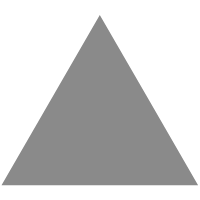
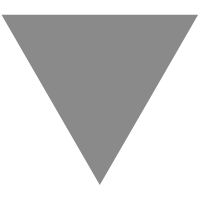
An interesting programming feature that almost no language has
source link: https://medium.com/@kasperpeulen/an-interesting-programming-feature-that-no-language-has-except-php-4de22f9e3964
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
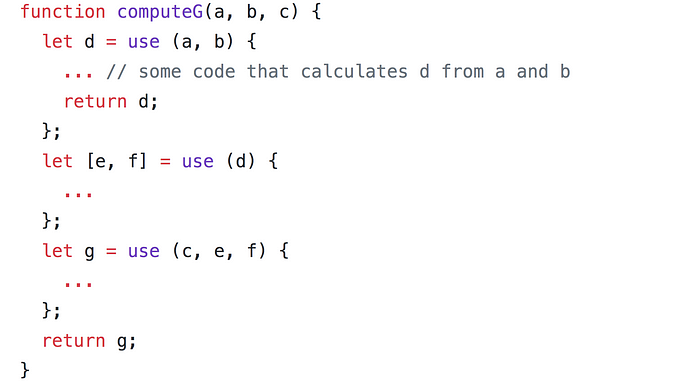
An interesting programming feature that almost no language has
Some time ago I was watching a video from Brian Will. It’s quite a long video and very controversial, but at the end he proposes a programming language feature that has been in the back of my head for a long time, and I would like to discuss it in this blog.
The feature allows to write self invoked anonymous functions that don’t let any scope from the outer function leak in. The syntax he proposes involves the use keyword, followed by explicit parameters, which is followed by a block that can use those parameters and return a value.
It would look like this in Javascript:
The following example shows how you can partially mimic this in Javascript using anonymous inline functions. The difference is that all the scope of the outer function is available in the inner function, which wouldn’t be available in the use block.
We could simplify this actually a bit, by not writing any arguments to the self invoked anonymous functions, because it doesn’t really matter. In Javascript all the scope from the outer functions will leak into the inner function. And as far as I know, this is true for most programming languages.
Now in many cases this is useful, for example, methods like map, filter and reduce from Array accept a function, and in that case, you want the scope of the outer function to be available.
So, why would this use block be useful? Why would I structure my program like I wrote above? The idea here is that I have structured my function into logical independent parts. The first part uses the variable a and b to compute the variable c, the next part uses d to compute e and f, and then c, e and f is used to compute our return value g.
The use keyword guarantees that those part are independent of each other, except for the parameters they use and the return values they give. Every part acts like a (possibly pure) function by itself. Now in a language that doesn’t has this feature, I would write the code above like this:
I think this style of writing a lot of shorter functions (or methods) instead of one very long function (as in the C-days) is getting more and more popular and accepted. It has a lot of benefits, and makes even more sense if you need to reuse computeD, computeEandF and computeG somewhere else. But if you don’t need to reuse it, I think that the proposed use block give even more benefits.
The benefits of the use block over writing functions would be:
- It is now easier to follow the chronology of the code. Now, the implementation of the function isn’t split up in different places in our code base. The inner functions may now be defined as top level functions in different files or maybe as methods in (different) classes.
- We now don’t have to make good names for all those functions, which is sometimes stated as being the hardest part of programming (we can still leave a comment if that is helpful).
You also get similar benefits as when we would have written functions:
- Any of the outer scope can not leak into the inner scope, except if you use explicit parameters. You are explicit in what the use block need to do his job.
- Any of the inner scope can not leak in the outer scope. Any variables that you declare in the use block, won’t be available in the outer function. The use block has no side effects for the rest of the function.
Now, if you later realise that you do want to reuse this code somewhere else, it now becomes trivial to make a top level function from this use block. Any editor could easily provide a refactor option for achieving this quickly.
What Brian Will didn’t realise that there are some languages that have this feature. C++ has this feature with a different syntax, Rust inline functions come very close and in PHP every anonymous function works this way. For example in PHP the above code would look like this:
I believe that this would be a truly great feature to have in any language, and I think this makes it easier to structure a long function in logical independent parts and would lead to less over abstraction. Many programmers follow the DRY principle by heart, which means that you should abstract if you are repeating yourself. I think this rule should go both ways:
Abstract if (and only if) you repeat yourself.
Of course, rules like this are rarely as general in practice as they are stated in theory, but I do think this feature helps to avoid abstractions if they are not needed. Happy coding :)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK