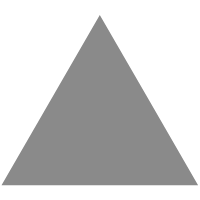
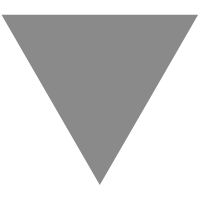
纯函数:每个开发人员都应该了解的知识
source link: https://www.techug.com/post/pure-functions-every-developer-should-know-about/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
在编程的海洋中冲浪时,我们通常会遇到许多冰山(即概念),这些冰山从外表上看非常渺小和基础,但实际上却非常巨大和深奥,纯函数就是其中之一。
定义简单地说,纯函数是函数式编程中的一个概念,它遵循以下两套规则:
- 确定性:“给定相同的输入参数集,纯函数将始终产生相同的输出”,读到这里,你可能会想起在哪里学过这句话,如果是的话,那么你就对了,这个概念是在数学中使用的。f(x,y) = x + y 是数学纯函数的一个例子,这个函数接受两个输入,即 x 和 y,对于同一对输入,总是产生相同的总和。
- 无副作用:纯函数没有副作用,这意味着它们不会修改其作用域之外的变量、改变全局状态、执行 I/O 操作或以计算结果之外的任何方式与外部世界交互。函数的唯一目的就是根据输入计算并返回值。
纯函数是什么样的 🤔 ?
现在,让我们通过一个简单的例子来理解这一点:在这个例子中,add 函数是纯函数,因为它接收两个参数(a 和 b)并返回它们的和。它不会修改任何外部状态,使用相同的参数调用它将始终产生相同的结果。
// Pure function function add(a, b) { return a + b; } console.log( add(1,1) ) // Output: 2
与之相比,不纯函数会在其作用域之外修改变量:
// Variable outside functions scope let total = 0; // Impure function function addToTotal(value) { total += value; return total; }
函数 addToTotal
是不纯的,因为它在其作用域之外修改了总变量,从而引入了副作用(另一个有趣的术语,你可以研究一下)。要预测函数在不同时间和不同环境下的行为变得更加困难。让我们举例说明,对于那些难以想象这为什么是个问题的人来说。
let itemPrice = 100; // Returns price after applying offer. function applyOffer(offerPercent){ // Updates variable outisde it's scope itemPrice = (itemPrice*(100-offerPercent))/100; return itemPrice; } console.log(applyOffer(10)); // Returns 90 for the first time console.log(applyOffer(10)); // Returns 81 for the second time 😕 console.log(itemPrice) // Returns 81
在这个例子中,你可以清楚地看到为什么不纯函数可能会导致问题。
额外信息:您知道 React 是围绕这一概念设计的吗?React 假定您编写的每个组件都是纯函数。这意味着在输入相同的情况下,您编写的 React 组件必须始终返回相同的 JSX:
让我们不纯的函数变纯:
为了使您刚才看到的 applyOffer
函数变得纯粹,您应该避免修改外部状态,而是确保它只依赖于其输入参数。
let itemPrice = 100; // Added a new arg, itemPrice. NOTE: you can name it whatever you want, I have just displayed the example of variable shadowing. function applyOffer(itemPrice,offerPercent){ // Updates variable outisde it's scope itemPrice = (itemPrice*(100-offerPercent))/100; return itemPrice; } console.log(applyOffer(itemPrice,10)); // Returns 90 for the first time console.log(applyOffer(itemPrice,10)); // Returns 90 for the second time 😄 console.log(itemPrice) // Returns 100
使用纯函数的好处:
- 易于信任,因为它们总是在相同的输入下给出相同的输出。
- 纯函数更容易组合,因为它们的 “输出只取决于输入”。
- 纯函数更易于测试和调试。
- 纯函数是可记忆的(因为它具有确定性)。
- 更易于并行化(阅读本文了解更多信息)
上述每项优势都可以写成一篇详细的文章,但现在,我只想让你思考一下,为什么这些显而易见的要点具有如此大的价值和用途。
我相信现在你已经很好地理解了什么是纯函数,以及为什么这个概念如此强大。关于 React 如何使用纯函数概念,您可以在 React 文档中找到相关示例。
理解这个概念很容易,但掌握它却很难。
本文文字及图片出自 Pure Functions: Every Developer should know about !!!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK