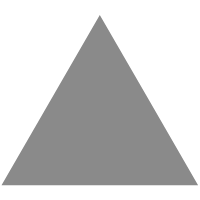
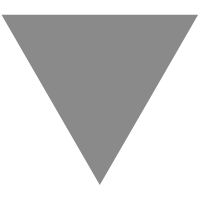
React Micro Frontends with ES Modules
source link: https://blog.bitsrc.io/react-micro-frontends-with-es-modules-bfed32014d3c
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
React Micro Frontends with ES Modules
Creating React Micro Frontends with ES Modules and Bit
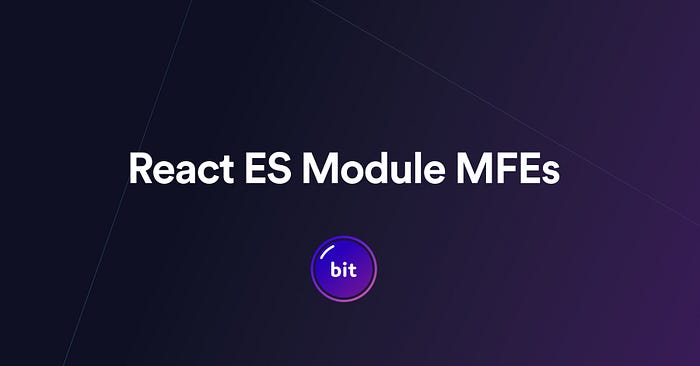
In this tutorial, we’ll explore how to create micro frontends as Bit components, bundle them into ES modules, and deploy them.
We’ll use Bit to share dependencies between the components (the MFE and the host applicaiton) and even standardize their development using reusable development environments.
Create a Bit workspace
We’ll start by creating a new Bit workspace to maintain our micro frontends:
bit new basic my-workspace --default-scope learnbit.react-esm-mfe
Replace the default scope value with your own scope.
Create the MFE and the host application
We’ll use Bit’s out-of-the-box templates to create the MFE and the host (these specific templates are of a Vite React app, but you can use any other template you like).
bit create react-app my-mfe
bit create react-app my-host-app
Run the following to list the available apps in your workspace:
bit app list
The output should look similar to this:
┌───────────────────────────────────┬─────────────┐
│ id │ name │
├───────────────────────────────────┼─────────────┤
│ learnbit.react-es-mfe/my-host-app │ my-host-app │
├───────────────────────────────────┼─────────────┤
│ learnbit.react-es-mfe/my-mfe │ my-mfe │
└───────────────────────────────────┴─────────────┘
To run the apps use the following commands:
bit run my-mfe
bit run my-host-app
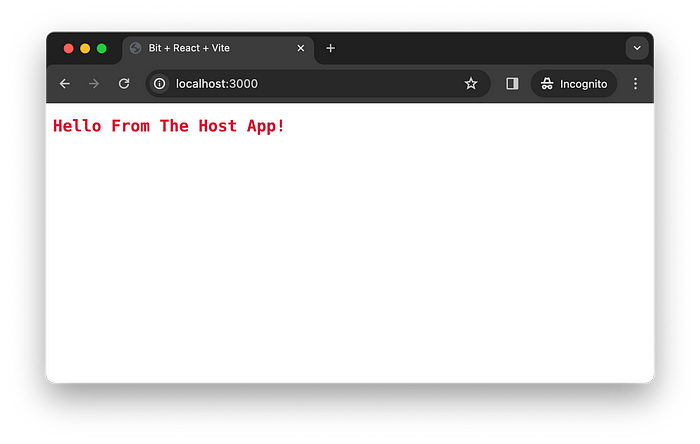
The host app running locally
To explore your workspace using Bit’s UI run:
bit start
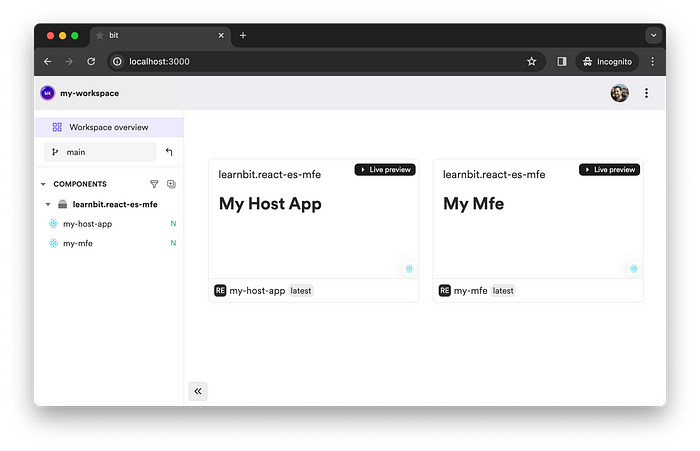
The components explored in Bit’s UI (`bit start`)
Create a reusable development environment
Micro frontends are often used to improve collaboration between teams and allow each team to work independently on its own part of the application.
We’ll export the MFEs (the Bit components) to their remote scopes (remote component hosting) to enjoy these benefits. This will essentially decouple the components from their original workspace and allow other teams to maintain them in whatever repo they choose (or even in no repo at all, by using disposable Bit workspaces).
Before we do that, let’s create a reusable development environment for the MFEs and the host. This will ensure all components are developed and built using the same tools and configurations.
It will also allow us to share dependencies between the MFEs, reducing their bundle size and avoiding conflicts between different versions of the same dependency.
bit create react-env my-react-env
The output should look similar to this:
1 component(s) were created
learnbit.react-es-mfe/my-react-env
location: react-es-mfe/my-react-env
env: teambit.envs/env (set by template)
package: @learnbit/react-es-mfe.my-react-env
Run the following to configure the host app and MFE to use the new environment:
bit envs set my-mfe learnbit.react-es-mfe/my-react-env
bit envs set my-host-app learnbit.react-es-mfe/my-react-env
Dev operations performed on our Bit components, such as bit compile
, bit test
, bit lint
, bit build
, and so on, will use the same tools and configurations, regardless of where these components are maintained. To learn more about reusable development environments, read the official docs:
Create dev environment | Bit
A development environment (env) is a collection of tools, procedures and configurations, used for the development of…
Configure the MFE
A Bit component is defined as an “app” (i.e., a deployable component) when it includes the *.bit-app.ts
file. This file will also configure our app’s build and deployment.
In this case, our Bit component will be configured as a React Vite app deployed to Netlify using the Netlify deployer component.
/** @filename: my-mfe.bit-app.ts */
import { ViteReact } from '@bitdev/react.app-types.vite-react';
import { Netlify } from '@teambit/cloud-providers.deployers.netlify';
const netlifyConfig = {
accessToken: process.env.NETLIFY_AUTH_TOKEN,
productionSiteName: 'bit-react-esm-mfe',
team: 'teambit',
};
export default ViteReact.from({
name: 'my-mfe',
ssr: false,
viteConfigPath: 'vite.config.js',
deploy: Netlify.deploy(netlifyConfig),
});
Head over to the vite.config.js
file to configure the build the remote app:
/** @filename: my-mfe/vite.config.js */
import { defineConfig } from 'vite';
import react from '@vitejs/plugin-react';
import path from 'path';
export default defineConfig({
plugins: [react()],
define: {
'process.env': '{}', // this is to avoid 'process is not defined' error in the browser
},
build: {
lib: {
formats: ['es'], // compile to ES module
entry: 'my-mfe.tsx', // since we share react-dom between the MFEs, we can expose the MFE without the render function
fileName: 'v0.0.1', // it's good practice to version the MFEs
},
rollupOptions: {
external: ['react'],
},
},
});
Consume the MFE by the host application
/** @filename: my-host-app.tsx */
import { lazy, Suspense } from 'react';
function Mfe() {
const MyMfe = lazy(() =>
import('https://bit-react-esm-mfe.netlify.app/v0.0.1.js').then(
(module) => ({
default: module.MyMfe,
})
)
);
return (
<Suspense fallback={<p>Loading...</p>}>
<MyMfe />
</Suspense>
);
}
export function MyHostApp() {
return (
<>
<h2>Hello From The Host App!</h2>
<Mfe />
</>
);
}
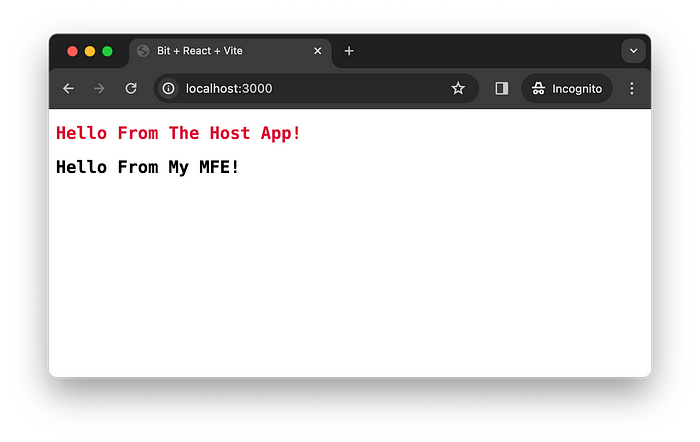
The MFE loaded dynamically by the host application
Release and deploy
Run the following to test, build, deploy, and release a new version of your Bit components. The components will be isolated from the workspace and built using Ripple CI, a CI specifically tailored for Bit components.
Ripple CI runs the build pipeline as it is configured for each component.
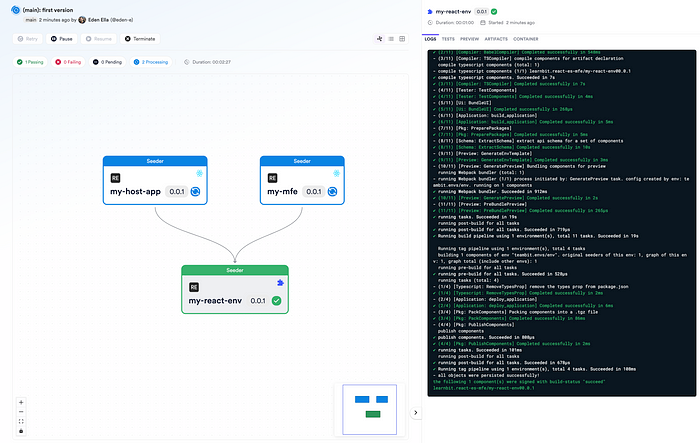
The components built in isolation using Ripple CI
Once the build process is over, the components’ source files and artifacts will be available in their remote scope on bit.cloud.
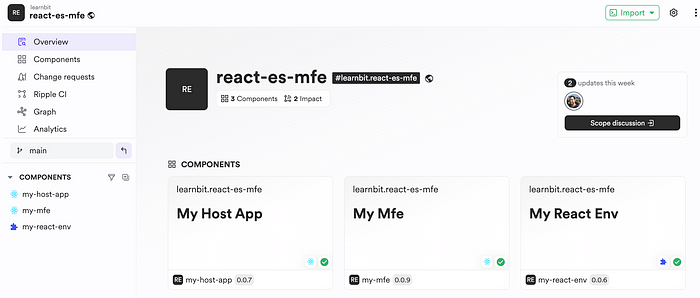
The components in their remote host on bit.cloud
Conclusion
In this tutorial, we’ve explored how to create micro frontends as Bit components, bundle them into ES modules, and deploy them.
We’ve used Bit to share dependencies between the components (the MFEs), and even standardize their development using reusable development environments. That has only been a small taste of what Bit can do for your micro frontend architecture.
By composing applications and micro frontends from independent Bit components, we are able to reuse code across different projects, collaborate more effectively, keep our applications lean and maintainable, and offer our users a consistent experience.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK