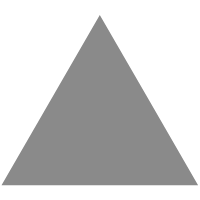
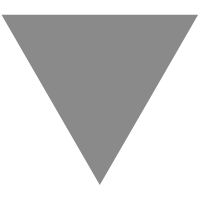
Microsoft Teams for Developers: Enhancing Communication With Call Initiating and...
source link: https://dzone.com/articles/microsoft-teams-for-developers-enhancing-communica
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Microsoft Teams for Developers: Enhancing Communication With Call Initiating and Recording
Learn how Microsoft Teams empowers developers to create seamless communication with smart calling solutions, APIs, and third-party integrations.
CORE ·
In today's fast-paced business environment, effective communication is the key to success. As remote work becomes more prevalent, organizations are increasingly relying on digital tools to support collaboration and productivity. Microsoft Teams is a leading platform that offers a wide range of features to facilitate seamless communication among team members.
In this article, we will delve into smart calling solutions with Microsoft Teams from a developer's perspective, exploring various APIs, integration options, and Java code samples for initiating and recording calls to enhance the user experience.
Overview of Microsoft Teams Calling Capabilities
Microsoft Teams provides a comprehensive suite of calling capabilities that allow users to make and receive calls, manage contacts, access voicemail, and more. Some of these features include:
- Direct routing: Enables organizations to bring their own telephone network to Microsoft Teams, allowing users to make and receive PSTN (Public Switched Telephone Network) calls
- Call queues: Allows organizations to create call queues to handle incoming calls, distribute them, and manage agent availability
- Auto attendant: Provides an automated system to answer and route incoming calls based on predefined rules
- Call analytics: Offers insights into call quality and performance for troubleshooting and optimization purposes
- Call recording: Allows organizations to record calls for compliance, training, or quality assurance purposes
Developers play a crucial role in leveraging these features and integrating them with existing systems to create a unified communication solution. Let's explore some of the tools and APIs available for developers to build smart calling solutions with Microsoft Teams.
Microsoft Graph API for Calling
The Microsoft Graph API is a powerful tool that enables developers to access and manipulate data within Microsoft 365 services, including Teams. By using the Graph API for calling, developers can perform various tasks such as initiating calls, managing call records, retrieving call details, and recording calls. Some of the key functionalities provided by the Graph API for calling include:
- Initiating calls: Developers can use the Graph API to initiate one-on-one or group calls, as well as create meetings with audio and video capabilities.
- Call controls: The API enables developers to manage calls by performing actions such as muting, transferring, or ending calls.
- Call records: Developers can access call records to retrieve information about call participants, duration, and other relevant details.
- Call recording: The API allows developers to start, stop, and download call recordings.
Java Code Sample: Initiating a Call With Microsoft Graph API
To initiate a call using the Microsoft Graph API, you'll need to set up the necessary authentication and make an HTTP POST
request to the /communications/calls
endpoint. Here's a Java code sample using the Microsoft Graph SDK to initiate a call:
import com.microsoft.graph.authentication.IAuthenticationProvider;
import com.microsoft.graph.authentication.TokenCredentialAuthProvider;
import com.microsoft.graph.models.*;
import com.microsoft.graph.requests.GraphServiceClient;
import java.util.Arrays;
public class TeamsCallInitiator {
public static void main(String[] args) {
String accessToken = "your_access_token_here"; // Replace with your access token
IAuthenticationProvider authProvider = new TokenCredentialAuthProvider(Arrays.asList("https://graph.microsoft.com/.default"), () -> accessToken);
GraphServiceClient graphClient = GraphServiceClient.builder().authenticationProvider(authProvider).buildClient();
Call call = new Call();
call.target = new InvitationParticipantInfo();
call.target.identity = new IdentitySet();
call.target.identity.user = new Identity();
call.target.identity.user.id = "user_id_here"; // Replace with the user's ID
call.mediaConfig = new ServiceHostedMediaConfig();
call.requestedModalities = Arrays.asList(Modality.AUDIO, Modality.VIDEO);
Call newCall = graphClient.communications().calls()
.buildRequest()
.post(call);
System.out.println("Call initiated: " + newCall.id);
}
}
Replace the your_access_token_here
and user_id_here
placeholders with your access token and the user's ID, respectively.
Java Code Sample: Recording a Call With Microsoft Graph API
To record a call using the Microsoft Graph API, you'll need to make an HTTP POST
request to the `/communications/calls/{id}/record`
endpoint, where {id}
is the call ID. Here's a Java code sample using the Microsoft Graph SDK to start recording a call:
import com.microsoft.graph.authentication.IAuthenticationProvider;
import com.microsoft.graph.authentication.TokenCredentialAuthProvider;
import com.microsoft.graph.models.*;
import com.microsoft.graph.requests.GraphServiceClient;
import java.util.Arrays;
public class TeamsCallRecorder {
public static void main(String[] args) {
String accessToken = "your_access_token_here"; // Replace with your access token
String callId = "call_id_here"; // Replace with the call ID
startRecording(accessToken, callId);
}
public static void startRecording(String accessToken, String callId) {
IAuthenticationProvider authProvider = new TokenCredentialAuthProvider(Arrays.asList("https://graph.microsoft.com/.default"), () -> accessToken);
GraphServiceClient graphClient = GraphServiceClient.builder().authenticationProvider(authProvider).buildClient();
RecordOperation recordOperation = new RecordOperation();
recordOperation.status = OperationStatus.NOT_STARTED;
RecordOperation startedRecording = graphClient.communications().calls(callId).record()
.buildRequest()
.post(recordOperation);
System.out.println("Recording started: " + startedRecording.id);
}
}
Replace the your_access_token_here
and call_id_here
placeholders with your access token and the call ID, respectively.
Integration With Third-Party Services
Integrating Microsoft Teams with third-party services can significantly enhance the calling experience for users. Some popular integration options include:
- CRM integration: By integrating Teams with CRM platforms like Salesforce or Dynamics 365, developers can enable click-to-call functionality, automatically log call details, and provide contextual information during calls.
- Contact center solutions: Integrating Teams with contact center solutions can streamline call handling, improve agent productivity, and enhance customer satisfaction.
- AI-powered analytics: Integration with AI-powered analytics tools can help organizations gain insights into call patterns, sentiment analysis, and more, enabling data-driven decision-making.
- Call recording storage: Integrating Teams with cloud storage providers like Azure Blob Storage or Amazon S3 can facilitate the storage and retrieval of recorded calls.
Building Custom Applications With Teams Toolkit
The Microsoft Teams Toolkit is a set of tools and resources designed to help developers build custom applications for Teams. Using the toolkit, developers can create custom calling experiences by embedding Teams calling features within their applications, including call recording. Some of the key components of the Teams Toolkit include:
- Yeoman Generator: A scaffolding tool that helps developers quickly create a new Teams application project with the necessary files and structure
- App Studio: A visual editor that allows developers to design and configure their Teams application, including manifest files and app package creation
- Teams SDK: A collection of JavaScript libraries and APIs that enable developers to interact with Teams' features and functionalities, including calling and recording
Real-World Example: KPMG Streamlines Audit Team Communication and Enhances Client Collaboration With Microsoft Teams Calling Solutions
KPMG, a global professional services firm, implemented Microsoft Teams calling solutions to enhance communication and collaboration among its employees across various departments and locations. One specific example of how KPMG utilized Microsoft Teams calling solutions is for their audit teams working with clients.
KPMG's audit teams often need to conduct meetings and calls with clients to discuss financial statements, review documentation, and address any concerns. To streamline this process and improve the overall experience, KPMG's IT team integrated Microsoft Teams with their existing systems using technical solutions.
Integration With CRM System
KPMG's IT team used the Microsoft Graph API to integrate Microsoft Teams with their CRM system. This allowed them to synchronize client information between the two systems and enabled click-to-call functionality directly from the CRM system. The integration also facilitated automatic logging of call details, timestamps, and participants, providing a comprehensive record of client interactions.
Secure Document Sharing
KPMG's developers built a custom application within Microsoft Teams to enable secure document sharing during calls. They leveraged Microsoft Teams' built-in security features, such as multi-factor authentication and end-to-end encryption, to ensure that sensitive financial documents were protected. The application also utilized Microsoft Teams' file-sharing capabilities, allowing audit teams to upload, share, and collaborate on documents in real time.
Optimizing Performance and Scalability
To ensure smooth performance and scalability, KPMG's IT team implemented load balancing and real-time monitoring solutions. They used Azure Load Balancer to distribute incoming client traffic across multiple Microsoft Teams instances, ensuring optimal performance even during periods of high demand. Additionally, they employed Azure Monitor to track key performance metrics, identify potential bottlenecks, and proactively address any issues.
By implementing Microsoft Teams calling solutions with these technical insights, KPMG's audit teams were able to improve productivity, enhance collaboration with clients, and streamline communication across the organization. The custom applications and integrations not only improved internal communication but also enhanced the overall client experience by providing faster and more efficient access to relevant information during calls.
Potential Drawbacks and Challenges for Developers Implementing Microsoft Teams
While Microsoft Teams offers numerous benefits and capabilities, developers may face some technical challenges when implementing smart calling solutions. Some potential drawbacks and challenges include:
- API limitations: The Microsoft Graph API, while powerful, may have some limitations or restrictions that developers need to be aware of, such as rate limiting and access control.
- Debugging and troubleshooting: Developers may encounter issues when integrating Microsoft Teams with third-party services or custom applications, requiring in-depth debugging and troubleshooting to resolve potential conflicts or compatibility problems.
- Customization limitations: While the Teams Toolkit provides a range of tools for building custom applications, there might be limitations in the extent of customization possible within the Teams platform, which could impact the desired functionality or user experience.
- Scalability concerns: As the number of users or integrations increases, developers must ensure that their custom applications and integrations can scale effectively without impacting performance or stability.
Conclusion
Smart calling solutions with Microsoft Teams empower developers to create seamless communication experiences that drive productivity and collaboration. By leveraging the Microsoft Graph API, integrating with third-party services, and building custom applications using the Teams Toolkit, developers can overcome potential challenges and enhance the user experience. While there may be some drawbacks and challenges in implementing Microsoft Teams, the benefits of improved communication, collaboration, and streamlined processes undoubtedly outweigh these obstacles. As organizations continue to adopt remote and hybrid work models, embracing smart calling solutions with Microsoft Teams will be crucial for success in the modern business environment.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK