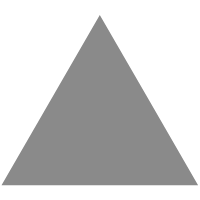
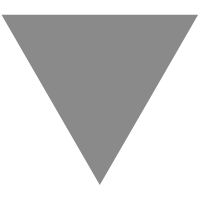
28 Javascript Array Methods: A Cheat Sheet for Developer
source link: https://dev.to/devsmitra/28-javascript-array-hacks-a-cheat-sheet-for-developer-5769
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
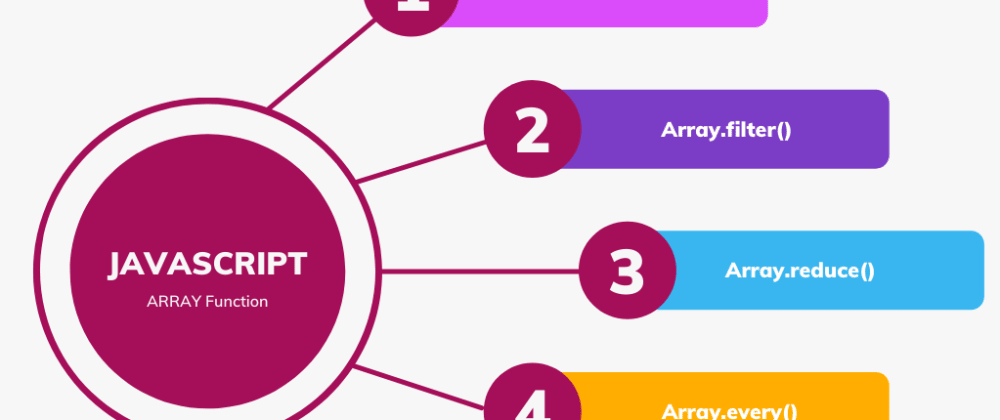
28 Javascript Array Methods: A Cheat Sheet for Developer
Let's understand javascript array functions and how to use them.
Array.map()
Returns a new array with the results of calling a provided function on every element in this array.
const list = [๐ซ, ๐ซ, ๐ซ, ๐ซ];
list.map((โช๏ธ) => ๐); // [๐, ๐, ๐, ๐]
// Code
const list = [1, 2, 3, 4];
list.map((el) => el * 2); // [2, 4, 6, 8]
Enter fullscreen mode
Exit fullscreen mode
Array.filter()
Returns a new array with all elements that pass the test implemented by the provided function.
const list = [๐, ๐ซ, ๐, ๐ซ];
list.filter((โช๏ธ) => โช๏ธ === ๐); // [๐, ๐]
// Code
const list = [1, 2, 3, 4];
list.filter((el) => el % 2 === 0); // [2, 4]
Enter fullscreen mode
Exit fullscreen mode
Array.reduce()
Reduce the array to a single value. The value returned by the function is stored in an accumulator (result/total).
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.reduce((โฌ๏ธ, โช๏ธ) => โฌ๏ธ + โช๏ธ); // ๐ + ๐ซ + ๐ + ๐ซ + ๐คช
// OR
const list = [1, 2, 3, 4, 5];
list.reduce((total, item) => total + item, 0); // 15
Enter fullscreen mode
Exit fullscreen mode
Array.reduceRight()
Executes a reducer function (that you provide) on each element of the array resulting in a single output value(from right to left).
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.reduceRight((โฌ๏ธ, โช๏ธ) => โฌ๏ธ + โช๏ธ); // ๐คช + ๐ซ + ๐ + ๐ซ + ๐
// Code
const list = [1, 2, 3, 4, 5];
list.reduceRight((total, item) => total + item, 0); // 15
Enter fullscreen mode
Exit fullscreen mode
Array.fill()
Fill the elements in an array with a static value.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.fill(๐); // [๐, ๐, ๐, ๐, ๐]
// Code
const list = [1, 2, 3, 4, 5];
list.fill(0); // [0, 0, 0, 0, 0]
Enter fullscreen mode
Exit fullscreen mode
Array.find()
Returns the value of the first element in the array that satisfies the provided testing function. Otherwise undefined is returned.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.find((โช๏ธ) => โช๏ธ === ๐); // ๐
list.find((โช๏ธ) => โช๏ธ === ๐); // undefined
// Code
const list = [1, 2, 3, 4, 5];
list.find((el) => el === 3); // 3
list.find((el) => el === 6); // undefined
Enter fullscreen mode
Exit fullscreen mode
Array.indexOf()
Returns the first index at which a given element can be found in the array, or -1 if it is not present.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.indexOf(๐); // 0
list.indexOf(๐ก); // -1
// Code
const list = [1, 2, 3, 4, 5];
list.indexOf(3); // 2
list.indexOf(6); // -1
Enter fullscreen mode
Exit fullscreen mode
Array.lastIndexOf()
Returns the last index at which a given element can be found in the array, or -1 if it is not present. The array is searched backwards, starting at fromIndex.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.lastIndexOf(๐); // 3
list.lastIndexOf(๐, 1); // 0
// Code
const list = [1, 2, 3, 4, 5];
list.lastIndexOf(3); // 2
list.lastIndexOf(3, 1); // -1
Enter fullscreen mode
Exit fullscreen mode
Array.findIndex()
Returns the index of the first element in the array that satisfies the provided testing function. Otherwise -1 is returned.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.findIndex((โช๏ธ) => โช๏ธ === ๐); // 0
// You might be thinking how it's different from `indexOf` ๐ค
const array = [5, 12, 8, 130, 44];
array.findIndex((element) => element > 13); // 3
// OR
const array = [{
id: ๐
}, {
id: ๐ซ
}, {
id: ๐คช
}];
array.findIndex((element) => element.id === ๐คช); // 2
Enter fullscreen mode
Exit fullscreen mode
Array.includes()
Returns true if the given element is present in the array.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.includes(๐); // true
// Code
const list = [1, 2, 3, 4, 5];
list.includes(3); // true
list.includes(6); // false
Enter fullscreen mode
Exit fullscreen mode
Array.pop()
Removes the last element from an array and returns that element.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.pop(); // ๐คช
list; // [๐, ๐ซ, ๐, ๐ซ]
// Code
const list = [1, 2, 3, 4, 5];
list.pop(); // 5
list; // [1, 2, 3, 4]
Enter fullscreen mode
Exit fullscreen mode
Array.push()
Appends new elements to the end of an array, and returns the new length.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.push(๐ก); // 5
list; // [๐, ๐ซ, ๐, ๐ซ, ๐คช, ๐ก]
// Code
const list = [1, 2, 3, 4, 5];
list.push(6); // 6
list; // [1, 2, 3, 4, 5, 6]
Enter fullscreen mode
Exit fullscreen mode
Array.shift()
Removes the first element from an array and returns that element.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.shift(); // ๐
list; // [๐ซ, ๐, ๐ซ, ๐คช]
// Code
const list = [1, 2, 3, 4, 5];
list.shift(); // 1
list; // [2, 3, 4, 5]
Enter fullscreen mode
Exit fullscreen mode
Array.unshift()
Adds new elements to the beginning of an array, and returns the new length.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.unshift(๐ก); // 6
list; // [๐ก, ๐, ๐ซ, ๐, ๐ซ, ๐คช]
// Code
const list = [1, 2, 3, 4, 5];
list.unshift(0); // 6
list; // [0, 1, 2, 3, 4, 5]
Enter fullscreen mode
Exit fullscreen mode
Array.splice()
Changes the contents of an array by removing or replacing existing elements and/or adding new elements in place.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.splice(1, 2); // [๐, ๐ซ]
list; // [๐, ๐ซ, ๐คช]
// Code
const list = [1, 2, 3, 4, 5];
list.splice(1, 2); // [2, 3]
list; // [1, 4, 5]
Enter fullscreen mode
Exit fullscreen mode
Array.slice()
Returns a shallow copy of a portion of an array into a new array object selected from begin to end (end not included). The original array will not be modified.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.slice(1, 3); // [๐ซ, ๐]
list; // [๐, ๐ซ, ๐, ๐ซ, ๐คช]
// Code
const list = [1, 2, 3, 4, 5];
list.slice(1, 3); // [2, 3]
list; // [1, 2, 3, 4, 5]
Enter fullscreen mode
Exit fullscreen mode
Array.join()
Joins all elements of an array into a string.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.join('ใฐ๏ธ'); // "๐ใฐ๏ธ๐ซใฐ๏ธ๐ใฐ๏ธ๐ซใฐ๏ธ๐คช"
// Code
const list = [1, 2, 3, 4, 5];
list.join(', '); // "1, 2, 3, 4, 5"
Enter fullscreen mode
Exit fullscreen mode
Array.reverse()
Reverses the order of the elements in an array.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.reverse(); // [๐คช, ๐ซ, ๐, ๐ซ, ๐]
list; // [๐คช, ๐ซ, ๐, ๐ซ, ๐]
// Code
const list = [1, 2, 3, 4, 5];
list.reverse(); // [5, 4, 3, 2, 1]
list; // [5, 4, 3, 2, 1]
Enter fullscreen mode
Exit fullscreen mode
Array.sort()
Sorts the elements of an array in place and returns the array. The default sort order is according to string Unicode code points.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.sort(); // [๐, ๐, ๐ซ, ๐ซ, ๐คช]
// This make more sense ๐ค
const array = ['D', 'B', 'A', 'C'];
array.sort(); // ๐ ['A', 'B', 'C', 'D']
// OR
const array = [4, 1, 3, 2, 10];
array.sort(); // ๐ง [1, 10, 2, 3, 4]
array.sort((a, b) => a - b); // ๐ [1, 2, 3, 4, 10]
Enter fullscreen mode
Exit fullscreen mode
Array.some()
Returns true if at least one element in the array passes the test implemented by the provided function.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.some((โช๏ธ) => โช๏ธ === ๐); // true
list.some((โช๏ธ) => โช๏ธ === ๐ก); // false
// Code
const list = [1, 2, 3, 4, 5];
list.some((el) => el === 3); // true
list.some((el) => el === 6); // false
Enter fullscreen mode
Exit fullscreen mode
Array.every()
Returns true if all elements in the array pass the test implemented by the provided function.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.every((โช๏ธ) => โช๏ธ === ๐); // false
const list = [๐, ๐, ๐, ๐, ๐];
list.every((โช๏ธ) => โช๏ธ === ๐); // true
// Code
const list = [1, 2, 3, 4, 5];
list.every((el) => el === 3); // false
const list = [2, 4, 6, 8, 10];
list.every((el) => el%2 === 0); // true
Enter fullscreen mode
Exit fullscreen mode
Array.from()
Creates a new array from an array-like or iterable object.
const list = ๐๐ซ๐๐ซ๐คช;
Array.from(list); // [๐, ๐ซ, ๐, ๐ซ, ๐คช]
const set = new Set(['๐', '๐ซ', '๐', '๐ซ', '๐คช']);
Array.from(set); // [๐, ๐ซ, ๐คช]
const range = (n) => Array.from({ length: n }, (_, i) => i + 1);
console.log(range(10)); // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Enter fullscreen mode
Exit fullscreen mode
Array.of()
Creates a new array with a variable number of arguments, regardless of number or type of the arguments.
const list = Array.of(๐, ๐ซ, ๐, ๐ซ, ๐คช);
list; // [๐, ๐ซ, ๐, ๐ซ, ๐คช]
// Code
const list = Array.of(1, 2, 3, 4, 5);
list; // [1, 2, 3, 4, 5]
Enter fullscreen mode
Exit fullscreen mode
Array.isArray()
Returns true if the given value is an array.
Array.isArray([๐, ๐ซ, ๐, ๐ซ, ๐คช]); // true
Array.isArray(๐คช); // false
// Code
Array.isArray([1, 2, 3, 4, 5]); // true
Array.isArray(5); // false
Enter fullscreen mode
Exit fullscreen mode
Array.at()
Returns a value at the specified index.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.at(1); // ๐ซ
// Return from last ๐ค
list.at(-1); // ๐คช
list.at(-2); // ๐ซ
// Code
const list = [1, 2, 3, 4, 5];
list.at(1); // 2
list.at(-1); // 5
list.at(-2); // 4
Enter fullscreen mode
Exit fullscreen mode
Array.copyWithin()
Copies array elements within the array. Returns the modified array.
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.copyWithin(1, 3); // [๐, ๐, ๐คช, ๐ซ, ๐คช]
const list = [๐, ๐ซ, ๐, ๐ซ, ๐คช];
list.copyWithin(0, 3, 4); // [๐ซ, ๐ซ, ๐, ๐ซ, ๐คช]
// Code
const list = [1, 2, 3, 4, 5];
list.copyWithin(0, 3, 4); // [4, 2, 3, 4, 5]
Enter fullscreen mode
Exit fullscreen mode
NOTE: ๐ค
- first argument is the target at which to start copying elements from.
- second argument is the index at which to start copying elements from.
- third argument is the index at which to stop copying elements from.
Array.flat()
Returns a new array with all sub-array elements concatenated into it recursively up to the specified depth.
const list = [๐, ๐ซ, [๐, ๐ซ, ๐คช]];
list.flat(Infinity); // [๐, ๐ซ, ๐, ๐ซ, ๐คช]
// Code
const list = [1, 2, [3, 4, [5, 6]]];
list.flat(Infinity); // [1, 2, 3, 4, 5, 6]
Enter fullscreen mode
Exit fullscreen mode
Array.flatMap()
Returns a new array formed by applying a given callback function to each element of the array,
const list = [๐, ๐ซ, [๐, ๐ซ, ๐คช]];
list.flatMap((โช๏ธ) => [โช๏ธ, โช๏ธ + โช๏ธ ]); // [๐, ๐๐, ๐ซ, ๐ซ๐ซ, ๐, ๐๐, ๐ซ, ๐ซ๐ซ, ๐คช, ๐คช๐คช]
// Code
const list = [1, 2, 3];
list.flatMap((el) => [el, el * el]); // [1, 1, 2, 4, 3, 9]
Enter fullscreen mode
Exit fullscreen mode
Must Read If you haven't
13 Typescript Utility: A Cheat Sheet for Developer
How to solve Express.js REST API routing problem with typescript decorators?
Javascript short reusable functions trick and tips
More content at Dev.to.
Catch me on Github, Twitter, LinkedIn, Medium, and Stackblitz.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK