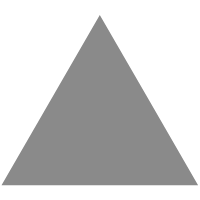
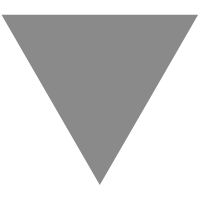
Speaking from scratch: 3. Arrays and collections
source link: https://blog.birost.com/a?ID=00000-07ff845c-7134-4f19-953d-132afccda936
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Array
1. Concept and use
-
Definition A variable can only store one data. If you want to store multiple data, you need to use an array to complete the data collection of the same type. The array is actually a container
-
The characteristics of the array: It is required to store the same type of data The number of elements must be clarified when storing
-
substance
Array is a kind of java container Array is actually a container that stores a fixed number and must be the same type of elements
-
classification
One-dimensional array Two-dimensional array Multi-dimensional array
-
Format of one-dimensional array declaration
Format 1: Element type [] Array name = new element type [number of elements or length of array];
Format 2: Element type [] Array name = new element type [] {element 1 , element 2 , element 3. ..};
Simplified version Element type [] Array name = {element 1 , element 2 , element 3. ..}; copy the code
- Common exceptions in arrays
- Enhanced for loop
for (type of loop variable name of loop variable: object to be traversed) { Loop body content; }
Object to be traversed: can be an array or a collection Loop variable type: actually the data type of the array The name of the loop variable: can be customized, iteratively display the content - each data stored in the array
Benefits: For containers, the traversal process is executed by the compiler, and the enhanced for loop is faster than ordinary for (http://www.cocoachina.com/articles/65360) Disadvantages: In the process of traversal, the subscript of the array cannot be output Copy code
-
Find the maximum
Define a variable, save the current maximum value and traverse the for loop. When a larger value is encountered, change the maximum value of the current record. After the loop ends, you can get the maximum value
-
Common methods of the Arrays class:
2. Sorting and searching
- Bubble Sort
- Select sort
- Insertion sort
Best efficiency: https: //blog.csdn.net/every__day/article/details/83419170
public static int [] sortI( int [] ins){ for ( int i = 1 ; i <ins.length; i ++){ for ( int j = i; j> 0 ; j --){ if (ins [j] <ins [j- 1 ]){ int temp = ins[j]; ins[j] = ins[j- 1 ]; ins[j- 1 ] = temp; } } } return ins; } Copy code
4. Binary search
Three. ArrayUntils
Methods in ArrayUtils:
1.add(): Add the given data to the specified array and return a new array.
2.addAll(): Merge two arrays.
3. contains(): Check whether the data exists in the array and return a boolean value.
4. getLength(): Returns the length of the array.
5.indexOf(): Query whether there is a specified value in the array starting from the first bit of the array, there is a value to return index, otherwise it returns -1.
6.lastIndexOf(): Query whether there is a specified value in the array starting from the last bit of the array, and there is a value that returns index, otherwise it returns -1.
7. Insert(): Add the specified element to the array at the specified position, and return a new array.
8. isEmpty(): Determine whether the array is empty and return a boolean value.
9.isNotEmpty(): Determine whether the array is empty, not null.
10.isSameLength(): Determine whether the length of the two arrays is the same. When the array is empty, the length is 0. Returns a boolean value.
11.isSameType(): Determine whether the types of the two arrays are the same, and return a boolean value.
12.isSorted(): Determine whether the array is sorted according to the natural arrangement order, and return a boolean value.
13.nullToEmpty():
14.remove(): delete the element at the specified position of the array and return a new array.
15.removeAll(): delete the element at the specified position and return a new array.
16.removeAllOccurences(): Remove the specified element from the array and return a new array.
17.removeElement(): Remove the specified element that appears for the first time from the array and return a new array.
18.removeElements(): Remove a specified number of elements from the array and return a new array.
19.reverse(): The array is reversed. You can also specify the start and end reverse positions.
20.subarray(): Intercept the array (the header does not include the tail) and return a new array.
21.swap(): Specify the elements of the two positions of the array to be exchanged or specify the two positions and add the length element of len to exchange.
22.toMap(): Convert the array to Map and return a collection of Map Objects.
23.toObject(): Convert an array of primitive data types into an array of object types.
24.toPrimitive(): Convert an array of object types into an array of primitive data types.
25.toString(): Output the array as Stirng and return a string.
26.toStringArray(): Convert Object array to String array type. Copy code
public class ArraryTest {
public static void main (String[] args) { int []array={ 4 , 5 , 9 }; //add()The result of adding method is: {4,5,9,6} int [] newArray=ArrayUtils.add(array, 6 ); System.out.println(ArrayUtils.toString(newArray)); //addAll() method, the result is: {4,5,9,5,9,6,7} int []arrayAll={ 4 , 5 , 9 }; int [] newArrayAll=ArrayUtils.addAll(arrayAll, 5 , 9 , 6 , 7 ); System.out.println(ArrayUtils.toString(newArrayAll)); //contains(): The result is: true, false System.out.println(ArrayUtils.contains(arrayAll, 9 )); System.out.println(ArrayUtils.contains(arrayAll, 3 )); //getLength(): The result is 3 System.out.println(ArrayUtils.getLength(arrayAll)); //indexOf(): 2. //indexOf(newArrayAll, 9, 3): 3 is to specify which bit to start the search from and return the result 4 System.out.println(ArrayUtils.indexOf(newArrayAll, 9 )); System.out.println(ArrayUtils.indexOf(newArrayAll, 9 , 3 )); //lastIndexOf() returns 4, 2 System.out.println(ArrayUtils.lastIndexOf(newArrayAll, 9 )); System.out.println(ArrayUtils.lastIndexOf(newArrayAll, 9 , 3 )); //insert(): The result is {4,5,3,9} int [] arr=ArrayUtils.insert( 2 , arrayAll, 3 ); System.out.println( "insert" +ArrayUtils.toString(arr)); //isEmpty(): The result is false, true int []a = null ; System.out.println(ArrayUtils.isEmpty(arr)); System.out.println(ArrayUtils.isEmpty(a)); //isNotEmpty(): The result is false, true System.out.println( "isNotEmpty:" +ArrayUtils.isNotEmpty(a)); System.out.println( "isNotEmpty:" +ArrayUtils.isNotEmpty(arr)); //isSorted(): The result is false and true int []sort1={ 5 , 6 , 9 , 1 }; int [] sort2={ 1 , 6 , 8 , 9 }; System.out.println( "sort1:" +ArrayUtils.isSorted(sort1)); System.out.println( "sort2:" +ArrayUtils.isSorted(sort2)); //remove(): The returned result is {5,6,1} int [] newRe=ArrayUtils.remove(sort1, 2 ); for ( int nr:newRe){ System.out.print(nr); } //reverse(): return new reverse: {1,9,6,5} ArrayUtils.reverse(sort1); System.out.println( "new reverse:" +ArrayUtils.toString(sort1)); //subarray(): return result subarray:{3,9} int [] sub={ 7 , 5 , 3 , 9 , 8 , 4 }; int [] newsub=ArrayUtils.subarray(sub, 2 , 4 ); System.out.println( "subarray:" +ArrayUtils.toString(newsub)); Object[] subs={ 7 , 5 , 3 , 9 , 8 , 4 }; Map<Object, Object>map=ArrayUtils.toMap(subs); } } Copy code
1. Concept
1. The origin of the collection
Usually, our program needs to know how many objects to create according to the program running time. But if it is not for the program to run, at the stage of program development, we simply don't know how many objects are needed, or even the exact type of it. In order to meet these conventional programming needs, we require that any number of objects can be created at any time and anywhere, and what should be used to accommodate these objects? We first thought of arrays, but arrays can only hold uniform types of data, and their length is fixed, so what should we do? The collection came into being!
2. What is a collection
The Java collection class is stored in the java.util package, which is a container for storing objects.
Note:
1. The collection can only store objects. For example, if you save an int type data 1 into a collection, it is actually automatically converted to an Integer class and then stored. Every basic type in Java has a corresponding reference type.
2. The collection stores references to multiple objects, and the objects themselves are still stored in heap memory.
3. The collection can store different types and an unlimited number of data types.
3. Java collection framework diagram
This picture comes from: blog.csdn.net/u010887744/...
I found a feature. All the above collection classes, except for the collection of the map series, that is, the collection on the left, all implement the Iterator interface. This is an interface for traversing the elements in the collection. The main ones are hashNext(), next(), and remove(). Kind of method. One of its sub-interfaces, ListIterator , adds three methods to it, namely add(), previous(), hasPrevious(). That is to say, if you implement the Iterator interface, when you traverse the elements in the collection, you can only traverse backwards, and the traversed elements will not be traversed again. Usually, unordered collections implement this interface, such as HashSet; and Those ordered collections of elements generally implement the LinkedIterator interface. The collection that implements this interface can be traversed in both directions. You can access the next element through next() and the previous element through previous(), such as ArrayList.
Another feature is the use of abstract classes. If you want to implement a collection class yourself, it will be very troublesome to implement those abstract interfaces and a lot of work. At this time, you can use abstract classes. These abstract classes provide us with many ready-made implementations. We only need to rewrite some methods or add some methods according to our own needs to implement the collection classes we need, and the workload is greatly reduced.
2. Iterator
1. Iterator concept
Iterator is the top-level interface of Java collections (excluding map series collections, Map interface is the top-level interface of map series collections)
Main method:
Object next () : Returns the reference of the element just passed by the iterator, the return value is Object, which needs to be cast to the type you need
boolean hasNext () : Determine whether there are any accessible elements in the container
void the Remove () : Delete the iterator over the elements just copy the code
For all collections except map series, we can traverse the elements in the collection through iterators.
2. Iterator and Iterable
Note: We can trace back to the top-level interface of the collection in the source code, such as the Collection interface, you can see that it inherits the class Iterable
Then this has to explain the difference between Iterator and Iterable:
Iterable: Exists in the java.lang package.
We can see that it encapsulates the Iterator interface. So as long as you implement the class that implements the Iterable interface, you can use the Iterator iterator.
Iterator: exists in the java.util package. The core methods next(), hasnext(), remove().
3. Collection
The parent interface of List interface and Set interface
Take a look at an example of the use of the Collection collection:
Four. List
1. Concept
An ordered collection that can be repeated.
Since the List interface is inherited from the Collection interface, the basic methods are as shown above.
3.typical implementations of the List interface:
1. List list1 = new ArrayList();
The underlying data structure is an array, the query is fast, the addition and deletion is slow; the thread is not safe, and the efficiency is high
2. List list2 = new Vector();
The underlying data structure is an array, fast query, slow addition and deletion; thread safety, low efficiency, almost eliminated this collection
3. List list3 = new LinkedList();
The underlying data structure is a linked list, which is slow to query and fast to add and delete; threads are not safe and efficient
2.ArrayList
1. ArrayList common API
Modifiers and typesMethod and descriptionbooleanadd(E e) Appends the specified element to the end of this list.voidadd(int index, E element) inserts the specified element into the specified position in this list.booleanaddAll(Collection<? extends E> c) Appends all elements in the specified collection to the end of this list in the order returned by the iterator of the specified collection.booleanaddAll(int index, Collection<? extends E> c) Starting from the specified position, insert all elements in the specified collection into this list.voidclear() Remove all elements from this list.Objectclone() returns a shallow copy of this ArrayList instance.booleancontains(Object o) If this list contains the specified element, it returns true.voidensureCapacity(int minCapacity) If necessary, increase the capacity of this ArrayList instance to ensure that it can hold at least the number of elements specified by the minimum capacity parameter.voidforEach(Consumer<? super E> action) Performs a given operation on each element, Iterable until all elements are processed or the operation raises an exception.Eget(int index) Returns the element at the specified position in this list.intindexOf(Object o) Returns the index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element.booleanisEmpty() returns true if this list contains no elements.Iteratoriterator() returns an iterator of the elements in this list in the proper order.intlastIndexOf(Object o) Returns the index of the last occurrence of the specified element in this list, or -1 if this list does not contain the element.ListIteratorlistIterator() returns a list iterator of the elements in this list (in the correct order).ListIteratorlistIterator(int index) Starting at the specified position in the list, returns a list iterator of the elements in the list (in the correct order).Eremove(int index) Remove the element at the specified position in this list.booleanremove(Object o) Removes the first match of the specified element from this list (if it exists).booleanremoveAll(Collection<?> c) Remove all elements contained in the specified collection from this list.booleanremoveIf(Predicate<? super E> filter) Remove all elements in this set that satisfy the given predicate.protected voidremoveRange(int fromIndex, int toIndex) Remove all elements whose index is between fromIndex and toIndex from this list.voidreplaceAll(UnaryOperator operator) Replaces each element of the list with the result of applying the operator to that element.booleanretainAll(Collection<?> c) retains only the elements contained in the specified collection in this list.Eset(int index, E element) Replace the element at the specified position in this list with the specified element.intsize() returns the number of elements in this list.voidsort(Comparator<? super E> c) Sort this list according to the specified order Comparator .Spliteratorspliterator() creates late binding and fails fast Spliterator on the elements in this list .ListsubList(int fromIndex, int toIndex) returns the specified fromIndex, the view of the part of the list contained and exclusive to toIndex.Object[]toArray() returns an array containing all the elements in this list in the proper order (from the first element to the last element). T[]toArray(T[] a) Returns an array containing all the elements in this list in the proper order (from the first element to the last element); the runtime type of the returned array is the runtime type of the specified array.voidtrimToSize() Adjust the capacity of this ArrayList instance to the current size of the list.
2. Comparing the usage
public void testList () { List<String> list = new ArrayList<>(); list.add( "hello" ); list.add( "world" ); list.add( "java" ); list.add( "python" ); list.add( "php" );
//Select to delete list.removeIf( new Predicate<String>() { @Override public boolean test (String t) { return t.length()> 4 ; } });
//lambda expression list.removeIf(s -> s.equals( "java" ));
//Use iterator to traverse Iterator<String> iterator = list.iterator(); iterator.forEachRemaining( new Consumer<String>() { @Override public void accept (String t) { System.out.println(t); } }); iterator.forEachRemaining(System.out::println); //output list list.stream().forEach(s -> System.out.println(s));
//list is converted to a string array String[] arr = list.toArray( new String[ 0 ]); Arrays.stream(arr).forEach(System.out::println);
//Powerful ListIterator ListIterator<String> lIterator = list.listIterator(); while (lIterator.hasNext()) { System.out.println(lIterator.next()); lIterator.add( "Go" ); } lIterator.forEachRemaining(System.out::println); } Copy code
3. List deduplication
1. String deduplication:
//set collection to remove duplicates , without changing the original order public static void pastLeep1 (List<String> list) { System.out.println( "list = [" + list.toString() + "]" ); List<String> listNew = new ArrayList<>(); Set set = new HashSet(); for (String str:list) { if (set.add(str)){ listNew.add(str); } } System.out.println( "listNew = [" + listNew.toString() + "]" ); }
//After traversing, judge and assign to another list collection public static void pastLeep2 (List<String> list) { System.out.println( "list = [" + list.toString() + "]" ); List<String> listNew = new ArrayList<>(); for (String str:list) { if (!listNew.contains(str)){ listNew.add(str); } } System.out.println( "listNew = [" + listNew.toString() + "]" ); }
//set deduplication public static void pastLeep3 (List<String> list) { System.out.println( "list = [" + list + "]" ); Set set = new HashSet(); List<String> listNew = new ArrayList<>(); set.addAll(list); listNew.addAll(set); System.out.println( "listNew = [" + listNew + "]" ); }
//set deduplication (reduced to one line) public static void pastLeep4 (List<String> list) { System.out.println( "list = [" + list + "]" ); List<String> listNew = new ArrayList<>( new HashSet(list)); System.out.println( "listNew = [" + listNew + "]" ); }
Remove duplication and sort in natural order public static void pastLeep5 (List<String> list) { System.out.println( "list = [" + list + "]" ); List<String> listNew = new ArrayList<>( new TreeSet<String>(list)); System.out.println( "listNew = [" + listNew + "]" ); }
2. Object de-duplication method: package com.hcycom.iams.ncolog;
import java.util.*; import static java.util.Comparator.comparingLong; import static java.util.stream.Collectors.collectingAndThen; import static java.util.stream.Collectors.toCollection;
public class Test {
public static void main (String[] args) { Data data1 = new Data( 1 , "aaaa" ); Data data2 = new Data( 2 , "dddd" ); Data data3 = new Data( 1 , "vvvv" ); Data data4 = new Data( 4 , "rrrr" ); Data data5 = new Data( 1 , "ssss" ); List<Data> list = Arrays.asList(data1,data2,data3,data4,data5); List<Data> l = test2(list); System.out.println(Arrays.toString(l.toArray()));
}
//Object deduplication public static List<Data> test2 (List<Data> list) { List<Data> unique = list.stream().collect( collectingAndThen( toCollection(() -> new TreeSet<>(comparingLong(Data::getId))), ArrayList:: new ) ); return unique; } }
//Entity object class Data {
private int id; private String name;
public Data ( int id, String name) { this .id = id; this .name = name; } } Copy code
3.LinkedList
1. Common API of LinkedList
Modifiers and typesMethod and descriptionbooleanadd(E e) Appends the specified element to the end of this list.voidadd(int index, E element) inserts the specified element into the specified position in this list.booleanaddAll(Collection<? extends E> c) Appends all elements in the specified collection to the end of this list in the order returned by the iterator of the specified collection.booleanaddAll(int index, Collection<? extends E> c) Starting from the specified position, insert all elements in the specified collection into this list.voidaddFirst(E e) Insert the specified element at the beginning of this list.voidaddLast(E e) Appends the specified element to the end of this list.voidclear() Remove all elements from this list.Objectclone() returns a shallow copy of this LinkedList.booleancontains(Object o) true If this list contains the specified element, return.IteratordescendingIterator() returns an iterator of the elements in this deque in reverse order.Eelement() retrieves but does not delete the head (first element) of this list.Eget(int index) Returns the element at the specified position in this list.EgetFirst() EgetLast() intindexOf(Object o) -1 intlastIndexOf(Object o) -1 ListIteratorlistIterator(int index) booleanoffer(E e) booleanofferFirst(E e) booleanofferLast(E e) Epeek() EpeekFirst() null EpeekLast() null Epoll() EpollFirst() null EpollLast() null Epop() voidpush(E e) Eremove() Eremove(int index) booleanremove(Object o) EremoveFirst() booleanremoveFirstOccurrence(Object o) EremoveLast() booleanremoveLastOccurrence(Object o) Eset(int index, E element) intsize() Spliteratorspliterator() Spliterator Object[]toArray() T[]toArray(T[] a) ;
Set()
1.HashSet
1 HashSet API
booleanadd(E e) voidclear() Objectclone() HashSet booleancontains(Object o) set true booleanisEmpty() set true Iteratoriterator() set booleanremove(Object o) intsize() Spliteratorspliterator()
2 HashSet
Set hashSet = new HashSet();
HashSet: equals() true hashCode
1 HashSet HashSet hashCode() hashCode hashCode HashSet
1.1 hashCode hashCode() 1.2 hashCode equals() 1.2.1 hashCode equals true hashSet 1.2.2 hashCode equals false equals() true hashCode hashCode() equals() HashSet equals() true hashCode
3 LinkedHashSet
Set linkedHashSet = new LinkedHashSet();
2.TreeSet
1 TreeSet
Set treeSet = new TreeSet();
TreeSet:
TreeSet() TreeSet , Comparable , null
*.( ) .
2 TreeSet API
booleanadd(E e) booleanaddAll(Collection<? extends E> c) Eceiling(E e) set null voidclear() Objectclone() TreeSet Comparator<? super E>comparator() set set null booleancontains(Object o) true set IteratordescendingIterator() NavigableSetdescendingSet() set Efirst() set Efloor(E e) set null SortedSetheadSet(E toElement) set toElement NavigableSetheadSet(E toElement, boolean inclusive) set inclusive true toElement Ehigher(E e) null booleanisEmpty() true set Iteratoriterator() Elast() Elower(E e) null EpollFirst() null EpollLast() null booleanremove(Object o) intsize() Spliteratorspliterator() Spliterator NavigableSetsubSet(E fromElement, boolean fromInclusive, E toElement, boolean toInclusive) set fromElement to toElement SortedSetsubSet(E fromElement, E toElement) set fromElement toElement SortedSettailSet(E fromElement) set fromElement NavigableSettailSet(E fromElement, boolean inclusive) set inclusive true fromElement
3 TreeSet
Comparable compareTo(Object obj) this > obj, 1 this < obj, -1 this = obj, 0
Comparable compareTo(Object obj) ,
TreeSet , Comparator . : Comparator compare equals()
TreeSet equals() compareTo(Object obj)
3. Set
1 2 Set set = Collections.synchronizedSet(set )
HashSet: equals() true,hashCode() HashSet equals() hashCode()
LinkedHashSet: HashSet HashSet
TreeSet: -
1.Map
1. :
key-value key value
2 Entry(key,value Map Entry
3 Map Collection Iterable Map for-each
2. :
3.Map
2.HashMap
1 HashMap
HashMap() 16 0.75 HashMap HashMap(int initialCapacity) 0.75 HashMap HashMap(int initialCapacity, float loadFactor) HashMap HashMap(Map<? extends K,? extends V> m) Map HashMap
2 HashMap API
voidclear() Objectclone() HashMap Vcompute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) null VcomputeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) null null VcomputeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) null booleancontainsKey(Object key) true booleancontainsValue(Object value) true Set<Map.Entry<K,V>>entrySet() Set voidforEach(BiConsumer<? super K,? super V> action) Vget(Object key) null VgetOrDefault(Object key, V defaultValue) defaultValue booleanisEmpty() - true SetkeySet() Set Vmerge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) null Vput(K key, V value) voidputAll(Map<? extends K,? extends V> m) VputIfAbsent(K key, V value) null null Vremove(Object key) booleanremove(Object key, Object value) Vreplace(K key, V value) booleanreplace(K key, V oldValue, V newValue) voidreplaceAll(BiFunction<? super K,? super V,? extends V> function) intsize() - Collectionvalues() Collection
3.TreeMap
TreeMap() TreeMap(Comparator<? super K> comparator) TreeMap(Map<? extends K,? extends V> m) TreeMap(SortedMap<K,? extends V> m)
Map.Entry<K,V>ceilingEntry(K key) - null KceilingKey(K key) null voidclear() Objectclone() TreeMap Comparator<? super K>comparator() null booleancontainsKey(Object key) true booleancontainsValue(Object value) true NavigableSetdescendingKeySet() NavigableSet NavigableMap<K,V>descendingMap() Set<Map.Entry<K,V>>entrySet() Set Map.Entry<K,V>firstEntry() - null KfirstKey() Map.Entry<K,V>floorEntry(K key) - null KfloorKey(K key) null voidforEach(BiConsumer<? super K,? super V> action) Vget(Object key) null SortedMap<K,V>headMap(K toKey) toKey NavigableMap<K,V>headMap(K toKey, boolean inclusive) inclusive true toKey Map.Entry<K,V>higherEntry(K key) - null KhigherKey(K key) null SetkeySet() Set Map.Entry<K,V>lastEntry() - null KlastKey() Map.Entry<K,V>lowerEntry(K key) - null KlowerKey(K key) null NavigableSetnavigableKeySet() NavigableSet Map.Entry<K,V>pollFirstEntry() - null Map.Entry<K,V>pollLastEntry() - null Vput(K key, V value) voidputAll(Map<? extends K,? extends V> map) Vremove(Object key) TreeMap Vreplace(K key, V value) booleanreplace(K key, V oldValue, V newValue) voidreplaceAll(BiFunction<? super K,? super V,? extends V> function) intsize() - NavigableMap<K,V>subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive) fromKey toKey SortedMap<K,V>subMap(K fromKey, K toKey) fromKey toKey SortedMap<K,V>tailMap(K fromKey) fromKey NavigableMap<K,V>tailMap(K fromKey, boolean inclusive) inclusive true fromKey Collectionvalues() Collection
4. Map Set
-
HashMap HashSet TreeMap TreeSet - LinkedHashMap LinkedHashSet -
-
Set Set Map Key
Java Java Java Java
1.Java
3.Java
Collection Java
Set
List List
Map key value . Map key key value
Queue Dequeue SortedSet SortedMap ListIterator
4. Collection Cloneable Serializable
Collection Collection List Collection Set Collection clone Collection
5. Map Collection
Map Map Map Map Collection
Map Collection Map key-value key value
6.Iterator
7.Enumeration Iterator
8. Iterator.add()
10.Iterater ListIterator
1 Iterator Set List ListIterator List
2 Iterator ListIterator
3 ListIterator Iterator
11. List
List<String> strList = new ArrayList<>(); //for-each for(String obj : strList){ System.out.println(obj); } //using iterator Iterator<String> it = strList.iterator(); while(it.hasNext()){ String obj = it.next(); System.out.println(obj); }
ConcurrentModificationException
12. fail-fast
13.fail-fast fail-safe
14. ConcurrentModificationException
15. Iterator
16.UnsupportedOperationException
17. Java HashMap
HashMap Map.Entry key-value HashMap put get hashCode() equals() key-value put HashMap Key hashCode() key-value Entry LinkedList entry equals() key value entry key get hashCode() equals() Entry
HashMap HashMap 32 0.75 entry map HashMap map 2 key-value HashMap
18.hashCode() equals()
19. Map key
20.Map
Map 1 Set keyset() map key Set map map map Iterator Remove Set.remove removeAll retainAll clear map add addAll
2 Collection values() map value Collection collection map map collection collection map Iterator Remove Set.remove removeAll retainAll clear map add addAll
3 Set<Map.Entry<K,V>> entrySet() map map map collection map entry setValue Iterator Remove Set.remove removeAll retainAll clear map add addAll
21.HashMap HashTable
1 HashMap key value null HashTable
2 HashTable HashMap HashMap HashTable
3 Java1.4 LinkedHashMap HashMap HashMap LinkedHashMap HashTable
4 HashMap key Set fail-fast HashTable key Enumeration fail-fast
5 HashTable Map CocurrentHashMap
22. HashMap TreeMap
23.ArrayList Vector
ArrayList Vector 1
2
3 ArrayList Vector fail-fast
4 ArrayList Vector null
ArrayList Vector 1 Vector ArrayList CopyOnWriteArrayList
2 ArrayList Vector
3 ArrayList Collections
24.Array ArrayList Array
Array ArrayList
Array ArrayList
Array ArrayList addAll removeAll iterator ArrayList Array
1
2 Collections
3 [][] List<List<>>
25.ArrayList LinkedList
ArrayList LinkedList List 1 ArrayList Array O(1) LinkedList O(n) ArrayList
2 ArrayList LinkedList
3 LinkedList ArrayList LinkedList
27.EnumSet
30.BlockingQueue
32.Collections
33.Comparable Comparator
34.Comparable Comparator
Comparable Comparator Comparable
Comparator Comparator
37. synchronized
40. Java
1 Array ArrayList Map TreeMap Set
2
3
4 ClassCastException
5 JDK Map key hashCode() equals()
6 Collections
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK