io.netty:netty-all:4.1.52.Final'
- 创建Netty服务器配置: 创建一个Netty服务器配置类,用于启动Netty服务器并添加所需的channel handlers。
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.http.HttpServerCodec;
import io.netty.handler.codec.http.websocketx.WebSocketFrame;
import io.netty.handler.ssl.SslContext;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class NettyServerConfig {
@Value("${netty.port}")
private int port;
@Bean(destroyMethod = "shutdownGracefully")
EventLoopGroup bossGroup() {
return new NioEventLoopGroup();
}
@Bean(destroyMethod = "shutdownGracefully")
EventLoopGroup workerGroup() {
return new NioEventLoopGroup();
}
@Bean
ServerBootstrap serverBootstrap(EventLoopGroup bossGroup, EventLoopGroup workerGroup) {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) {
ch.pipeline().addLast(new HttpServerCodec());
// Add more handlers here, such as your WebSocket handler.
}
});
return b;
}
@Bean
ChannelFuture serverBootstrapFuture(ServerBootstrap serverBootstrap) {
return serverBootstrap.bind(port).syncUninterruptibly();
}
}
- 创建WebSocket Channel Handler: 创建一个处理WebSocket连接的Channel Handler。
import io.netty.channel.ChannelHandlerContext;
import io.netty.channel.SimpleChannelInboundHandler;
import io.netty.handler.codec.http.websocketx.TextWebSocketFrame;
import io.netty.handler.codec.http.websocketx.WebSocketFrame;
public class WebSocketFrameHandler extends SimpleChannelInboundHandler<WebSocketFrame> {
@Override
protected void channelRead0(ChannelHandlerContext ctx, WebSocketFrame frame) throws Exception {
// Handle text frame which is expected to be the most used frame type.
if (frame instanceof TextWebSocketFrame) {
TextWebSocketFrame textFrame = (TextWebSocketFrame) frame;
// Broadcast received message to all channels.
ctx.channel().writeAndFlush(new TextWebSocketFrame(textFrame.text()));
} else {
String message = "unsupported frame type: " + frame.getClass().getName();
throw new UnsupportedOperationException(message);
}
}
}
- 配置Spring Boot启动类: 在Spring Boot启动类中,添加启动Netty服务器的代码。
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Application implements CommandLineRunner {
private final ChannelFuture serverBootstrapFuture;
public Application(ChannelFuture serverBootstrapFuture) {
this.serverBootstrapFuture = serverBootstrapFuture;
}
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Override
public void run(String... args) {
serverBootstrapFuture.channel().closeFuture().addListener(future -> {
// Perform post-close operations here if you need to.
});
}
}
- 前端集成: 创建一个HTML页面用于WebSocket连接到Netty服务器,并提供聊天界面。
<!DOCTYPE html>
<html>
<head>
<title>Netty Chat Room</title>
</head>
<body>
<textarea id="chat" rows="10" cols="30"></textarea><br>
<input type="text" id="message" placeholder="Enter your message"/>
<button onclick="sendMessage()">Send</button>
<script type="text/javascript">
var socket = new WebSocket("ws://localhost:8080/chatroom");
socket.onmessage = function(event) {
var chat = document.getElementById('chat');
chat.value = chat.value + '\n' + event.data;
};
function sendMessage(){
var message = document.getElementById('message').value;
socket.send(message);
}
</script>
</body>
</html>
在上述代码中,Netty服务器会在定义的端口上启动,并监听WebSocket连接。WebSocketFrameHandler
类将处理所有WebSocket消息。这是一个基础的示例,仅用于说明如何在Spring Boot中集成Netty以及启用WebSocket通信。实际的生产环境中,你可能需要添加更多的安全性、异常处理和消息格式化等功能。
- 赞
- 收藏
- 评论
- 分享
- 举报
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK