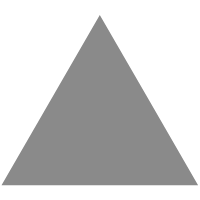
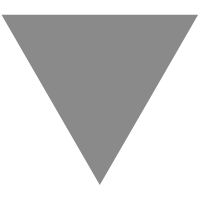
GitHub - sindresorhus/Defaults: Swifty and modern UserDefaults
source link: https://github.com/sindresorhus/Defaults
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
readme.md
Defaults 
Swifty and modern UserDefaults
Highlights
- Strongly typed: You declare the type and default value upfront.
- Codable support: You can store any Codable value, like an enum.
- Debuggable: The data is stored as JSON-serialized values.
- Lightweight: It's only ~100 lines of code.
Requirements
- macOS 10.12+
- Xcode 9.3+
- Swift 4.1+
Install
With SPM:
.package(url: "https://github.com/sindresorhus/Defaults", from: "0.1.0")
With Carthage:
github "sindresorhus/Defaults"
Usage
You declare the defaults keys upfront with type and default value.
import Cocoa import Defaults extension Defaults.Keys { static let quality = Defaults.Key<Double>("quality", default: 0.8) // ^ ^ ^ ^ // Key Type UserDefaults name Default value }
You can then access it as a subscript on the defaults
global (note lowercase):
defaults[.quality] //=> 0.8 defaults[.quality] = 0.5 //=> 0.5 defaults[.quality] += 0.1 //=> 0.6 defaults[.quality] = "?" //=> [Cannot assign value of type 'String' to type 'Double']
You can also declare optional keys for when you don't want to declare a default value upfront:
extension Defaults.Keys { static let name = Defaults.OptionalKey<Double>("name") } if let name = defaults[.name] { print(name) }
Enum example
enum DurationKeys: String, Codable { case tenMinutes = "10 Minutes" case halfHour = "30 Minutes" case oneHour = "1 Hour" } extension Defaults.Keys { static let defaultDuration = Defaults.Key<DurationKeys>("defaultDuration", default: .oneHour) } defaults[.defaultDuration].rawValue //=> "1 Hour"
It's just UserDefaults with sugar
This works too:
extension Defaults.Keys { static let isUnicorn = Defaults.Key<Bool>("isUnicorn", default: true) } UserDefaults.standard[.isUnicorn] //=> true
Shared UserDefaults
extension Defaults.Keys { static let isUnicorn = Defaults.Key<Bool>("isUnicorn", default: true) } let extensionDefaults = UserDefaults(suiteName: "com.unicorn.app")! extensionDefaults[.isUnicorn] //=> true
API
let defaults = Defaults()
Defaults.Keys
Type: class
Stores the keys.
Defaults.Key
Type: class
Create a key with a default value.
Defaults.OptionalKey
Type: class
Create a key with an optional value.
defaults.clear()
Type: func
Clear the user defaults.
FAQ
How is this different from SwiftyUserDefaults
?
It's inspired by it and other solutions. The main difference is that this module doesn't hardcode the default values and comes with Codable support.
Related
- LaunchAtLogin - Add "Launch at Login" functionality to your macOS app
- DockProgress - Show progress in your app's Dock icon
- Gifski - Convert videos to high-quality GIFs on your Mac
License
MIT © Sindre Sorhus
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK