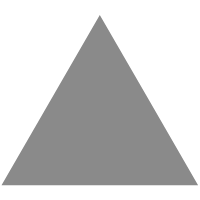
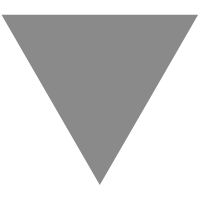
Install and Execute Python Applications Using pipx
source link: https://realpython.com/python-pipx/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Get Started With pipx
On the surface, pipx
resembles pip
because it also lets you install Python packages from PyPI or another package index. However, unlike pip
, it doesn’t install packages into your system-wide Python interpreter or even an activated virtual environment. Instead, it automatically creates and manages virtual environments for you to isolate the dependencies of every package that you install.
Additionally, pipx
adds symbolic links to your PATH
variable for every command-line script exposed by the installed packages. As a result, you can invoke those scripts directly from the command line without explicitly running them through the Python interpreter.
Think of pipx
as Python’s equivalent of npx
in the JavaScript ecosystem. Both tools let you install and execute third-party modules in the command line just as if they were standalone applications. However, not all modules are created equal.
Broadly speaking, you can classify the code distributed through PyPI into three categories:
- Importable: It’s either pure-Python source code or Python bindings of compiled shared objects that you want to import in your Python projects. Typically, they’re libraries like Requests or Polars, providing reusable pieces of code to help you solve a common problem. Alternatively, they might be frameworks like FastAPI or PyGame that you build your applications around.
- Runnable: These are usually command-line utility tools like
black
,isort
, orflake8
that assist you during the development phase. They could also be full-fledged applications likebpython
or the JupyterLab environment, which is primarily implemented in a foreign TypeScript programming language. - Hybrid: They combine both worlds by providing importable code and runnable scripts at the same time. Flask and Django are good examples, as they offer utility scripts while remaining web frameworks for the most part.
Making a distribution package runnable or hybrid involves defining one or more entry points in the corresponding configuration file. Historically, these would be setup.py
or setup.cfg
, but modern build systems in Python should generally rely on the pyproject.toml
file and define their entry points in the [project.scripts]
TOML table.
Note: If you use Poetry to manage your project’s dependencies, then you can add the appropriate script declarations in the tool-specific [tool.poetry.scripts]
table.
Each entry point represents an independent script that you can run by typing its name at the command prompt. For example, if you’ve ever used the django-admin
command, then you’ve called out an entry point to the Django framework.
Note: Don’t confuse entry points, which link to individual functions or callables in your code, with runnable Python packages that rely on the __main__
module to provide a command-line interface.
For example, Rich is a library of building blocks for creating text-based user interfaces in Python. At the same time, you can run this package with python -m rich
to display a demo application that illustrates various visual components at your fingertips. Despite this, pipx
won’t recognize it as runnable because the library doesn’t define any entry points.
To sum up, the pipx
tool will only let you install Python packages with at least one entry point. It’ll refuse to install runnable packages like Rich and bare-bones libraries that ship Python code meant just for importing.
Once you identify a Python package with entry points that you’d like to use, you should first create and activate a dedicated virtual environment as a best practice. By keeping the package isolated from the rest of your system, you’ll eliminate the risk of dependency conflicts across various projects that might require the same Python library in different versions. Furthermore, you won’t need the superuser permissions to install the package.
Deciding where and how to create a virtual environment and then remembering to activate it every time before running the corresponding script can become a burden. Fortunately, pipx
automates these steps and provides even more features that you’ll explore in this tutorial. But first, you need to get pipx
running itself.
Test Drive pipx
Without Installation
If you’re unsure whether pipx
will address your needs and would prefer not to commit to it until you’ve properly tested the tool, then there’s good news! Thanks to a self-contained executable available for download, you can give pipx
a spin without having to install it.
To get that executable, visit the project’s release page on the official GitHub repository in your web browser and grab the latest version of a file named pipx.pyz
. Files with the .pyz
extension represent runnable Python ZIP applications, which are essentially ZIP archives containing Python source code and some metadata, akin to JAR files in Java. They can optionally vendor third-party dependencies that you’d otherwise have to install by hand.
Note: Internally, the pipx
project uses shiv
to build its Python ZIP application. When you first run a Python ZIP application that was built with shiv
, it’ll unpack itself into a hidden folder named .shiv/
located in your user’s home directory. As a result, subsequent runs of the same application will reuse the already extracted files, speeding up the startup time.
Afterward, you can run pipx.pyz
by passing the path to your downloaded copy of the file to your Python interpreter—just as you would with a regular Python script:
PS> python .\pipx.pyz --version
1.4.3
In addition to this, on macOS and Linux, you can use chmod
to make the file executable (+x
) and run it directly without specifying the python
command:
$ chmod +x pipx.pyz
$ ./pipx.pyz --version
1.4.3
This is made possible because of a shebang line included at the beginning of the ZIP archive, indicating the interpreter to run the file through. Even though it’s technically a binary file, the shell recognizes the shebang line before handing over the file to your Python interpreter.
You can pass command-line arguments and options to pipx.pyz
just as you would with the installed version of pipx
. For example, to install IPython into its own isolated environment, you can use this command:
PS> python .\pipx.pyz install ipython
installed package ipython 8.22.1, installed using Python 3.12.2
These apps are now globally available
- ipython.exe
- ipython3.exe
These manual pages are now globally available
- man1\ipython.1
done! ✨ 🌟 ✨
Depending on which python
executable you choose to run the ZIP application, pipx
will base the virtual environment on that Python version.
A benefit of running pipx
as a self-contained Python ZIP application is that it won’t clutter your computer. On the other hand, it can get tedious after a while as you’ll need to specify its full path or locate the pipx.pyz
file and navigate to the parent directory each time. If you get tired of this nuisance, then it’s probably time to install pipx
on your system.
Install pipx
as a Standalone Tool
You’ve got several choices when it comes to installing pipx
on your computer, including an option to install pipx
through pipx
itself! This is not recommended, however, as it can lead to unexpected problems down the line.
Your next best option is to install pipx
using pip
. After all, the tool is available on PyPI as an ordinary Python package. That’s the most straightforward and reliable installation method, which will cause you the least amount of headaches. But, use it only when you don’t mind a few extra dependencies in your global Python interpreter, and never share it with other projects:
PS> python -m pip install pipx
This always brings the tool’s latest release that should work out of the box. However, because pipx
ends up being installed as a Python module, you’ll need to invoke it by using the full python -m pipx
command. To use the plain pipx
command instead, you can configure Unix shell completions, as described in the next section.
Anyhow, the officially recommended way to get pipx
installed is through your operating system’s package manager, such as Scoop on Windows, Homebrew on macOS, or APT on Debian-based Linux distributions:
PS> scoop install pipx
Unfortunately, there’s no corresponding pipx
package for Chocolatey, which you might’ve used to install Python if you followed Real Python’s Windows coding setup guide mentioned in the introduction.
When you install pipx
as a system package, it becomes a standalone command that you can run from anywhere in your file system. At the same time, it may not be the most up-to-date release of pipx
, and you may end up installing a whole lot of additional dependencies, such as yet another Python interpreter.
It’s important to understand that installing pipx
as a system package ties it to the specific Python interpreter listed as a system package dependency, which may be obsolete. So, when you later install an application with pipx
, the tool will stick to whatever Python interpreter it came with to create a new virtual environment. But don’t worry. You’ll learn how to override this default choice when needed.
Configure pipx
Before the First Run
Regardless of whether you’ve installed pipx
or not, the first thing you must always do before you can use the tool to the fullest is to add the necessary folder paths to your PATH
environment variable. If you forget this step, then pipx
will remind you about it and provide a helpful message on the first occasion:
PS> pipx install ipython
installed package ipython 8.22.1, installed using Python 3.12.2
These apps are now globally available
- ipython.exe
- ipython3.exe
These manual pages are now globally available
- man1\ipython.1
⚠️ Note: 'C:\Users\User\.local\bin' is not on your PATH environment variable.
⮑ These apps will not be globally accessible until your PATH is updated.
⮑ Run `pipx ensurepath` to automatically add it, or manually modify your
⮑ PATH in your shell's config file (i.e. ~/.bashrc).
done! ✨ 🌟 ✨
After successfully installing IPython, pipx
created two symbolic links to the ipython
and ipython3
executables in the associated virtual environment. Unfortunately, there’s currently no way to access those symlinks from your terminal because their parent folder isn’t listed on the PATH
variable.
To fix this, you can run the command suggested in the output message above and then reopen the terminal or reload your shell configuration:
PS> pipx ensurepath
Success! Added C:\Users\User\.local\bin to the PATH environment variable.
Consider adding shell completions for pipx.
⮑ Run 'pipx completions' for instructions.
You will need to open a new terminal or re-login for the PATH changes
⮑ to take effect.
Otherwise pipx is ready to go! ✨ 🌟 ✨
On Windows, running pipx ensurepath
modifies your user’s %PATH%
environment variable. It appends a path to the home directory where pipx
stores the installed packages, virtual environments, and more.
If you’re on macOS or Linux, then running the same command will add the following entry at the bottom of your shell’s configuration file, such as .bashrc
or .zshrc
, located in your user’s home directory:
~/.bashrc
# ...
# Created by `pipx` on 2024-02-22 13:08:43
export PATH="$PATH:/home/user/.local/bin"
It has virtually the same effect as modifying the %PATH%
variable on Windows. The next time you try running pipx ensurepath
, it’ll detect that you’ve already run it and won’t add redundant entries to your shell configuration.
To reveal the directory paths used by pipx
on your computer, you can invoke the environment
subcommand:
PS> pipx environment
Environment variables (set by user):
PIPX_HOME=
PIPX_BIN_DIR=
PIPX_MAN_DIR=
PIPX_SHARED_LIBS=
PIPX_DEFAULT_PYTHON=
USE_EMOJI=
Derived values (computed by pipx):
PIPX_HOME=C:\Users\User\AppData\Local\pipx\pipx
PIPX_BIN_DIR=C:\Users\User\.local\bin
PIPX_MAN_DIR=C:\Users\User\.local\share\man
PIPX_SHARED_LIBS=C:\Users\User\AppData\Local\pipx\pipx\shared
PIPX_LOCAL_VENVS=C:\Users\User\AppData\Local\pipx\pipx\venvs
PIPX_LOG_DIR=C:\Users\User\AppData\Local\pipx\pipx\Logs
PIPX_TRASH_DIR=C:\Users\User\AppData\Local\pipx\pipx\trash
PIPX_VENV_CACHEDIR=C:\Users\User\AppData\Local\pipx\pipx\Cache
PIPX_DEFAULT_PYTHON=C:\Users\User\.pyenv\pyenv-win\versions\3.12.2\python.exe
USE_EMOJI=true
It displays the folder paths specific to your platform, which you can override by specifying one or more environment variables listed at the top of the output. You can also override the path to your default Python interpreter and disable the use of emoji this way.
One last step, which is completely optional and only applicable to macOS and Linux, was hinted at by your first run of the ensurepath
subcommand. It encouraged you to consider adding shell completions for pipx
to your terminal. Run pipx completions
to get the relevant instructions for the most popular shell flavors:
$ pipx completions
Add the appropriate command to your shell's config file
so that it is run on startup. You will likely have to restart
or re-login for the autocompletion to start working.
bash:
eval "$(register-python-argcomplete pipx)"
(...)
For example, to enable the pipx
completions in Bash, you can add the highlighted line to your ~/.bashrc
configuration file and reload it:
$ echo 'eval "$(register-python-argcomplete pipx)"' >> ~/.bashrc
$ source ~/.bashrc
This will enable command auto-completion for pipx
in Bash. Additionally, if you installed pipx
as a Python package using pip
, then it’ll also create a symlink to the pipx
executable so that you can invoke it directly rather than as python -m pipx
.
Now that you have everything in place, you can start using pipx
to install and run Python applications in isolated environments.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK