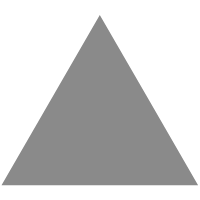
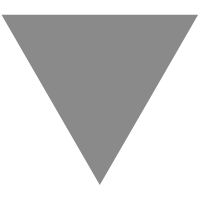
Top 5 Tips for Optimizing React Performance
source link: https://blog.bitsrc.io/tips-for-optimizing-react-performance-890f2b3a36d7
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
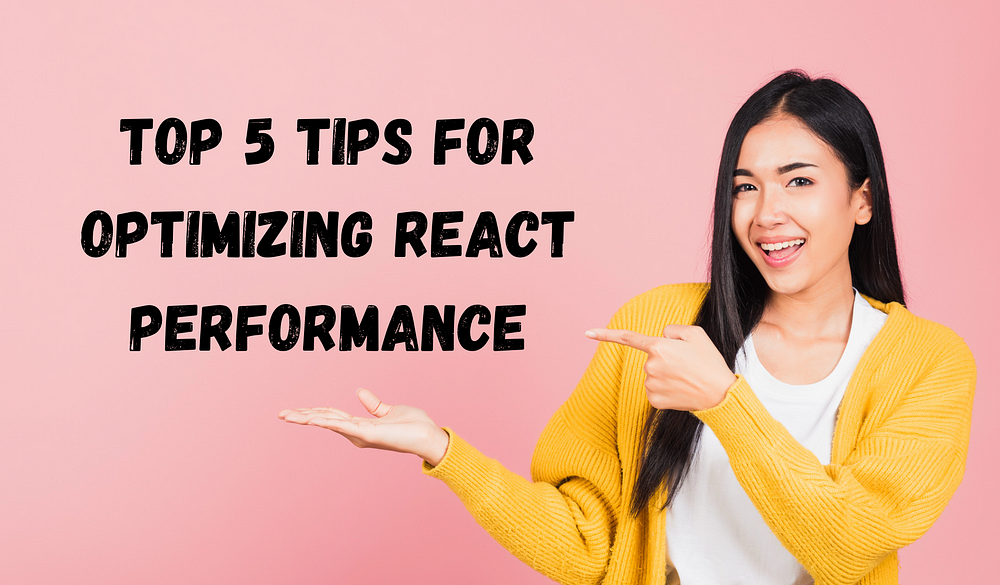
React.js lets you build dynamic and interactive web applications. However, achieving top-notch performance can be a challenge, especially when dealing with large data sets or complex components.
So, let’s take a look at how you can optimize your React applications for maximum performance!
1. Reduce unnecessary renders
When building React applications, it’s essential to optimize performance. One critical aspect is minimizing the number of component re-renders. Unnecessary re-renders can lead to sluggish user experiences and impact overall responsiveness.
import React, { memo } from 'react';
const ComponentB = memo((props) => {
return <div>{props.propB}</div>;
});
By wrapping ComponentB
with memo()
, it will only re-render when propB
actually changes value, regardless of how many times its parent re-renders.
useCallback() is a hook that memoizes callback functions. It’s particularly useful when passing callbacks as props to child components.
import React, { useCallback } from 'react';
const ParentComponent = () => {
const handleButtonClick = useCallback(() => {
// Handle button click logic
}, []);
return <ChildComponent onClick={handleButtonClick} />;
};
By using useCallback()
, you ensure that the callback function remains the same across renders unless its dependencies change.
While optimizing performance with techniques like React.memo()
and useCallback()
, it’s essential to strike a balance. Memoization, which compares old and new props, can be resource-intensive for large props or when passing React components as props.
2. Lazy loading with code splitting
Lazy loading and code splitting are techniques used to optimize the performance of a React application by loading only the necessary components and splitting the code into smaller bundles, reducing the initial load time.
Lazy loading can be implemented using the Suspense
component and the lazy
function from the react
module. The lazy
function allows you to define a component that will be loaded lazily when it is needed.
Here’s an example:
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function MyComponent() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
In this example, LazyComponent
will only be loaded when it is needed and displayed within the Suspense
component. The fallback
prop is used to specify the content to be displayed while the component is being loaded.
Code splitting can be achieved using dynamic imports, which allow you to split your code into smaller bundles that can be loaded on demand. Here’s an example:
import React, { lazy, Suspense } from 'react';
const LazyComponent = lazy(() => import('./LazyComponent'));
function MyComponent() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<button onClick={() => import('./AnotherLazyComponent')
.then((module) => {
const AnotherLazyComponent = module.AnotherLazyComponent;
setComponent(<AnotherLazyComponent />);
})}>
Load Another Lazy Component
</button>
<LazyComponent />
</Suspense>
</div>
);
}
In this example, AnotherLazyComponent is loaded dynamically when the button is clicked, allowing you to split your code into smaller bundles and reduce the initial load time.
By using lazy loading and code splitting, you can ensure that your application only loads the necessary components and code, reducing the initial load time and improving the overall performance.
3. Debouncing and Throttling
Debouncing and throttling are techniques used to control the rate at which functions are called. Debouncing ensures that a function is called only once during a given period, while throttling ensures that a function is called at most once during a given period. These techniques can be useful for improving performance in React applications, especially when dealing with user input that can trigger expensive computations or network requests.
Let’s start with debouncing. Imagine you have a search bar in your React application that sends a network request to a server every time the user types a character. This can result in a lot of unnecessary requests and slow down the application. To avoid this, you can use debouncing to ensure that the network request is only sent after the user has stopped typing for a certain amount of time.
Here’s an example of how to use lodash’s debounce
function to implement debouncing in a React component:
import React, { useState } from 'react';
import debounce from 'lodash.debounce';
function SearchBar() {
const [query, setQuery] = useState('');
// Debounce the search function to ensure it's only called once
// after the user has stopped typing for 500ms
const debouncedSearch = debounce(query => {
// Send network request to search for the query
console.log(Searching for: ${query} );
}, 500);
const handleQueryChange = event => {
const newQuery = event.target.value;
setQuery(newQuery);
debouncedSearch(newQuery);
};
return (
<input type="text" value={query} onChange={handleQueryChange} />
);
}
In this example, the handleQueryChange
function is called every time the user types a character in the search bar. However, instead of calling the debouncedSearch
function directly, we pass it to the debounce
function to ensure it's only called once after the user has stopped typing for 500ms.
Now let’s talk about throttling. Throttling can be useful when you want to limit the rate at which a function is called, even if it’s called multiple times in quick succession. For example, you might want to limit scroll events to 100ms to avoid overwhelming the browser’s event loop.
Here’s an example of how to use lodash’s throttle
function to implement throttling in a React component:
import React from 'react';
import throttle from 'lodash.throttle';
function ScrollComponent() {
const [scrollTop, setScrollTop] = useState(0);
// Throttle the scroll function to ensure it's only called once
// every 100ms, even if the user scrolls multiple times during that period
const throttledScroll = throttle(() => {
// Update the scrollTop state with the current scroll position
setScrollTop(window.pageYOffset);
}, 100);
const handleScroll = () => {
throttledScroll();
};
return (
<div onScroll={handleScroll} style={{ height: '500vh' }}>
<p>Scroll down to see the scroll position:</p>
<p>Scroll position: {scrollTop}</p>
</div>
);
}
In this example, the handleScroll
function is called every time the user scrolls the page. However, instead of updating the scrollTop
state directly, we pass it to the throttle
function to ensure it's only called once every 100ms, even if the user scrolls multiple times during that period.
By using debouncing and throttling in your React applications, you can create more responsive and efficient user interfaces that provide a better experience for your users.
4. Virtualize long lists
Virtualization is a technique for improving the performance of applications that display long lists of items. The idea behind virtualization is only to render the items that are currently visible on the screen rather than rendering all of the items in the list. This can significantly reduce the amount of memory and CPU usage required to display the list, resulting in faster load times and a smoother user experience.
Here is an example of how you might use react-virtualized to virtualize a long list:
import React from 'react';
import { List } from 'react-virtualized';
// Define the height of each row in the list
const rowHeight = 30;
// Define the total number of items in the list
const totalItems = 1000;
// Define the size of the visible area (i.e., the viewport)
const rowCount = 10;
const width = 300;
const height = rowCount * rowHeight;
// Define the data for the list
const listData = Array.from({ length: totalItems }, (_, i) => Item ${i} );
// Define the component to render for each row in the list
const Row = ({ index, style }) => (
<div style={style}>{listData[index]}</div>
);
// Render the virtualized list
const VirtualizedList = () => (
<List
width={width}
height={height}
rowCount={totalItems}
rowHeight={rowHeight}
rowRenderer={Row}
/>
);
// Export the VirtualizedList component for use in your app
export default VirtualizedList;
In this example, we define the height of each row in the list (rowHeight
), the total number of items in the list (totalItems
), and the size of the visible area (rowCount
, width
, and height
). We also define the data for the list (listData
) and the component to render for each row (Row
).
Finally, we render the virtualized list using the List
component from react-virtualized
. The List
component takes several props, including the width and height of the list, the number of rows and the height of each row, and a rowRenderer
function that defines how to render each row.
By using react-virtualized
to virtualize our long list, we can significantly improve the performance of our React application, especially for lists with a large number of items.
5. Optimizing your images
Optimizing images is crucial for improving the performance of your React application, as images can significantly impact page load times.
Here’s an example of how to use the react-optimized-image package to optimize images in a React application.
import React from 'react';
import OptimizedImage from 'react-optimized-image';
const MyComponent = () => (
<OptimizedImage
src="https://example.com/image.jpg"
alt="Optimized image"
width={300}
height={200}
loading="lazy" // optional: set to "eager" to load the image immediately
/>
);
export default MyComponent;
In this example, we’re using the OptimizedImage
component to load an image from a remote URL. We're also specifying the width
and height
of the image, as well as setting the loading
prop to"lazy"
to defer loading of the image until it's near the viewport.
The react-optimized-image
package automatically optimizes the image using the imgix
service, which provides image compression, resizing, and other optimizations. The package also includes support for lazy loading and progressive loading, which can further improve load times and user experience.
Conclusion
Performance optimization is crucial in modern web development, especially with the increasing complexity of web applications.
By focusing on reducing unnecessary renders, employing lazy loading with code splitting, using debouncing and throttling, virtualizing long lists, and optimizing images, developers can significantly enhance the performance of their React applications.
Try out these measures in your React apps to ensure that they’re performing as well as they can!
Thank you for reading.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK