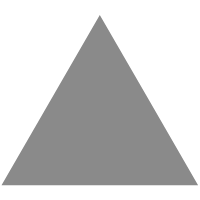
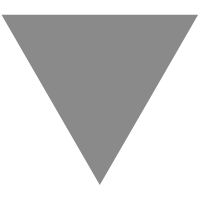
GitHub - garronej/screen-scaler: 🖥️ One-size-fit-all web design: Screen-size agn...
source link: https://github.com/garronej/screen-scaler
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Repository files navigation
One-size-fit-all web design: Screen-size agnostic development environment.
Motivation and Warning
Screen-Scaler is a niche tool designed for very specific use cases. It enables you to design your application as though every user has the same screen size, automating the adjustment process for different screen dimensions by scaling your app to fit. While this can significantly streamline development for certain projects, it is not a replacement for responsive design and is not recommended for general-purpose applications.
Responsive design remains the gold standard for creating web applications that offer the best user experience across a wide range of devices. Screen-Scaler should be considered either a temporary hack or a solution for projects where user screens are highly uniform and predictable.
Features
- 📏 Automatically scales your app to fit any screen size that differs from your target resolution.
- 🎭 Fully spoofs DOM APIs to emulate your specified settings.
- 🔌 Requires no changes to your existing code base; it's a simple function call and integrates seamlessly with any CSS Framework and UI library.
- 🛠️ Offers flexibility by enabling scaling only for specific screen size ranges. For instance, if your app renders well on large screens but breaks on smaller ones, you can activate scaling only for screen widths below
1000px
. - ♿ Preserves accessibility features, allowing users to zoom in and out with
ctrl + mouse wheel
or⌘ + '+/-'
, provided you enable this functionality (and you should).
Usage
Make it so that your app is always rendered as if the user had a screen resolution width of 1920.
import { enableScreenScaler } from "screen-scaler";
enableScreenScaler({
// The zoom factor is for supporting when the user zooms in or out (ctrl + mouse wheel or ⌘ + '+' or ⌘ + '-') ...
targetWindowInnerWidth: ({ zoomFactor }) => 1920 * zoomFactor,
// If you don't want to enables your user to zoom you can provide an absolute value
//targetWindowInnerWidth: 1920
// This is the id of the root div of your app. With modern frameworks it's usually "root" or "app".
rootDivId: "app"
});
Run the test App
git clone https://github.com/garronej/screen-scaler
cd screen-scaler
yarn
yarn start-test-app
Screen scaler is setup in the src/index.tsx
file.
Limitations and workarounds
Server side rendering
SSR isn't supported, if in Next.js you will have to wrap your all app in a no-ssr
component.
vh
and vw
CSS properties
The use of vh
and vw
CSS properties is not supported, they can't be spoofed.
If you where using 100vh
to make your app take the full height of the screen, you can use the following workaround:
root.render(
<React.StrictMode>
<div style={{
overflow: "hidden",
- height: "100vh"
+ height: "100%", // Or height: window.innerHeight
}}>
</div>
</React.StrictMode>
);
Portrait mode
When your app is rendered in a device in portrait mode, if you haven't accommodated for this your app will appear very tiny at the top of the screen and most of the screen will be unused.
In this case, you have two options:
1: Implement a portrait mode version of your app. 2: Tell your user to rotate their device:
import { enableScreenScaler } from "screen-scaler/react";
const { ScreenScalerOutOfRangeFallbackProvider } = enableScreenScaler({
rootDivId: "root",
targetWindowInnerWidth: ({ zoomFactor, isPortraitOrientation }) =>
isPortraitOrientation ? undefined : 1920 * zoomFactor
});
export function App() {
return (
<ScreenScalerOutOfRangeFallbackProvider
fallback={<h1>Please Rotate your phone, this app does not render well in portrait mode.</h1>}
>
{/* Your app here */}
</ScreenScalerOutOfRangeFallbackProvider>
);
}
NOTE: We provide this example using the dedicated React adapter. To do that in another framework you will need to replace your app by a fallback element when your
targetWindowInnerWidth
returnsundefined
. If you'd like an adapter for your framework of choice, please open an issue.
Readability issues
The issue with scaling down your app is that the text becomes increasingly smaller as the screen size decreases. Conversely, on very large screens, everything appears disproportionately large, creating the impression that the app is designed for children. These issues can be mitigated by dynamically adjusting the target width.
enableScreenScaler({
// Example: Disabling the scaling for screen width above 1100px
targetWindowInnerWidth: ({ actualWindowInnerWidth }) => Math.max(windowInnerWidth, 1100)
});
Showcases
This library has been used to build the following projects:
Contributing
git clone https://github.com/garronej/screen-scaler
cd screen-scaler
yarn
# Start the test app in watch mode
yarn start-test-app
# Link in an external project in watch mode
yarn link-in-app YOUR-APP # ../YOUR-APP is supposed to exist
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK