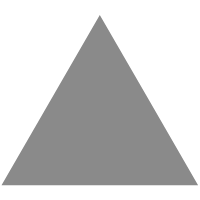
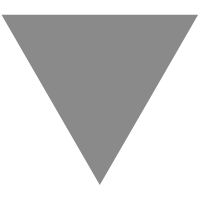
5 practical tips to finally learn React in 2018 - Gosha Arinich
source link: https://goshakkk.name/tips-finally-learn-react/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
5 practical tips to finally learn React in 2018
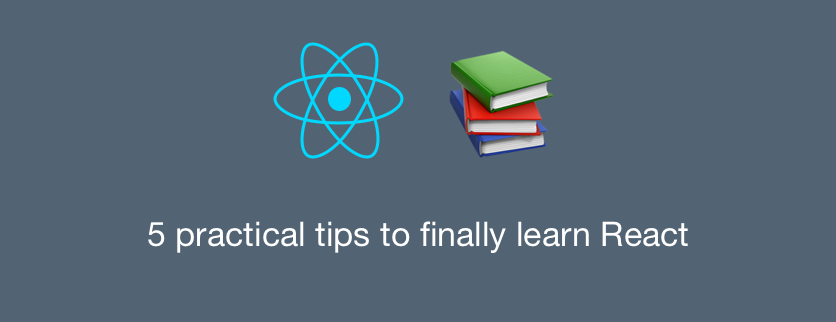
I get asked something along the lines of the following pretty often:
Job needs me to know React like yesterday... I've literally never done React, or even read about it, until three days ago.
If you feel like this too, I'm here to help.
I've learned React (as many other things) the hard way: I got my feet wet, experimented a lot, read a lot, sometimes spent weeks without much progress while other times I could have had a dozen a-ha moments in a single evening. My learning was pretty much self-guided, with no one to show me a simpler (or a better) way.
My hope with this article — and my blog and mailing list in general — is to share what I've learned in years so that you don't have to waste time.
Bear in mind that this advice relates to learning React fundamentals. These are not hard-and-fast truths that you have to abide by till the end of times. Instead, these are just some helpful guidelines to help you learn React fundamentals faster — so you can move on to the other things.
1. Skip all the buzzwords (for now)
You are bound to come across people saying you have to know all these mysterious things. Just seeing a long list of alien words is depressing enough.
So skip these — at first. I'm not saying you should never bother to read about these, they all have their uses. I'm just saying that, at this point, you're better off without them.
You will catch up later, when you have a solid understanding of React fundamentals.
For example, and this list is not exhaustive:
- You do not have to be a Webpack pro — use create-react-app.
- You do not have to use Redux or MobX — master the state mechanism that React provides. Often, it's all you need.
- You do not have to use GraphQL, Apollo, Relay.
- You do not have to use React-Router — you can do a simple conditional (more on that in a bit).
2. Don't fetch data yet — hardcode it
Data is the blood of applications, yes. And it usually lives on the server, which means you're going to ask one (or several) of these:
- "How do I retrieve data from MySQL in React?"
- "Do I have to write my server now?"
- "Fetch vs. axios?"
These are all valid questions to ask... once you know React fundamentals well. Until then, these are just going to get in your way.
What I propose instead is: hardcode some fake data, all in one place.
There's nothing wrong with the below:
var data = {
products: [
{ id: 1, title: 'Consulting' },
{ id: 2, title: 'TMFHR' },
],
};
// then just use `data`
const HomePage = () => (
<div>
{data.products.map(product => (
<p>{product.title}</p>
))}
</div>
);
Think about fetch
ing it later!
3. Make just one page
Come up with a learning project if you don't have one yet.
Don't overthink the project at a whole. Pick one page — any page — and make it into a real component. No excuses, no delays. One page is doable.
Here, let me help get you started:
class PageA extends Component {
render() {
return (
<div>Do things</div>
);
}
}
ReactDOM.render(<PageA />, document.body);
"But it needs some data from the server..." See the previous section, use fake data.
Once you have one page, make another. And another.
You can just change the ReactDOM.render
call to show a different page.
It's not perfect — one page at a time with no way to switch — but it lets you get started fast.
Speaking of switching pages...
4. The simplest, no-dependency router is state
"How am I going to move between pages without React-Router?"
The router itself is just a glorified conditional statement that decides which page component to render.
class App extends Component {
render() {
const currentPage = 'a';
if (currentPage === 'a') {
return <PageA />;
} else if (currentPage === 'b') {
return <PageB />;
} else {
return <div>Something went wrong</div>
}
}
}
Storing the current page name in a local variable is not ideal as we can't change it... so we can store it in the state instead:
class App extends Component {
state = {
currentPage: 'a',
};
render() {
const { currentPage } = this.state;
if (currentPage === 'a') {
return <PageA />;
} else if (currentPage === 'b') {
return <PageB />;
} else {
return <div>Something went wrong</div>
}
}
}
And since PageA
might want to go to PageB
, we can pass each page a function to switch to another page:
class App extends Component {
state = {
currentPage: 'a',
};
goTo = (pageName) => this.setState({ currentPage: pageName });
render() {
const { currentPage } = this.state;
if (currentPage === 'a') {
return <PageA goTo={this.goTo} />;
} else if (currentPage === 'b') {
return <PageB goTo={this.goTo} />;
} else {
return <div>Something went wrong</div>
}
}
}
const PageA = ({ goTo }) => (
<div>
<h2>Page A</h2>
<a onClick={() => goTo('b')}>Page B</a>
</div>
);
This approach has a lot of shortcomings when it comes to production use... but when you're only starting to learn React, it can be a viable router.
(I've written a separate post about this, if you are interested: Simplest, no-dependency router is... state
5. What's next
Before proceeding any further, you should be comfortable with React as it is. That means having a feel for splitting things into components, using state and props to drive your little application, and so on.
If you're not there yet, pause. Give yourself the time (& practice!) to get there.
After that, there are many ways you can go further, some of which are:
- Learn to use a proper router.
- Do a level-up and make your fake data "async."
- Grow your app to the point when React state alone is not enough (and try Redux or MobX, or both!)
See also
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK