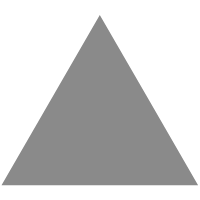
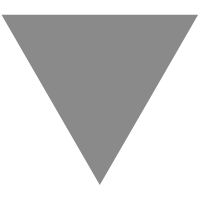
JSP - Form Processing - GeeksforGeeks
source link: https://www.geeksforgeeks.org/jsp-form-processing/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
In JavaServer Pages, Form processing is the fundamental aspect of web development, and it can allow users to interact with websites by submitting data. It can handle form submissions involving extracting the data from the HTTP requests and generating the appropriate responses.
Form Processing in JSP
Form processing in the JavaServer Pages involves receiving data submitted through the HTML forms extracting this data from the request object and generating the response based on the received data.
Key Terminologies:
- JavaServer Pages (JSP): This is a technology that can help software developers create dynamic web pages in HTML, XML, and other document types. It can allow Java code to be inserted into HTML pages and that code executed on the server before the pages are served on client’s websites.
- Form processing: This refers to the handling of data transmitted via HTML forms on web pages. It can insert customer form data into HTML requests, and process this data, generating responses.
- HTTP Methods:
- GET: HTTP GET requests are used to request data from the specified object and when a form is submitted using the GET method and the form data is loaded into the query parameters of the URL.
- POST: This can be an HTTP POST request if the data to be processed is sent to the specified object and when the form is submitted using the POST method and the form data is sent to the body of the HTTP request.
- Request Object: A request object in a JSP can represent an HTTP request made by a client and provide methods for retrieving information sent by the client such as form parameters, headers, and cookies
- Response Object: The response object in the JSP can represent an HTTP response to be sent back to the client, and provide a response header, and methods for setting cookies and delivering content to the client browser.
- Parameter: It is the values sent to the server as part of an HTTP request and in form processing the parameters typically can represent the form field values submitted by the users.
- Form Validation: It is the process of ensuring that the data submitted through the form meets certain criteria or constraints and it can help prevent errors and ensure the integrity and security of the data being processed.
- Servlets: It is a Java program that can extend the capabilities of the server and servlets can often be used to handle more complex form-processing tasks such as data validation database operations and business logic.
- Session: It is a tool for checking the context between HTTP requests from a single client and can be used to store user-specific data between multiple requests such as user authentication tokens or shopping cart content
In JavaServer Pages (JSP), form processing involves handling data submitted through HTML forms. This can be done using various HTTP methods like GET and POST. Here are examples of processing forms using both GET and POST methods.
JSP Form Processing Using GET Method
The GET method is one of the HTTP methods used to send form data to a web server. When this happens, the form is submitted using the GET method and the form data is inserted into the query parameters of the URL.
Step-by-Step implementation of GET Method
Step 1: Create the dynamic web project using eclipse and its project named as jsp-GET-demo.
Note: If you beginner click here to know the process of creating the dynamic web project.
Step 2: After creating a JSP form named index.jsp and this form includes input fields to store user data and the form attributes can be set on the JSP page to customize the form to be submitted and set the method attribute enter “GET”.
HTML
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>GET Form Processing</title>
<style>
/* CSS styles for better visibility */
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
.container {
width: 50%;
margin: 50px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h2 {
color: #333;
text-align: center;
}
form {
margin-top: 20px;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
}
input[type="text"],
input[type="email"],
input[type="submit"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box; /* Ensure padding and border are included in width */
}
input[type="submit"] {
background-color: #007bff;
color: #fff;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<div class="container">
<h2>Submit Form (GET Method)</h2>
<form action="processGet.jsp" method="GET">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br>
<input type="submit" value="Submit">
</form>
</div>
</body>
</html>
Step 3: Create a new JSP page and name it processGet.jsp which processes the submission process and retrieves the form data from the request object using the getParameter() method on this page. This method can name the form field as its parameter and return the value entered by the user.
HTML
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>GET Form Processing</title>
<link rel="stylesheet" type="text/css" href="WEB-INF/css/style.css">
<style>
/* Additional CSS for better visibility */
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
.container {
width: 50%;
margin: 50px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h2, h3 {
color: #333;
text-align: center;
}
p {
text-align: center;
}
</style>
</head>
<body>
<div class="container">
<h2>GET Form Processing</h2>
<%-- Retrieving parameters from the request --%>
<% String name = request.getParameter("name"); %>
<% String email = request.getParameter("email"); %>
<h3>Hello, <%= name %></h3>
<p>Your email is: <%= email %></p>
</div>
</body>
</html>
Step 4: Once we complete the project, it runs the tomcat server then output shows the form. Refer the below output image for better understanding.
Output:
1. Display the Form
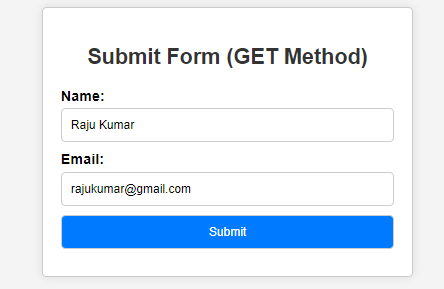
2. Display the processed Data:
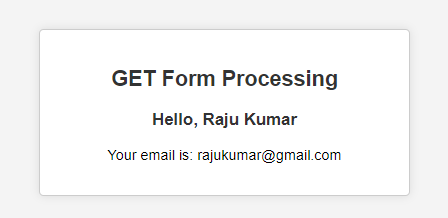
JSP Form Processing Using Post Method
Below is the step-by-step implementation of POST method.
Step-by-Step implementation of POST Method
Step 1: Create the dynamic web project using eclipse and its project named as jsp-POST-demo.
Step 2: Create a JSP form named index.jsp and this form includes input fields to store user data and form attributes that can be stored on a JSP page to configure the form to be submitted and set the method attribute to “POST “.
HTML
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Form Processing</title>
<link rel="stylesheet" type="text/css" href="WEB-INF/css/style.css">
<style>
/* Additional CSS for better visibility */
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
.container {
width: 50%;
margin: 50px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h2 {
color: #333;
text-align: center;
}
form {
margin-top: 20px;
}
label {
display: block;
margin-bottom: 5px;
font-weight: bold;
}
input[type="text"],
input[type="email"],
input[type="checkbox"],
input[type="radio"],
input[type="submit"] {
width: 100%;
padding: 10px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
box-sizing: border-box; /* Ensure padding and border are included in width */
}
input[type="submit"] {
background-color: #007bff;
color: #fff;
cursor: pointer;
}
input[type="submit"]:hover {
background-color: #0056b3;
}
</style>
</head>
<body>
<div class="container">
<h2>Submit Form (POST Method)</h2>
<form action="processPost.jsp" method="POST">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br>
<label for="subscribe">Subscribe:</label>
<input type="checkbox" id="subscribe" name="subscribe"><br>
<label for="gender">Gender:</label>
<input type="radio" id="male" name="gender" value="male"> Male
<input type="radio" id="female" name="gender" value="female"> Female<br>
<input type="submit" value="Submit">
</form>
</div>
</body>
</html>
Step 3: Create the new JSP page and it named as processPost.jsp to handles the form submission and in this page can retrieve the form data from the request object using getParameter() method. This method can take the name of the form field as its the parameter and returns the value entered by user.
HTML
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>POST Form Processing</title>
<link rel="stylesheet" type="text/css" href="WEB-INF/css/style.css">
<style>
/* Additional CSS for better visibility */
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
.container {
width: 50%;
margin: 50px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
background-color: #fff;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
h2, h3 {
color: #333;
text-align: center;
}
p {
text-align: center;
}
</style>
</head>
<body>
<div class="container">
<h2>POST Form Processing</h2>
<%-- Retrieving parameters from the request --%>
<% String name = request.getParameter("name"); %>
<% String email = request.getParameter("email"); %>
<% String subscribe = request.getParameter("subscribe"); %>
<% String gender = request.getParameter("gender"); %>
<h3>Hello, <%= name %></h3>
<p>Your email is: <%= email %></p>
<p>Subscribed: <%= (subscribe != null) ? "Yes" : "No" %></p>
<p>Gender: <%= gender %></p>
</div>
</body>
</html>
Step 4: Once we complete the project, it runs the tomcat server then output shows the form. Refer the below output image for better understanding.
Output:
1. Display the FORM:
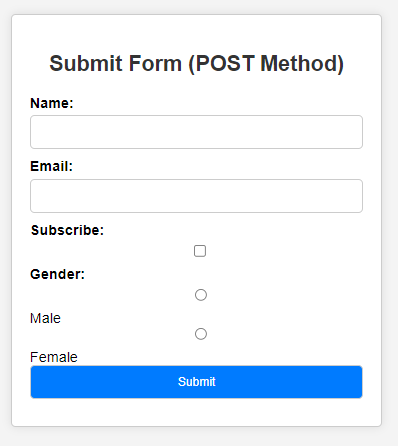
2. Display Processed Data:
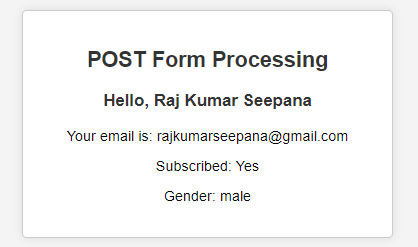
If we follow the above steps, then we can successfully build the JSP form processing demo project.
Feeling lost in the vast world of Backend Development? It's time for a change! Join our Java Backend Development - Live Course and embark on an exciting journey to master backend development efficiently and on schedule.
What We Offer:
- Comprehensive Course
- Expert Guidance for Efficient Learning
- Hands-on Experience with Real-world Projects
- Proven Track Record with 100,000+ Successful Geeks
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK