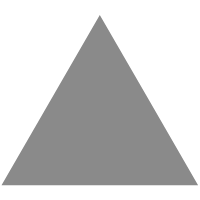
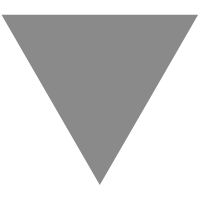
Check if all the elements can be made equal on dividing with X and Y
source link: https://www.geeksforgeeks.org/check-if-all-the-elements-can-be-made-equal-on-dividing-with-x-and-y/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
- Last Updated : 20 Nov, 2019
Given an array arr[] and two integers X and Y. The task is to check whether it is possible to make all the elements equal by dividing them with X and Y any number of times including 0.
Examples:
Input: arr[] = {2, 4, 6, 8}, X = 2, Y = 3
Output: Yes
2 -> 2
4 -> (4 / X) = (4 / 2) = 2
6 -> (6 / Y) = (6 / 3) = 2
8 -> (8 / X) = (8 / 2) = 4 and 4 -> (4 / X) = (4 / 2) = 2Input: arr[] = {2, 4, 10}, X = 11, Y = 12
Output: No
Approach: Find the gcd of all the elements from the given array because this gcd is the value which can we get by dividing all the elements with some arbitrary constants say gcd = arr[0] / k1 or arr[1] / k2 or … or arr[n-1] / kn. Now the task is to find whether these constants k1, k2, k3, …, kn are of the form X * X * X * … * Y Y Y * ….. If yes then it is possible to make all the elements equal with the given operation else it isn’t.
Below is the implementation of the above approach:
- Python3
filter_none
edit
close
play_arrow
link
brightness_4
code
// C++ implementation of the approach
#include <bits/stdc++.h>
using
namespace
std;
// Function that returns true if num
// is of the form x*x*x*...*y*y*...
bool
isDivisible(
int
num,
int
x,
int
y)
{
// While num divisible is divible
// by either x or y, keep dividing
while
(num % x == 0 || num % y == 0) {
if
(num % x == 0)
num /= x;
if
(num % y == 0)
num /= y;
}
// If num > 1, it means it cannot be
// further divided by either x or y
if
(num > 1)
return
false
;
return
true
;
}
// Function that returns true if all
// the array elements can be made
// equal with the given operation
bool
isPossible(
int
arr[],
int
n,
int
x,
int
y)
{
// To store the gcd of the array elements
int
gcd = arr[0];
for
(
int
i = 1; i < n; i++)
gcd = __gcd(gcd, arr[i]);
// For every element of the array
for
(
int
i = 0; i < n; i++) {
// Check if k is of the form x*x*..*y*y*...
// where (gcd * k = arr[i])
if
(!isDivisible(arr[i] / gcd, x, y))
return
false
;
}
return
true
;
}
// Driver code
int
main()
{
int
arr[] = { 2, 4, 6, 8 };
int
n =
sizeof
(arr) /
sizeof
(arr[0]);
int
x = 2, y = 3;
if
(isPossible(arr, n, x, y))
cout <<
"Yes"
;
else
cout <<
"No"
;
return
0;
}
Yes
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK