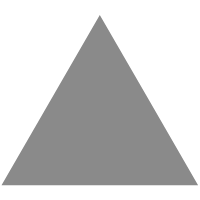
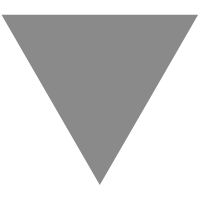
Optionalize your getters with help from Intellij IDEA custom inspections
source link: https://medium.com/@dannyb7878/optionalize-your-getters-with-help-from-intellij-idea-custom-inspections-299bdc6c4361
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Optionalize your getters with help from Intellij IDEA custom inspections
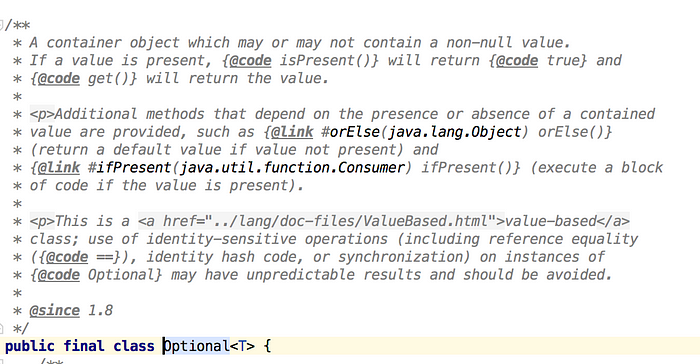
In Java 8, we saw Optional coming into our life. As opposed to the Stream API, I think this is somewhat underused feature.
One of its great uses is in your data classes, instead of your simple getters you can wrap it Optional and have better null safety.
I will try to demonstrate it with an example. Suppose you have a data class such as MySuperCoolDataObject, with nothing more than a simple getter:
public class MySuperCoolDataObject {
private String myString;
public String getMyString() {
return myString;
}
}
Could myString be null? Maybe, the point that you don’t know. That object might be serialized from some JSON payload or created in different part of the system. So, you probably will have the following code, or some variant of it, everywhere with you use it.
String myString = coolDataObject.getMyString();
if (myString != null) {
// only now do something with it
}
It could happen when you first write this code, it could happen after your first null pointer, or it might useless check as this field can never null.
In the first two cases, where this field is nullable, what if the creator of the data class was kind enough to state the intention clearly?
This is were Optional can help us, and produce the following code:
public class MySuperCoolDataObject {
private String myString;
public Optional<String> getMyString() {
return Optional.ofNullable(myString);
}
}
Here you can’t forget treating the option that this getter can return null. You have one less of the dreaded NullPointerException to worry about. And you can enjoy all the goodies that Optional provides you to write better code.
But writing this new getter method takes some time and can be annoying after doing it multiple times. Creating getter is simple command in any Java IDE. What if we create the optionalized getter in more simple way?
Before showing the custom inspection way, I would like to note that you can use the native Intellij IDEA powers and change the getter to return Optional of the type. Using the show intention shortcut Intellij kindly offers us to wrap the return value using Optional:
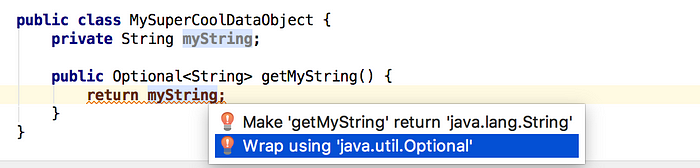
And you will get the same result as the optionalized data class.
So let me now show you to the custom inspection way!
One of the many tools Intellij IDEA offers you is the structual replace. It can be used in many ways to improve our code, and the speed of writing. So let’s see how we can use it in our advantage for creating the optionalized getter.
First open the inspection dialog box:

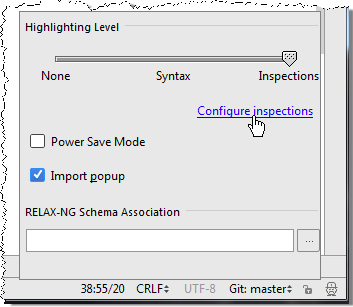
In the resulting dialog, search for Structural search inspection
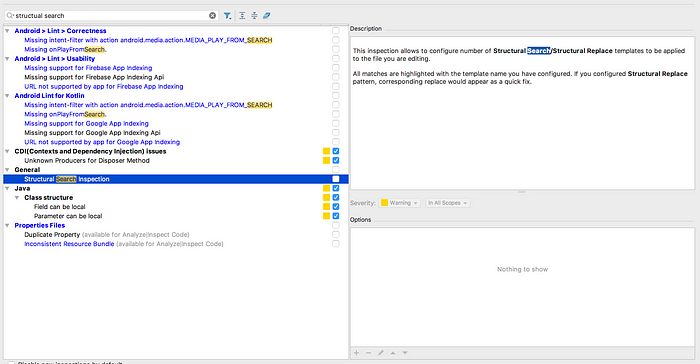
Enable it, and add a new replace template:
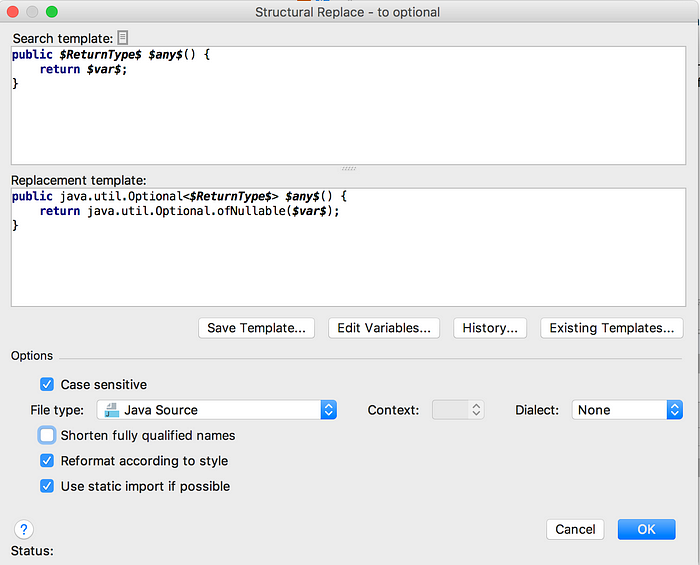
Remember to change the severity to the one you think best (probably not the default one: Warnings)
And now you can enjoy it:

Remember to change the severity to the one you think best (probably not the default one: Warnings)
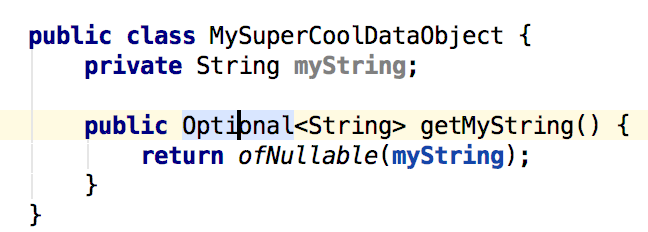
After applying the fix
So enjoy your new powers to write a better code and faster!
Some notes:
- As with everything, use Optional with care. It can be sometimes annoying to work with.
- If you already know the custom inspection tool, I would love you to share your experiences with using this tool.
Further reads:
[1] https://www.jetbrains.com/help/idea/creating-custom-inspections.html
Creating custom insepction — the jetbrains guide
[2] https://www.youtube.com/watch?time_continue=3339&v=Ej0sss6cq14
Great talk about how using Optional correctly and it’s powers.
[3] http://www.oracle.com/technetwork/articles/java/java8-optional-2175753.html
[4] https://blog.jetbrains.com/idea/2017/08/code-smells-null/
Two great articles on Optionals.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK