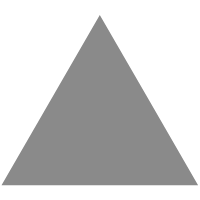
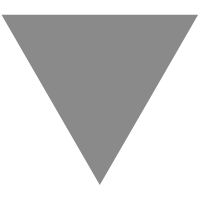
Functional Interface and Lambda Expressions in Java 8
source link: https://www.tuicool.com/articles/hit/Y3Yf6fJ
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Functional Interface
A functional interface is an interface with only one abstract method. This means that the interface implementation will only represent one behavior. Examples of a functional interface in Java are:
-
java.lang.Runnable
-
java.util.Comparator
-
java.util.concurrent.Callable
-
java.io.FileFilter
An important point to remember is that the functional interface can have a number of default methods but only one abstract method.
Lambda Expressions
Lambda expressions are introduced in Java 8, and they can represent the instance of a functional interface.
To explain the above statement, we are defining a functional interface named "SquareRoot." It has only one abstract method, "findSquareRoot." @FunctionalInterface
annotations are used to declare an interface as a functional interface.
@FunctionalInterface public interface SquareRoot { abstract double findSquareRoot(int n); }
Before Java 8, we could do the following to use the SquareRoot interface:
-
Create an implementation class called
SquareRootImpl
and implement thefindSquareRoot
method and use the instance ofSquareRootImpl
to find the square root of input values.
class SquareRootImpl implements SquareRoot { @Override public double findSquareRoot(int n) { return Math.sqrt(n); } }
Usage of the above implementation can be done below:
SquareRootImpl squareRootImpl = new SquareRootImpl(); squareRootImpl.findSquareRoot(in);
2. Create an anonymous implementation of the SquareRoot interface and use it to find the square root value.
SquareRoot squareRoot = new SquareRoot() { @Override public double findSquareRoot(int n) { return Math.sqrt(n); } };
Here is the usage:
squareRoot.findSquareRoot(in);
The above approaches are very verbose and have a boilerplate code. The same can be done using the lambda expression in fewer lines of code
SquareRoot squareRoot = (n) -> (Math.sqrt(n)); squareRoot.findSquareRoot(in);
squareRoot
is instance of the functional interface "SquareRoot." The interface has only one abstract method and the (n) -> (Math.sqrt(n))
expression provides the implementation of the abstract method findSquareRoot
.
Explained: (n) -> (Math.sqrt(n))
In this example method, findSquareRoot
expects one input of type double.
The left side of the expression is (n), "n" represents the value expected by the method findSquareRoot
.
The right side of the expression followed by the ->
sign is (Math.sqrt(n))
.
This is the actual method code, using the Math.sqrt
function to find the squareRoot
.
In the example of the abstract function, findSquareRoot
accepts one input of the type int
and returns value of type double.
Abstract method: abstract double findSquareRoot(int n)
;
Lambda expression: SquareRoot squareRoot = (n) -> (Math.sqrt(n))
"n" is the input and Math.srqrt(n)
is the output. The input and output type should match the abstract method in the interface.
Block Lambda Expression
Lambda expressions can have multiple lines; the following is the syntax:
SquareRoot squareRoot = (n) -> { double result = Math.sqrt(n); return result; };
Here is an example of the usage:
squareRoot.findSquareRoot(in);
Lambda Expressions as an Argument to Function
You can pass the squareRoot
as input to any function. This is done the way we could pass an implementation instance of an interface in earlier versions of Java.
private static double squareRootJava8LambdaAsArgument(int i) { SquareRoot squareRoot = (n) -> (Math.sqrt(n)); return displayData(squareRoot, i); } private static double displayData(SquareRoot squareRoot, int i) { return squareRoot.findSquareRoot(9); }
In the above example, the square root is passed as an input to the method displayData
.
Lambda Expression With the Type of Input Syntax
We can use the type on input in syntax, e.g. int n,
in this case.
SquareRoot squareRoot = (int n) -> (Math.sqrt(n));
Lambda Expression With No Input
A function can have no input, which can be seen below:
Abstarct method in functionalInterface abstract double sayHello(); Lambda expression for abstract method will look like () -> (System.out.println(“Hellow”))
The () represents an empty input, and the response is String Hellow
.
Lambda Expression With More Than One Input
The abstract method sum takes two inputs of type int
.
abstract method sum in functional interface abstract int sum(int a, int b); Lambda expression for abstract method will look like (a,b) -> (a+b) OR (int a,int b) -> (a+b)
The detailed code can be found here .
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK