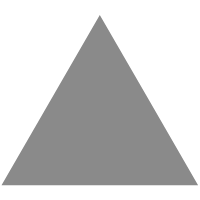
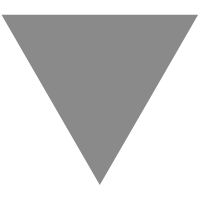
使用JavaScript的一些小技巧
source link: https://www.tuicool.com/articles/QZrMji7
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
任何一门技术在实际中都会有一些属于自己的小技巧。同样的,在使用JavaScript时也有一些自己的小技巧,只不过很多时候有可能容易被大家忽略。而在互联网上,时不时的有很多同行朋友会总结(或收集)一些这方面的小技巧。作为一位JavaScript的菜鸟级的同学,更应该要留意这些小技巧,因为这些小技巧可以在实际业务的开发中帮助我们解决问题,而且会很容易的解决问题。在这篇文章中,会整理一些大家熟悉或不熟悉的有关于JavaScript的小技巧。
数组
先来看使用数组中常用的一些小技巧。
数组去重
ES6提供了几种简洁的数组去重的方法,但该方法并不适合处理非基本类型的数组。对于基本类型的数组去重,可以使用 ... new Set()
来过滤掉数组中重复的值,创建一个只有唯一值的新数组。
const array = [1, 1, 2, 3, 5, 5, 1] const uniqueArray = [...new Set(array)]; console.log(uniqueArray); > Result:(4) [1, 2, 3, 5]
这是ES6中的新特性,在ES6之前,要实现同样的效果,我们需要使用更多的代码。该技巧适用于包含基本类型的数组: undefined
、 null
、 boolean
、 string
和 number
。如果数组中包含了一个 object
, function
或其他数组,那就需要使用另一种方法。
除了上面的方法之外,还可以使用 Array.from(new Set())
来实现:
const array = [1, 1, 2, 3, 5, 5, 1] Array.from(new Set(array)) > Result:(4) [1, 2, 3, 5]
另外,还可以使用 Array
的 .filter
及 indexOf()
来实现:
const array = [1, 1, 2, 3, 5, 5, 1] array.filter((arr, index) => array.indexOf(arr) === index) > Result:(4) [1, 2, 3, 5]
注意, indexOf()
方法将返回数组中第一个出现的数组项。这就是为什么我们可以在每次迭代中将 indexOf()
方法返回的索引与当索索引进行比较,以确定当前项是否重复。
确保数组的长度
在处理网格结构时,如果原始数据每行的长度不相等,就需要重新创建该数据。为了确保每行的数据长度相等,可以使用 Array.fill
来处理:
let array = Array(5).fill(''); console.log(array); > Result: (5) ["", "", "", "", ""]
数组映射
不使用 Array.map
来映射数组值的方法。
const array = [ { name: '大漠', email: '[email protected]' }, { name: 'Airen', email: '[email protected]' } ] const name = Array.from(array, ({ name }) => name) > Result: (2) ["大漠", "Airen"]
数组截断
如果你想从数组末尾删除值(删除数组中的最后一项),有比使用 splice()
更快的替代方法。
例如,你知道原始数组的大小,可以重新定义数组的 length
属性的值,就可以实现从数组末尾删除值:
let array = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9] console.log(array.length) > Result: 10 array.length = 4 console.log(array) > Result: (4) [0, 1, 2, 3]
这是一个特别简洁的解决方案。但是, slice()
方法运行更快,性能更好:
let array = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]; array = array.slice(0, 4); console.log(array); > Result: [0, 1, 2, 3]
过滤掉数组中的falsy值
如果你想过滤数组中的 falsy
值,比如 0
、 undefined
、 null
、 false
,那么可以通过 map
和 filter
方法实现:
const array = [0, 1, '0', '1', '大漠', 'w3cplus.com', undefined, true, false, null, 'undefined', 'null', NaN, 'NaN', '1' + 0] array.map(item => { return item }).filter(Boolean) > Result: (10) [1, "0", "1", "大漠", "w3cplus.com", true, "undefined", "null", "NaN", "10"]
获取数组的最后一项
数组的 slice()
取值为正值时,从数组的开始处截取数组的项,如果取值为负整数时,可以从数组末属开始获取数组项。
let array = [1, 2, 3, 4, 5, 6, 7] const firstArrayVal = array.slice(0, 1) > Result: [1] const lastArrayVal = array.slice(-1) > Result: [7] console.log(array.slice(1)) > Result: (6) [2, 3, 4, 5, 6, 7] console.log(array.slice(array.length)) > Result: []
正如上面示例所示,使用 array.slice(-1)
获取数组的最后一项,除此之外还可以使用下面的方式来获取数组的最后一项:
console.log(array.slice(array.length - 1)) > Result: [7]
过滤并排序字符串列表
你可能有一个很多名字组成的列表,需要过滤掉重复的名字并按字母表将其排序。
在我们的例子里准备用不同版本语言的JavaScript 保留字的列表,但是你能发现,有很多重复的关键字而且它们并没有按字母表顺序排列。所以这是一个完美的字符串列表(数组)来测试我们的JavaScript小知识。
var keywords = ['do', 'if', 'in', 'for', 'new', 'try', 'var', 'case', 'else', 'enum', 'null', 'this', 'true', 'void', 'with', 'break', 'catch', 'class', 'const', 'false', 'super', 'throw', 'while', 'delete', 'export', 'import', 'return', 'switch', 'typeof', 'default', 'extends', 'finally', 'continue', 'debugger', 'function', 'do', 'if', 'in', 'for', 'int', 'new', 'try', 'var', 'byte', 'case', 'char', 'else', 'enum', 'goto', 'long', 'null', 'this', 'true', 'void', 'with', 'break', 'catch', 'class', 'const', 'false', 'final', 'float', 'short', 'super', 'throw', 'while', 'delete', 'double', 'export', 'import', 'native', 'public', 'return', 'static', 'switch', 'throws', 'typeof', 'boolean', 'default', 'extends', 'finally', 'package', 'private', 'abstract', 'continue', 'debugger', 'function', 'volatile', 'interface', 'protected', 'transient', 'implements', 'instanceof', 'synchronized', 'do', 'if', 'in', 'for', 'let', 'new', 'try', 'var', 'case', 'else', 'enum', 'eval', 'null', 'this', 'true', 'void', 'with', 'break', 'catch', 'class', 'const', 'false', 'super', 'throw', 'while', 'yield', 'delete', 'export', 'import', 'public', 'return', 'static', 'switch', 'typeof', 'default', 'extends', 'finally', 'package', 'private', 'continue', 'debugger', 'function', 'arguments', 'interface', 'protected', 'implements', 'instanceof', 'do', 'if', 'in', 'for', 'let', 'new', 'try', 'var', 'case', 'else', 'enum', 'eval', 'null', 'this', 'true', 'void', 'with', 'await', 'break', 'catch', 'class', 'const', 'false', 'super', 'throw', 'while', 'yield', 'delete', 'export', 'import', 'public', 'return', 'static', 'switch', 'typeof', 'default', 'extends', 'finally', 'package', 'private', 'continue', 'debugger', 'function', 'arguments', 'interface', 'protected', 'implements', 'instanceof'];
因为我们不想改变我们的原始列表,所以我们准备用高阶函数叫做 filter
,它将基于我们传递的回调方法返回一个新的过滤后的数组。回调方法将比较当前关键字在原始列表里的索引和新列表中的索引,仅当索引匹配时将当前关键字push到新数组。
最后我们准备使用 sort
方法排序过滤后的列表,sort只接受一个比较方法作为参数,并返回按字母表排序后的列表。
在ES6下使用箭头函数看起来更简单:
const filteredAndSortedKeywords = keywords .filter((keyword, index) => keywords.lastIndexOf(keyword) === index) .sort((a, b) => a < b ? -1 : 1);
这是最后过滤和排序后的JavaScript保留字列表:
console.log(filteredAndSortedKeywords); > Result: ['abstract', 'arguments', 'await', 'boolean', 'break', 'byte', 'case', 'catch', 'char', 'class', 'const', 'continue', 'debugger', 'default', 'delete', 'do', 'double', 'else', 'enum', 'eval', 'export', 'extends', 'false', 'final', 'finally', 'float', 'for', 'function', 'goto', 'if', 'implements', 'import', 'in', 'instanceof', 'int', 'interface', 'let', 'long', 'native', 'new', 'null', 'package', 'private', 'protected', 'public', 'return', 'short', 'static', 'super', 'switch', 'synchronized', 'this', 'throw', 'throws', 'transient', 'true', 'try', 'typeof', 'var', 'void', 'volatile', 'while', 'with', 'yield']
清空数组
如果你定义了一个数组,然后你想清空它。 通常,你会这样做:
let array = [1, 2, 3, 4]; function emptyArray() { array = []; } emptyArray();
但是,这有一个效率更高的方法来清空数组。 你可以这样写:
let array = [1, 2, 3, 4]; function emptyArray() { array.length = 0; } emptyArray();
拍平多维数组
使用 ...
运算符,将多维数组拍平:
const arr = [1, [2, '大漠'], 3, ['blog', '1', 2, 3]] const flatArray = [].concat(...arr) console.log(flatArray) > Result: (8) [1, 2, "大漠", 3, "blog", "1", 2, 3]
不过上面的方法只适用于二维数组。不过通过递归调用,可以使用它适用于二维以下的数组:
function flattenArray(arr) { const flattened = [].concat(...arr); return flattened.some(item => Array.isArray(item)) ? flattenArray(flattened) : flattened; } const array = [1, [2, '大漠'], 3, [['blog', '1'], 2, 3]] const flatArr = flattenArray(array) console.log(flatArr) > Result: (8) [1, 2, "大漠", 3, "blog", "1", 2, 3]
从数组中获取最大值和最小值
可以使用 Math.max
和 Math.min
取出数组中的最大小值和最小值:
const numbers = [15, 80, -9, 90, -99] const maxInNumbers = Math.max.apply(Math, numbers) const minInNumbers = Math.min.apply(Math, numbers) console.log(maxInNumbers) > Result: 90 console.log(minInNumbers) > Result: -99
另外还可以使用ES6的 ...
运算符来完成:
const numbers = [1, 2, 3, 4]; Math.max(...numbers) > Result: 4 Math.min(...numbers) > > Result: 1
对象
在操作对象时也有一些小技巧。
使用 ...
运算符合并对象或数组中的对象
同样使用ES的 ...
运算符可以替代人工操作,合并对象或者合并数组中的对象。
// 合并对象 const obj1 = { name: '大漠', url: 'w3cplus.com' } const obj2 = { name: 'airen', age: 30 } const mergingObj = {...obj1, ...obj2} > Result: {name: "airen", url: "w3cplus.com", age: 30} // 合并数组中的对象 const array = [ { name: '大漠', email: '[email protected]' }, { name: 'Airen', email: '[email protected]' } ] const result = array.reduce((accumulator, item) => { return { ...accumulator, [item.name]: item.email } }, {}) > Result: {大漠: "[email protected]", Airen: "[email protected]"}
有条件的添加对象属性
不再需要根据一个条件创建两个不同的对象,以使它具有特定的属性。为此,使用 ...
操作符是最简单的。
const getUser = (emailIncluded) => { return { name: '大漠', blog: 'w3cplus', ...emailIncluded && {email: '[email protected]'} } } const user = getUser(true) console.log(user) > Result: {name: "大漠", blog: "w3cplus", email: "[email protected]"} const userWithoutEmail = getUser(false) console.log(userWithoutEmail) > Result: {name: "大漠", blog: "w3cplus"}
解构原始数据
你可以在使用数据的时候,把所有数据都放在一个对象中。同时想在这个数据对象中获取自己想要的数据。在这里可以使用ES6的Destructuring特性来实现。比如你想把下面这个 obj
中的数据分成两个部分:
const obj = { name: '大漠', blog: 'w3cplus', email: '[email protected]', joined: '2019-06-19', followers: 45 } let user = {}, userDetails = {} ({name: user.name, email: user.email, ...userDetails} = obj) > {name: "大漠", blog: "w3cplus", email: "[email protected]", joined: "2019-06-19", followers: 45} console.log(user) > Result: {name: "大漠", email: "[email protected]"} console.log(userDetails) > Result: {blog: "w3cplus", joined: "2019-06-19", followers: 45}
动态更改对象的key
在过去,我们首先必须声明一个对象,然后在需要动态属性名的情况下分配一个属性。在以前,这是不可能以声明的方式实现的。不过在ES6中,我们可以实现:
const dynamicKey = 'email' let obj = { name: '大漠', blog: 'w3cplus', [dynamicKey]: '[email protected]' } console.log(obj) > Result: {name: "大漠", blog: "w3cplus", email: "[email protected]"}
判断对象的数据类型
使用 Object.prototype.toString
配合闭包来实现对象数据类型的判断:
const isType = type => target => `[object ${type}]` === Object.prototype.toString.call(target) const isArray = isType('Array')([1, 2, 3]) console.log(isArray) > Result: true
上面的代码相当于:
function isType(type){ return function (target) { return `[object ${type}]` === Object.prototype.toString.call(target) } } isType('Array')([1,2,3]) > Result: true
或者:
const isType = type => target => `[object ${type}]` === Object.prototype.toString.call(target) const isString = isType('String') const res = isString(('1')) console.log(res) > Result: true
检查某对象是否有某属性
当你需要检查某属性是否存在于一个对象,你可能会这样做:
var obj = { name: '大漠' }; if (obj.name) { console.log(true) // > Result: true }
这是可以的,但是你需要知道有两种原生方法可以解决此类问题。 in
操作符 和 Object.hasOwnProperty
,任何继承自 Object
的对象都可以使用这两种方法。
var obj = { name: '大漠' }; obj.hasOwnProperty('name'); // > true 'name' in obj; // > true obj.hasOwnProperty('valueOf'); // > false, valueOf 继承自原型链 'valueOf' in obj; // > true
两者检查属性的深度不同,换言之 hasOwnProperty
只在本身有此属性时返回 true
,而 in
操作符不区分属性来自于本身或继承自原型链。
这是另一个例子:
var myFunc = function() { this.name = '大漠'; }; myFunc.prototype.age = '10 days'; var user = new myFunc(); user.hasOwnProperty('name'); > Result: true user.hasOwnProperty('age'); > Result: false, 因为age来自于原型链
创造一个纯对象
使用 Object.create(null)
可以创建一个纯对象,它不会从 Object
类继承任何方法(例如:构造函数、 toString()
等):
const pureObject = Object.create(null); console.log(pureObject); //=> {} console.log(pureObject.constructor); //=> undefined console.log(pureObject.toString); //=> undefined console.log(pureObject.hasOwnProperty); //=> undefined
数据类型转换
JavaScript中数据类型有 Number
、 String
、 Boolean
、 Object
、 Array
和 Function
等,在实际使用时会碰到数据类型的转换。在转换数据类型时也有一些小技巧。
转换为布尔值
布尔值除了 true
和 false
之外,JavaScript还可以将所有其他值视为“ 真实的
”或“ 虚假的
”。除非另有定义,JavaScript中除了 0
、 ''
、 null
、 undefined
、 NaN
和 false
之外的值都是 真实的
。
我们可以很容易地在真和假之间使用 !
运算符进行切换,它也会将类型转换为 Boolean
。比如:
const isTrue = !0; const isFasle = !1; const isFasle = !!0 // !0 => true,true的反即是false console.log(isTrue) > Result: true console.log(typeof isTrue) > Result: 'boolean'
这种类型的转换在条件语句中非常方便,比如将 !1
当作 false
。
转换为字符串
我们可以使用运算符 +
后紧跟一组空的引号 ''
快速地将数字或布尔值转为字符串:
const val = 1 + '' const val2 = false + '' console.log(val) > Result: "1" console.log(typeof val) > Result: "string" console.log(val2) > Result: "false" console.log(typeof val2) > Result: "string"
转换为数值
上面我们看到了,使用 +
紧跟一个空的字符串 ''
就可以将数值转换为字符串。相反的,使用加法运算符 +
可以快速实现相反的效果。
let int = '12' int = +int console.log(int) > Result: 12 console.log(typeof int) > Result: 'number'
用同样的方法可以将布尔值转换为数值:
console.log(+true) > Return: 1 console.log(+false) > Return: 0
在某些上下文中, +
会被解释为 连接操作符
,而不是 加法
运算符。当这种情况发生时,希望返回一个整数,而不是浮点数,那么可以使用两个波浪号 ~~
。双波浪号 ~~
被称为 按位不运算符
,它和 -n - 1
等价。例如, ~15 = -16
。这是因为 - (-n - 1) - 1 = n + 1 - 1 = n
。换句话说, ~ - 16 = 15
。
我们也可以使用 ~~
将数字字符串转换成整数型:
const int = ~~'15' console.log(int) > Result: 15 console.log(typeof int) > Result: 'number'
同样的, NOT
操作符也可以用于布尔值: ~true = -2
, ~false = -1
。
浮点数转换为整数
平常都会使用 Math.floor()
、 Math.ceil()
或 Math.round()
将浮点数转换为整数。在JavaScript中还有一种更快的方法,即使用 |
(位或运算符)将浮点数截断为整数。
console.log(23.9 | 0); > Result: 23 console.log(-23.9 | 0); > Result: -23
|
的行为取决于处理的是正数还是负数,所以最好只在确定的情况下使用这个快捷方式。
如果 n
是正数,则 n | 0
有效地向下舍入。如果 n
是负数,它有效地四舍五入。更准确的说,该操作删除小数点后的内容,将浮点数截断为整数。还可以使用 ~~
来获得相同的舍入效果,如上所述,实际上任何位操作符都会强制浮点数为整数。这些特殊操作之所以有效,是因为一旦强制为整数,值就保持不变。
|
还可以用于从整数的末尾删除任意数量的数字。这意味着我们不需要像下面这样来转换类型:
let str = "1553"; Number(str.substring(0, str.length - 1)); > Result: 155
我们可以像下面这样使用 |
运算符来替代:
console.log(1553 / 10 | 0) > Result: 155 console.log(1553 / 100 | 0) > Result: 15 console.log(1553 / 1000 | 0) > Result: 1
使用 !!
操作符转换布尔值
有时候我们需要对一个变量查检其是否存在或者检查值是否有一个有效值,如果存在就返回 true
值。为了做这样的验证,我们可以使用 !!
操作符来实现是非常的方便与简单。对于变量可以使用 !!variable
做检测,只要变量的值为: 0
、 null
、 " "
、 undefined
或者 NaN
都将返回的是 false
,反之返回的是 true
。比如下面的示例:
function Account(cash) { this.cash = cash; this.hasMoney = !!cash; } var account = new Account(100.50); console.log(account.cash); > Result: 100.50 console.log(account.hasMoney); > Result: true var emptyAccount = new Account(0); console.log(emptyAccount.cash); > Result: 0 console.log(emptyAccount.hasMoney); > Result: false
在这个示例中,只要 account.cash
的值大于 0
,那么 account.hasMoney
返回的值就是 true
。
还可以使用 !!
操作符将 truthy
或 falsy
值转换为布尔值:
!!"" // > false !!0 // > false !!null // > false !!undefined // > false !!NaN // > false !!"hello" // > true !!1 // > true !!{} // > true !![] // > true
小结
文章主要收集和整理了一些有关于JavaScript使用的小技巧。既然是技巧在必要的时候能帮助我们快速的解决一些问题。如果你有这方面的相关积累,欢迎在下面的评论中与我们一起分享。后续将会持续更新,希望对大家有所帮助。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK