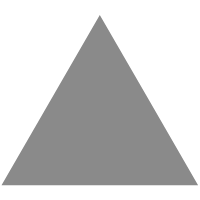
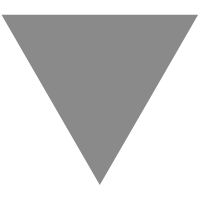
Introduction to Node.js
source link: https://www.tuicool.com/articles/YBfemuz
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Nodejs Background
Node.js was first introduced to the community at JS-EU conference in 2009 by its creator Ryan Dhal. He saw the problem with blocking on I/O and how JavaScript was the perfect language for getting mainstream programmers doing asynchronous programming on the server. His presentation was received with a standing ovation.


Node.js is one of the most popular projects on Github after beating out heavyweights such as Ruby on Rails and jQuery.


Cross Platform
Node.js is cross-platform. It can run on Windows, Unix, and Mac OS X.
NPM (Node Package Manager)
Automatically installed with Node
Commands: npm help
| install
| ls
| search
Packages can be installed locally or globally via -g (useful to use packages as cmdline tools)
Npm install will create a directory ./node_modules/
Nodejs Building Block
An event loop and a low level I/O API call Libuv, Google Chrome’s V8 and custom C++, and JavaScript code developed specifically for the Node.js platform itself. These three things makes up the Nodejs Platform.


Event Loop
The event loop is what allows Node.js to perform non-blocking I/O operation even though JavaScript is single threaded by offloading operations to the system kernel (the core of the OS). When one of these operations completes, the kernel tells Node.js that the appropriate callback may be added to the poll queue to eventually be executed.
When Node.js Starts
process.nextTick()
Event loop structure
Consists of phases. Each phase has a FIFO (first in first out) queue of callbacks to execute.
On each phase, Node.js runs any operations specific to that phase, then runs callbacks in that phase’s queue until empty queue or max num of callbacks are executed then moves to the next phase.
Since any operations may schedule more operations and new events processed in the poll phase are queued by the kernel, poll events can be queued while polling events are being processed.
Between each run of the event loop, Node.js checks if it is waiting for any asynchronous I/O or timers and shuts down cleanly if there are not any.
Timers:executes callbacks scheduled by setTimeout()
and setInterval()
. A timer specifies the threshold after which a provided callback may be executed rather than the exact time a person wants it to be run.
Pending I/O callbacks: executes almost all callbacks with exception of close callbacks, the ones scheduled by timers, and setImmediate
.
Idle, prepare:only used internally.
Poll:retrieve new I/O events; Node will block here when appropriate.
Two main functions:
- Executing scripts for timers whose threshold has elapsed, then
- Processing events in the poll queue.
Module and Packages
A module is any file or directory that can be loaded by Node.js’ using require()
.
A package is a file or directory described by a package.json
.
In the Node.js module system, each file is treated as a separate module
// mymodule.js
const anotherModule = require(‘anotherModule’)
console.log(module);
In each module, the module free variable is a reference to the object representing the current module.
When a file is run directly from Node.js, require.main
is set to its module. Meaning it is possible to determine whether a file has been run directly by testing require.main===module
.
For a file foo.js
, this will be true
if run via Node foo.js
, but false if run by require(‘./foo’)
because the module provides a filename property, the entry point of the current app can be obtained by checking require.main.filename
.
Core Modules
Core modules are compiled into Node binary distribution and loaded automatically when the Node process starts. Still, these need to be imported in order to be used in your application.
Some core modules: http, url, querystring, path, fs, util.
Node.js in Practice
JavaScript
Typing:dynamic, weak
Multi-paradigm:imperative, object-oriented, functional, event-driven.
Standard:ECMAScript — ES’2015 (ES6): classes, modules, generators, arrow functions, promises.
Influenced by: Java, C, Python.
Primitive types: numbers, strings, boolean, Null, Undefined.
Examples
var x = 10.7; // hoisted at global or function level
const y = “hello”; // block-level scoped
var arr = [1,2,3];
for(var i = 0; i<arr.length; i++) { console.log(arr[i]); }
Learning Path and Resources
To learn Node.js, you need 3 things.
- Knowledge of JavaScript
- Understanding of how server side works
- Node.js
Tutorials
· Learn Node.js — Best Node.js tutorials
· Learn Node JS in a Week via Video Screencast
· Thinkful Web Development Bootcamp || Node Skills Course
Books
· Smashing Node.js: JavaScript Everywher
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK