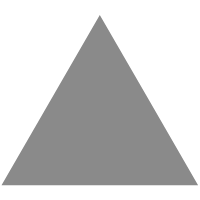
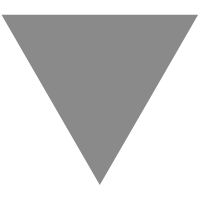
Strings from an array which are not prefix of any other string
source link: https://www.geeksforgeeks.org/strings-from-an-array-which-are-not-prefix-of-any-other-string/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
- Difficulty Level : Medium
- Last Updated : 16 Oct, 2019
Given an array arr[] of strings, the task is to print the strings from the array which are not prefix of any other string from the same array.
Examples:
Input: arr[] = {“apple”, “app”, “there”, “the”, “like”}
Output:
apple
like
there
Here “app” is a prefix of “apple”
Hence, it is not printed and
“the” is a prefix of “there”Input: arr[] = {“a”, “aa”, “aaa”, “aaaa”}
Output:
aaaa
Naive approach: For every string of the array, we check if it is prefix of any other string. If it is then don’t display it.
Efficient approach: We pick strings from array one by one and insert it into Trie. Then there are two cases for the insertion of the string:
- While inserting if we found that the picked string is a prefix of an already inserted string then we don’t insert this string into the Trie.
- If a prefix is inserted first into the Trie and afterwards we find that the string is a prefix of some word then we simply make isEndOfWord = false for that particular node.
After constructing the Trie, we traverse it and display all the words in the Trie.
Below is the implementation of the above approach:
filter_none
edit
close
play_arrow
link
brightness_4
code
// C++ implementation of the approach
#include <bits/stdc++.h>
using
namespace
std;
const
int
ALPHABET_SIZE = 26;
// Trie node
struct
TrieNode {
struct
TrieNode* children[ALPHABET_SIZE];
// isEndOfWord is true if the node represents
// end of a word
bool
isEndOfWord;
};
// Returns new trie node (initialized to NULLs)
struct
TrieNode* getNode(
void
)
{
struct
TrieNode* pNode =
new
TrieNode;
pNode->isEndOfWord =
false
;
for
(
int
i = 0; i < ALPHABET_SIZE; i++)
pNode->children[i] = NULL;
return
pNode;
}
// Function to insert a string into trie
void
insert(
struct
TrieNode* root, string key)
{
struct
TrieNode* pCrawl = root;
for
(
int
i = 0; i < key.length(); i++) {
int
index = key[i] -
'a'
;
if
(!pCrawl->children[index])
pCrawl->children[index] = getNode();
// While inerting a word make
// each isEndOfWord as false
pCrawl->isEndOfWord =
false
;
pCrawl = pCrawl->children[index];
}
int
i;
// Check if this word is prefix of
// some already inserted word
// If it is then don't insert this word
for
(i = 0; i < 26; i++) {
if
(pCrawl->children[i]) {
break
;
}
}
// If present word is not prefix of
// any other word then insert it
if
(i == 26) {
pCrawl->isEndOfWord =
true
;
}
}
// Function to display words in Trie
void
display(
struct
TrieNode* root,
char
str[],
int
level)
{
// If node is leaf node, it indicates end
// of string, so a null character is added
// and string is displayed
if
(root->isEndOfWord) {
str[level] =
'\0'
;
cout << str << endl;
}
int
i;
for
(i = 0; i < ALPHABET_SIZE; i++) {
// If NON NULL child is found
// add parent key to str and
// call the display function recursively
// for child node
if
(root->children[i]) {
str[level] = i +
'a'
;
display(root->children[i], str, level + 1);
}
}
}
// Driver code
int
main()
{
string keys[] = {
"apple"
,
"app"
,
"there"
,
"the"
,
"like"
};
int
n =
sizeof
(keys) /
sizeof
(string);
struct
TrieNode* root = getNode();
// Construct trie
for
(
int
i = 0; i < n; i++)
insert(root, keys[i]);
char
str[100];
display(root, str, 0);
return
0;
}
apple like there
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK