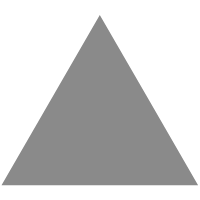
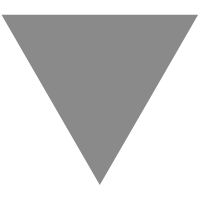
How To Bind Specific Keys to the Keyup and Keydown Events in Angular
source link: https://www.digitalocean.com/community/tutorials/angular-binding-keyup-keydown-events
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Introduction
When binding to either the keyup
or keydown
events in your Angular 2+ templates, you can specify key names. This will apply a filter to be applied to the event, so it will trigger only when specific keys are pressed.
In this article, you will learn how to use key names when listening for keyup
and keydown
events.
Using Key Names
First, let’s look at an example without using a key name.
Let’s say we have an <input>
element for users to provide information. We want to log to the console when the user presses the ENTER
key:
<input (keydown)="onKeydown($event)">
We have bound a keydown
event handler that fires onKeydown()
:
Next, let’s write the onKeydown()
function to handle pressing the ENTER
key:
onKeydown(event) {
if (event.key === "Enter") {
console.log(event);
}
}
A check is performed on every keydown
event to determine if the event.key
value is Enter
. If true
, we log the event
to the console.
Now the same example, but with the addition of the ENTER
key name to the event:
<input (keydown.enter)="onKeydown($event)">
We have bound a keydown.enter
pseudo-event handler that fires onKeydown()
:
Next, let’s rewrite the onKeydown()
function:
onKeydown(event) {
console.log(event);
}
By relying upon Angular’s keydown.enter
pseudo-event, it is no longer necessary to manually check to see if the event.key
value is Enter
.
Using Special Modifier Keys and Combinations
This feature works for special and modifier keys like ENTER
, escape (ESC
), SHIFT
, ALT
, TAB
, BACKSPACE
, and command (meta):
Key(s)
Key Name
ENTER
<input (keydown.enter)="...">
ESC
<input (keydown.esc)="...">
ALT
<input (keydown.alt)="...">
TAB
<input (keydown.tab)="...">
BACKSPACE
<input (keydown.backspace)="...">
CONTROL
<input (keydown.control)="...">
COMMAND
<input (keydown.meta)="...">
But it also works for letters, numbers, arrows, and functional keys (F1
through F12
):
Key(s)
Key Name
A
<input (keydown.a)="...">
9
<input (keydown.9)="...">
ARROWUP
<input (keydown.arrowup)="...">
F4
<input (keydown.f4)="...">
Here is a complete list of Key Values that Angular is capable of filtering.
You can also combine keys together to trigger the event only when the key combination is triggered. In the following example, the event will trigger only if the CONTROL
and 1
keys are pressed at the same time:
<input (keyup.control.1)="onKeydown($event)">
Here are a few more examples to give you an idea of what’s possible:
Key(s)
Key Name
SHIFT+ESC
<input (keydown.shift.esc)="...">
SHIFT+ARROWDOWN
<input (keydown.shift.arrowdown)="...">
SHIFT+CONTROL+Z
<input (keydown.shift.control.z)="...">
Conclusion
You have learned how Angular 2+ templates support filtering key names with keyup
and keydown
pseudo-events.
The benefits of this approach include less repetitive manual checks for key values and handling modifier key and non-modifier key combinations.
If you’d like to learn more about Angular, check out our Angular topic page for exercises and programming projects.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK