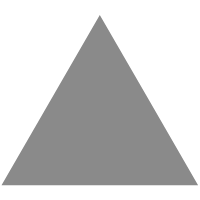
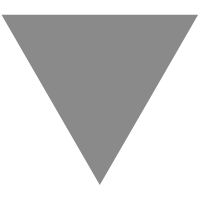
从零开始,使用SwiftUI和PDFKit快速构建完全可定制的PDF阅读器
source link: https://codechina.org/2023/01/swiftui-pdfkit-pdfreader/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
从零开始,使用SwiftUI和PDFKit快速构建完全可定制的PDF阅读器 - Tinyfool的个人网站
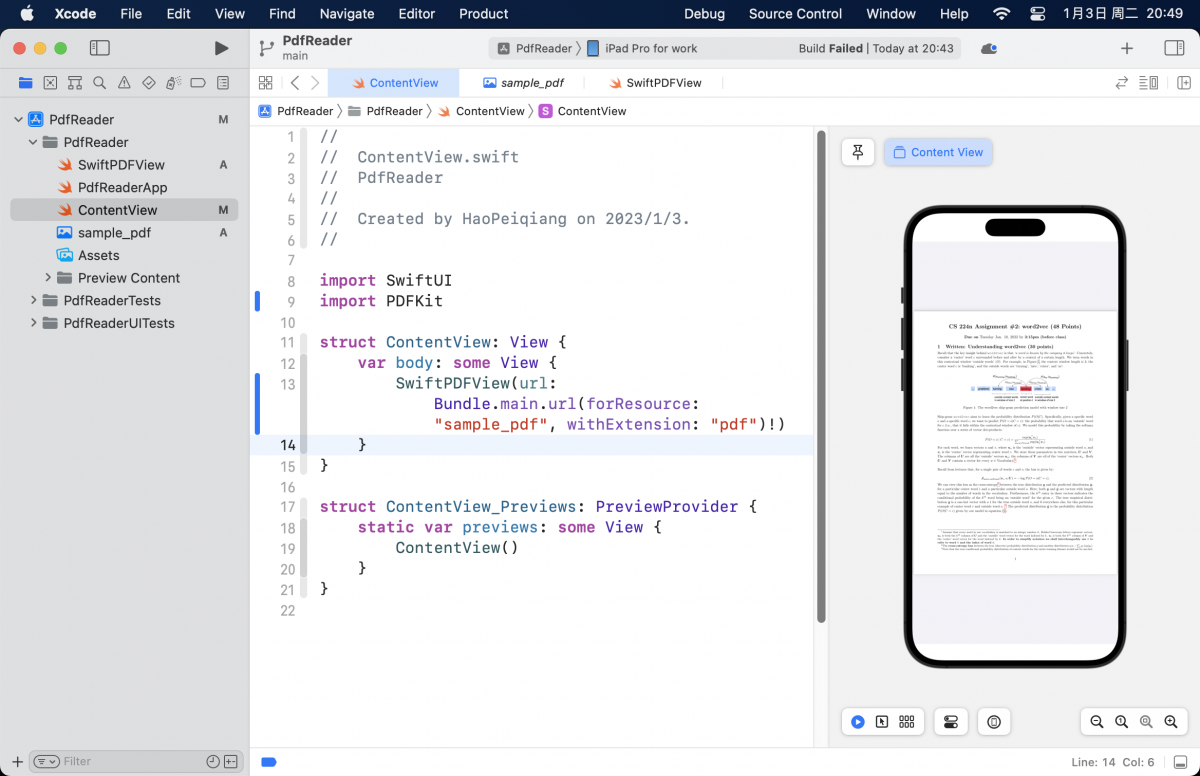
SwiftUI快速创建UI的能力非常强大,苹果还提供了PDFKit框架,所以,用SwiftUI和PDFKit可以用非常少的代码,非常快速的创建一个完全可定制的PDF阅读器。
我们来从零开始,做一个PDF阅读器。
首先,打开Xcode,创建一个新的iOS App。项目名字可以就叫做PdfReader。Interface选择Swift UI,language选择Swift。
因为PDFKit是一个UIKit库,不是原生的SwiftUI对象,所以,我们先需要做一个UIViewRepresentable类,来作为两者之间的桥梁。我已经帮你准备好了一个。你只需要创建一个SwiftPDFView.swift,把如下代码复制进去即可。
//
// SwiftPDFView.swift
// PdfReader
//
// Created by HaoPeiqiang on 2023/1/3.
//
import SwiftUI
import PDFKit
struct SwiftPDFView: UIViewRepresentable {
let url:URL
func makeUIView(context: Context) -> PDFView {
let pdfView = PDFView()
pdfView.document = PDFDocument(url: url)
pdfView.displayMode = .singlePage
pdfView.autoScales = true
pdfView.usePageViewController(true, withViewOptions: nil)
return pdfView
}
func updateUIView(_ pdfView: PDFView, context: Context) {
}
}
struct SwiftPDFView_Previews: PreviewProvider {
static var previews: some View {
SwiftPDFView(url:Bundle.main.url(forResource: "sample_pdf", withExtension: "pdf")!)
}
}
然后,你可以找到自动生成的ContentView.swift文件,把在body内部自动生成的代码删除,加上SwiftPDFView(url: Bundle.main.url(forResource: "sample_pdf", withExtension: "pdf")!)
。好,三分钟不到,你已经成功创建了一个PDF阅读器了。
如果你打开了Xcode代码区域右侧的预览区域,都不用执行App,你已经可以看到你的PDF浏览器已经可以工作了,也可以在PDF页面之间翻页。
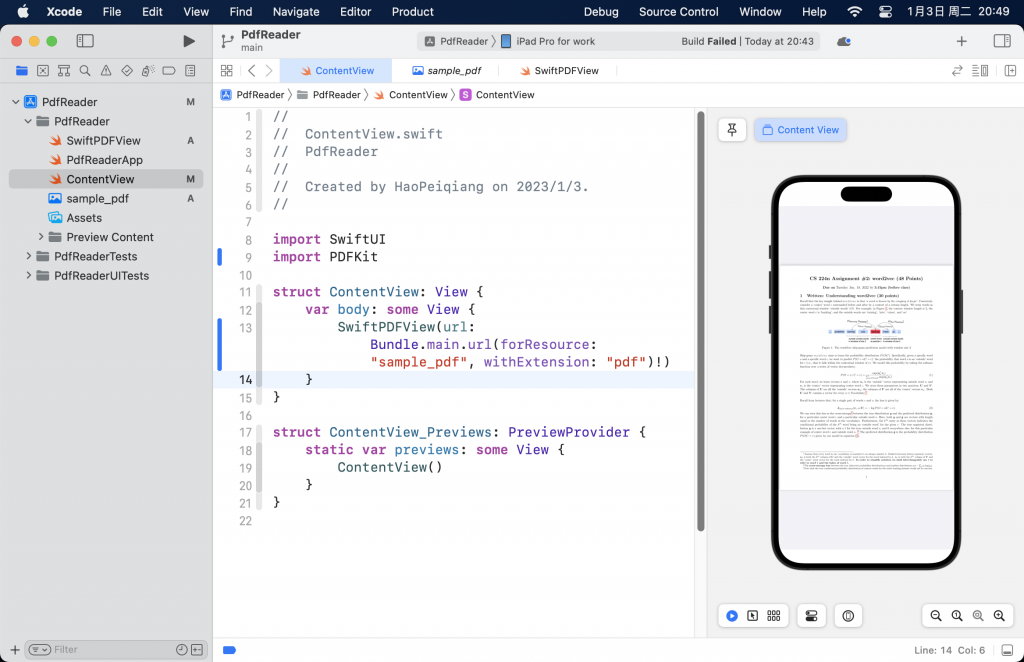
加入缩略图导航栏
你可能见过有的PDF App,在PDF页面下方有一排缩略图,可以导航也可以预览PDF的文件内容,这个叫做PDFThumbnailView
。加入PDFThumbnailView
可以让我们的PDF阅读器,看起来更专业。
我们可以回到SwiftPDFView.swift
文件,在SwiftPDFView类的代码里面加入如下代码:
func setThumbnailView(_ pdfView:PDFView) {
let thumbnailView = PDFThumbnailView()
thumbnailView.translatesAutoresizingMaskIntoConstraints = false
pdfView.addSubview(thumbnailView)
thumbnailView.leadingAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.leadingAnchor).isActive = true
thumbnailView.trailingAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.trailingAnchor).isActive = true
thumbnailView.bottomAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.bottomAnchor).isActive = true
thumbnailView.bottomAnchor.constraint(equalTo: pdfView.safeAreaLayoutGuide.bottomAnchor).isActive = true
thumbnailView.heightAnchor.constraint(equalToConstant: 40).isActive = true
thumbnailView.thumbnailSize = CGSize(width: 20, height: 30)
thumbnailView.layoutMode = .horizontal
thumbnailView.pdfView = pdfView
}
然后在刚才的makeUIView
方法,return pdfView
之前加入一句self.setThumbnailView(pdfView)
即可。
然后,查看预览区域,就会发现PDF显示区域下面出现了缩略图导航栏。
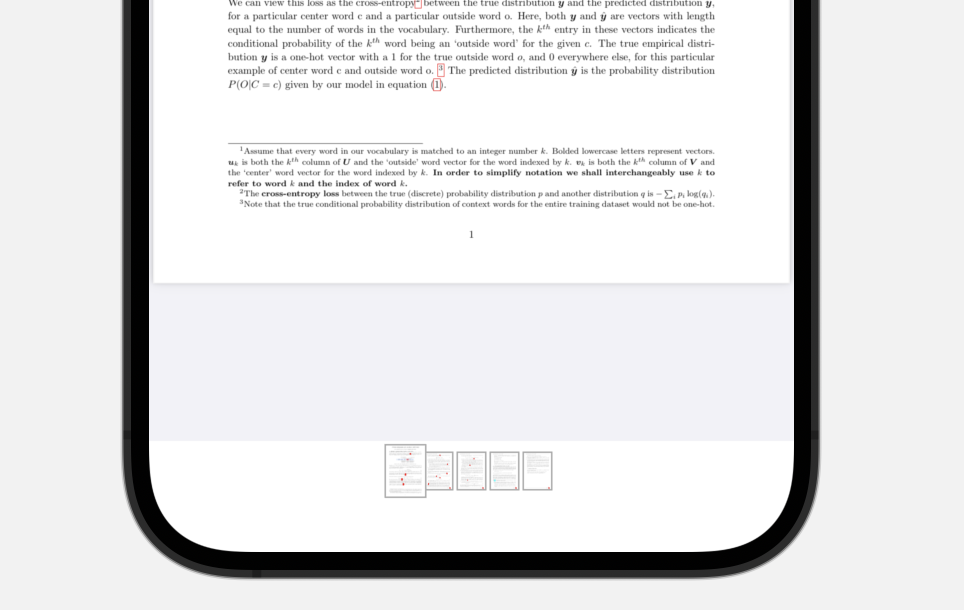
项目代码可从Github下载 https://github.com/Swift-Cast/PdfReader
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK