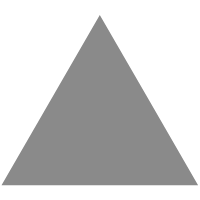
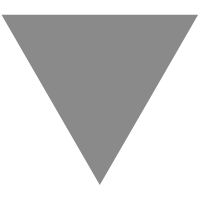
How To Deploy a Next.js App to App Platform
source link: https://www.digitalocean.com/community/tutorials/how-to-deploy-a-next-js-app-to-app-platform
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Tutorial
How To Deploy a Next.js App to App Platform
Node.jsDeploymentDevelopmentJavaScriptDigitalOcean App PlatformNext.js
Next.js is one-of-a-kind framework built on top of React. It lets you build React apps quickly because it comes with features like Image Optimization, Zero Config, Incremental Static Generation, File-system Routing, Code-splitting and Bundling and more.
Next.js deployment is unique in that it lets you deploy a couple different ways:
- Static Export: You can create all the HTML pages for your site. By generating all of your HTML pages, you can serve static files, which guarantees a fast experience across the entire site. This is a good solution if you have fewer pages and aren’t worried about build times to export your pages to HTML.
- Custom Server: You can deploy a Node server that will dynamically generate pages or serve static ones. In larger sites, creating a static export of every page can be a time-consuming process. The dynamic approach is good for when you have thousands of pages and don’t want to wait for long build times during a Static Export.
For large sites, a Static Export may not be the best solution. Static Exports can take a long time for sites that have thousands of pages like e-commerce sites or large blogs. A Node.js Custom Server lets you do static incremental regeneration. Instead of generating your entire site’s HTML at once, you can generate pages on the fly. This is beneficial because you don’t have to wait to build/export your entire site; you can generate it as users visit each page.
In this tutorial, you will deploy a Next.js application to DigitalOcean’s App Platform using a Static Export and a Custom Server. App Platform is a Platform as a Service (PaaS) that lets you deploy applications from a GitHub repo.
Prerequisites
To complete this tutorial, you will need:
- A DigitalOcean account to use App Platform.
- A GitHub account so you can push your repository and connect your account to DigitalOcean.
- A local development environment for Node.js. Follow How to Install Node.js and Create a Local Development Environment.
- A text editor like Visual Studio Code or Atom.
- Familiarity with JavaScript. You can look at the How To Code in JavaScript series to learn more.
Creating a Basic Next.js Application
You’ll need a Next.js application to deploy to App Platform, so in this step you’ll create one with create-next-app, the CLI tool to generate new starter Next.js apps. You can also find the completed app at the DigitalOcean Community GitHub repository.
First, create the Next.js app with the create-next-app
command using npx
:
npx create-next-app my-next-app
This creates the my-next-app
directory, initializes the directory as a Git repository, and makes an initial commit:
Output
npx: installed 1 in 2.201s
Creating a new Next.js app in /Users/brianhogan/Dropbox/work/do/articles/67580_next_app_platform/my-next-app.
Installing react, react-dom, and next using npm...
...
Initialized a git repository.
Success! Created my-next-app at /Users/brianhogan/Dropbox/work/do/articles/67580_next_app_platform/my-next-app
Inside that directory, you can run several commands:
npm run dev
Starts the development server.
npm run build
Builds the app for production.
npm start
Runs the built app in production mode.
We suggest that you begin by typing:
cd my-next-app
npm run dev
Switch to the my-next-app
directory:
cd my-next-app
DigitalOcean App Platform deploys your code from GitHub repositories, so you’ll need to push your local repository to GitHub. The create-next-app
command already made an initial commit, so you don’t have to commit your files first.
Open your browser and navigate to GitHub, log in with your profile, and create a new repository called sharkopedia
. Create an empty repository without a README
or license file.
Once you’ve created the repository, return to the command line to push your local files to GitHub.
First, add GitHub as a remote repository:
git remote add origin https://github.com/your_username/my-next-app
Next, rename the default branch main
, to match what GitHub expects:
git branch -M main
Finally, push your main
branch to GitHub’s main
branch:
git push -u origin main
Your files will transfer.
With your files in GitHub, you can deploy your app. First, you’ll deploy your app as a static site.
Deploying Next.js as a Static Site
To deploy the Next.js app to App Platform as a Static Site, you’ll use Next’s built-in commands to generate all of your HTML files. You first call next build
, followed by next export
. To make it easier, create a single npm run export
command that calls both.
Open package.json
in your editor and add the following export
script to the file:
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start",
"export": "npm run build && next export -o _static"
},
The export
command runs npm run build
first, which in turn runs next build
. It then runs next export
. App Platform looks for HTML files in a _static
folder, so this command uses the -o
switch to create the folder that App Platform wants to see and populate it with the static files.
You can run this command locally and see the new _static folder.
npm run export
The command generates the files:
Output
info - Creating an optimized production build
info - Compiled successfully
info - Collecting page data
info - Finalizing page optimization
Page Size First Load JS
┌ ○ / 3.44 kB 65 kB
├ └ css/9a89afcbe95084ea8b90.css 703 B
├ /_app 0 B 61.6 kB
├ ○ /404 3.44 kB 65 kB
└ λ /api/hello 0 B 61.6 kB
+ First Load JS shared by all 61.6 kB
├ chunks/f6078781a05fe1bcb0902d23dbbb2662c8d200b3.ca31a7.js 11.3 kB
├ chunks/framework.9116e7.js 41.8 kB
├ chunks/main.d1e355.js 7.3 kB
├ chunks/pages/_app.333f97.js 529 B
├ chunks/webpack.e06743.js 751 B
└ css/6e9ef204d6fd7ac61493.css 194 B
You now have to commit your code to GitHub so that you can update your GitHub repo for deploying to App Platform.
First, add the _static
folder to the .gitignore
file so you don’t check it in. Open .gitignore
in your editor and add these lines to the end:
# static folder for DigitalOcean App Platform
_static
Save the file and return to your terminal.
Run the following command to add the changed files:
git add -A
Create a commit:
git commit -m "adding export command"
Push the code to GitHub:
git push
Once the code is pushed, go into your DigitalOcean App Platform Dashboard and create a new app by pressing the Create App button.
Select the GitHub repository that contains your app and press Next:
Choose the region you and your customers are closest to, and ensure that the main
branch is selected. Select Autodeploy code changes to ensure that App Platform redploys your application whenever you push code changes to GitHub:
App Platform will detect that you have a Node.JS app. Change the type from Web Service to Static Site. Then change the build command to use the new export
command you created by entering npm run export
:
Press Next to move to the next step.
On the final page, select the Starter plan and press the Launch Starter App to deploy your app. You’ll see your new live URL in your dashboard.
Visit the link to see your site deployed.
You have successfully deployed Next.js as a Static Site to App Platform. Next, you will see how to deploy Next.js as a Custom Server to take advantage of the Incremental Static Generation feature that Next.js offers.
Deploying Next.js as a Custom Server
In addition to deploying Next as a static site, you can deploy it as a custom server. This means that you are deploying a Node server that can serve pages dynamically or statically.
The process is called incremental static regeneration. Next will wait for a user to visit a page and then generate the static HTML for that page. If a second user visits that same page, Next will serve the static file instead of generating it dynamically.
Next already has this feature built in using its next start
command in the package.json
scripts. All you have to do to use it with App Platform is to pass it a host and a port. App Platform listens by default on 0.0.0.0
on port 8080
.
To configure this, update the start
script in package.json
and specify the port and host. Open package.json
in your editor and modify the start
command so it looks like the following:
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start -H 0.0.0.0 -p ${PORT:-8080}",
...
Save the file.
You now have to commit your code to GitHub so that you can update your GitHub repo for deploying to App Platform. Run the command:
git add -A
Create a commit:
git commit -m "Changing start command for App Platform"
Push the code to GitHub:
git push origin main
Now you can deploy this Next.js app to App Platform as a Web Service. You’ll be deploying from the same GitHub repo. The main differences are that you’ll choose Web Service instead of a Static Site and you’ll also change your build
command.
First, visit your DigitalOcean App Platform Dashboard and create a new app.
Select your repository from GitHub:
Then name the application, choose your region, and ensure you’re using the main
branch:
Press Next to continue.
Then ensure that the Type is set to Web Service. Use npm run build
as the Build Command and npm start
as the Start command:
Press Next to continue.
Now choose the type of plan. Since this isn’t a static site, you won’t be able to use the Starter plan. Choose the Basic plan.
Choose the 1GB Ram | 1 vCPU option for the Container, and leave the Number of Containers set to 1. Then press Launch Basic App.
After a short time, your app is deployed. You can follow the URL displayed to visit your app in the browser.
As you make changes to your code and push them to your main
branch on GitHub, your application will automatically redeploy.
Conclusion
In this tutorial, you deployed Next.js two separate ways: as a Static Site and as a Custom Server. You can use one of the two strategies depending on your app or site’s needs.
Moving forward, you can look further into what App Platform has to offer. You may want to add a Web Service and a Database to create your own API that your Next.js app can consume, or use Next’s API Routes to create a small API. Look through the App Platform docs to see what other features you can take advantage of when building and deploying your apps.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK