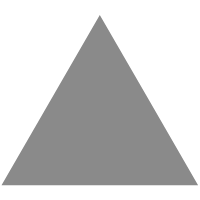
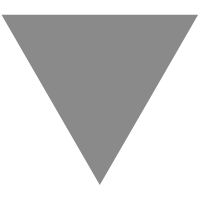
A peek into REST Assured.
source link: https://blog.knoldus.com/a-peek-into-rest-assured/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Hi folks,
In this blog, we will take a peek into the REST Assured and will try to understand the basic architecture of it. Moreover, we will try to have a brief understanding of writing a basic test script as well. So, let’s get going.
What is Rest Assured?
It won’t be an overstatement if we say that APIs are playing a very important role in the software industry nowadays. Hence proper automated test for these API’s are becoming essential. This is where REST Assured comes into play that is for creating tests for APIs.
REST Assured is a Java library that provides a domain-specific language (DSL) for writing powerful, maintainable tests for RESTful APIs. In this blog, we’ll see how to set up REST Assured and how to write and run the tests.
Configuration
We have used Maven as a build tool, therefore this blog will revolve around this build tool only. So to start we need to have the REST Assured dependency
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.3.3</version>
<scope>test</scope>
</dependency>
You can find the latest releases or latest version of this dependency here, https://mvnrepository.com/artifact/io.rest-assured/rest-assured. Here, you can find the dependency for other build tools as well, be it sbt, Gradle etc.
REST Assured can also be easily integrated with other testing frameworks such as TestNg , JUnit etc. In this blog, you’ll se that we have used TestNg as it makes one’s life easier. Through TestNg we get the power to tag our test methods, to add their priority and description as well.
Now, we are ready to move to our test script.
Taking the understanding of the Syntax
For this blog, we have used an open for all API which is Ergast Motor API. This API contains data related to F1 motor racing like drivers, circuits etc. You may find this API here, http://ergast.com/mrd/.
For a sample test script, we have created a test where we retrieve the list of race circuits in JSON format.
@Test(enabled = true)
public void circuitCount() {
given().
when().
get("http://ergast.com/api/f1/2017/circuits.json").
then().
assertThat(). body("MRData.CircuitTable.Circuits.circuitId",hasSize(20));
}
One thing to note here that REST Assured supports the BDD syntax which Given/When/Then. This leads to easy to read and easy to write test cases.
If we look at the code, we may see that the “hasSize()” function is used in the assertion statement. The “hasSize()” counts the number of circuits and checks whether there are 20 circuits or not.
The verification does the following.
- Captures the JSON response
- Make a query all the elements having circuitId
- Verifies that the result has 20 circuitId or not.
There are many more Hamcrest matchers/assertors like “equalTo()”, “lessThan” , “greaterThan()”, “hasItem()” etc. You may find the Hamcrest documentation useful, http://hamcrest.org/JavaHamcrest/ .
Validating other aspects other than the response body.
REST Assured not only give us the ability to validate the response body but the technical aspects of the response as well such as HTTP status code, the response content type and headers as well. Let’s have a look at a sample test script for these.
@Test
public void headersAndStatus() {
given().
when().
get("http://ergast.com/api/f1/2017/circuits.json").
then().
assertThat().
statusCode(200).
and().
contentType(ContentType.JSON).
and().
header("Content-Length",equalTo("4567"));
}
One more thing to point out here is that how easily have concatenated two assertions using the and() method, it makes the code more readable.
Parametrizing our Test
This is where the concept of Data-Driven Testing comes in. RESTful API supports two types of parameters.
- Query Parameter
- Path Parameter
Query Parameter : These are appened at the end of the RESTful API endpoint.
Path Parameter : These are the path of the API endpoint.
REST Assured can handle both types of parameters. Let’s have a look at a sample script for this.
For query parameter
@Test
public void something() {
String originalText = "test";
String trueValue = "someOne";
given().
param("text",originalText).
when().
get("http://md5.jsontest.com").
then().
assertThat().
body("md5",equalTo(trueValue));
}
For Path Parameter
@Test
public void CircuitsShouldBe20() {
String season = "2017";
int numberOfRaces = 20;
given().
pathParam("raceSeason",season).
when().
get("http://ergast.com/api/f1/{raceSeason}/circuits.json").
then().
assertThat().
body("MRData.CircuitTable.Circuits.circuitId",hasSize(numberOfRaces));
}
Instead of the param(), path parameters are defined using the pathParam() method.
Other features of REST Assured.
REST Assured offers a number of other features as well. Such as
- It has the ability to deserialize POJOs. This allows you to serialize the properties and values associated with a Java object instance directly into a JSON or an XML document, which can then be sent to a RESTful API using the POST method
- Logging requests and responses. This can be especially useful when you need to inspect API responses to create the appropriate checks, or when you want to make sure that the request you’re sending to an API is correct.
References
I hope you may have found this useful. Thank you.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK